Hello,
Logic i am looking for :
If floating Balance is less than 1% in negative of account balance => Close the trade (OR) if floating balance is less than $100 in negative => Close the trade
Code :
Error : Balance, Equity and Floating balance do not change in Real time. Instead it takes the initial value when installing the EA and the values remains same.
When you write code in
start()
It happend
Run your code in
void OnTimer(void); |
It can help you
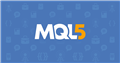
- www.mql5.com
Like this?
extern int negative_equity_percentage = 1; extern int negative_equity_balance = 50; double equity; double Account_balance; double floatingBalance;Void OnTimer() { equity = AccountEquity(); Account_balance = AccountBalance(); floatingBalance = equity - Account_balance; }
void CheckAndCloseTrade() { double NewspercentageThreshold = 0.01 * negative_equity_percentage * Account_balance; if ((floatingBalance < NewspercentageThreshold) || (floatingBalance < -negative_equity_balance)) { Print("Time to close the trades","/n"); } } int start() { CheckAndCloseTrade(); return(0); }
OnTimer would work, however it would run every 50ms and maybe 20 times every second. I recommend that you replace the start() with either Onstart() to turn it into a script; OR OnTick() to turn the code into an EA; and put the code that you have in OnTimer(), move that code into the OnStart() or OnTick(), where it will work immeditately if you want a script, OR 1 time every new tick, for an EA.
Having a system that requires closure of positions from condition 1. Account balance plus/minus 1% is practically the same to condition 2. Account equity above/below 1% from account balance. Number 2 will always be the first one to go through, so having the "||" syntax is redundant and having condition 1. has no use case. Furthermore, a fixed 1% will increase opportunity costs and may decrease volatility of returns from your system. Despite lowering the volatility of returns, overtime you may experience drawdown periods in which the real return is net negative because of those opportunity costs. I highly recommend you to evaluate risk parameters for better performance.
OnTimer would work, however it would run every 50ms and maybe 20 times every second. I recommend that you replace the start() with either Onstart() to turn it into a script; OR OnTick() to turn the code into an EA; and put the code that you have in OnTimer(), move that code into the OnStart() or OnTick(), where it will work immeditately if you want a script, OR 1 time every new tick, for an EA.
This is what you mean?
extern int negative_equity_percentage = 1; extern int negative_equity_balance = 50; double equity; double Account_balance; double floatingBalance;void OnTick() { equity = AccountEquity(); Account_balance = AccountBalance(); floatingBalance = equity - Account_balance; }
void CheckAndCloseTrade() { double NewspercentageThreshold = 0.01 * negative_equity_percentage * Account_balance; if ((floatingBalance < NewspercentageThreshold) || (floatingBalance < -negative_equity_balance)) { Print("Time to close the trades","/n"); } } int start() { CheckAndCloseTrade(); return(0); }
Do, i have to return anything in OnTick()?, Like return(0); ?
OnStart() and Start() - Behave differently?
Having a system that requires closure of positions from condition 1. Account balance plus/minus 1% is practically the same to condition 2. Account equity above/below 1% from account balance. Number 2 will always be the first one to go through, so having the "||" syntax is redundant and having condition 1. has no use case. Furthermore, a fixed 1% will increase opportunity costs and may decrease volatility of returns from your system. Despite lowering the volatility of returns, overtime you may experience drawdown periods in which the real return is net negative because of those opportunity costs. I highly recommend you to evaluate risk parameters for better performance.
Let say, if my account balance is $20K means 1% of 20K is 200 USD. in this case the second condition negative_equity_balance = 50 get false and execute the code by first condition negative_equity_percentage = 1.
Let say, if my account balance is $20K means 1% of 20K is 200 USD. in this case the second condition negative_equity_balance = 50 get false and execute the code by first condition negative_equity_percentage = 1.
This explanation has me fairly confused, you've stated:
If floating Balance is less than 1% in negative of account balance => Close the trade (OR) if floating balance is less than $100 in negative => Close the trade
This means you want to close positions when the balance is below the initial 1% of the account or $100 floating of the account. Now if in this particular example if the account size is $20,000, condition 2 again will always come through because 0.005 ($100) is less than 0.01
You need to give a detailed example of what you are talking about I am finding it hard to understand. Furthermore negative_equity_percentage = 1 is a multiplier and not a percentage,
double NewspercentageThreshold = 0.01 * negative_equity_percentage * Account_balance;
if ((floatingBalance < NewspercentageThreshold) || (floatingBalance < -negative_equity_balance))
The calculus and logic are both incorrect for what you are looking for. Using the $20,000 example and without any trades taken, you are basically saying if $200 is above equity then move forward or if $100 is above equity ($20,000) then move forward. Both conditions in this instance will never be true.
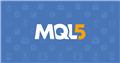
- www.mql5.com
This explanation has me fairly confused, you've stated:
If floating Balance is less than 1% in negative of account balance => Close the trade (OR) if floating balance is less than $100 in negative => Close the trade
This means you want to close positions when the balance is below the initial 1% of the account or $100 floating of the account. Now if in this particular example if the account size is $20,000, condition 2 again will always come through because 0.005 ($100) is less than 0.01
You need to give a detailed example of what you are talking about I am finding it hard to understand. Furthermore negative_equity_percentage = 1 is a multiplier and not a percentage,
The calculus and logic are both incorrect for what you are looking for. Using the $20,000 example and without any trades taken, you are basically saying if $200 is above equity then move forward or if $100 is above equity ($20,000) then move forward. Both conditions in this instance will never be true.
It's my mistake; you may be confused because I didn't share why I needed this. My EA has a news filter feature that pauses the EA from taking new trades, but old trades remain active until they hit TP or SL, and I did this with a purpose.
Now, I want to implement a condition: If 'news_closing' is true and 'News_filter' is true, then execute this condition: If the floating balance is less than 1% in the negative of the account balance, close the trade. Alternatively, if the floating balance is less than $100 in the negative, close the trade.
So, the full logic will look like
If (News_closing && News_filter) { double NewspercentageThreshold = 0.01 * negative_equity_percentage * Account_balance; //Calculating Percentage if ((floatingBalance < NewspercentageThreshold) || (floatingBalance < -negative_equity_balance)) { panicclosing(); //Panic closing is another function which close the all the trade if condition satisfied } }
Why do I need this? For example, if the news filter has paused new trades and an existing trade is running with a floating loss of -$800, I don't want to close it and take the loss. I can afford to cut losses up to 1% of the account balance or $100. So, that's the reason I want to add this condition to my EA. I hope it's clear now.
I am also trying to tell you that your calculus is incorrect:
double NewspercentageThreshold = 0.01 * negative_equity_percentage * Account_balance;
This will return a percentage of the account. So if your floating balance is equity then your equity will always be higher than this :
NewspercentageThreshold
Even with both conditions :
if ((floatingBalance < NewspercentageThreshold) || (floatingBalance < -negative_equity_balance))
That will never be true, if your nominal negative_equity_balance is $100 that will still be lower than the floating meaning that the condition wont be true, hence the logic being incorrect.
What you are looking for in comparison to what you've coded has no relevance.
You need a condition for when your equity reaches a certain point, cut losses by trying to exit at a smaller loss ie. $800 to $100. This is an entirely new logic.
yes. start with li'l is is old, and i dont even know if it works anymore.
OnStart and OnTick are used for different purposes too. OnTick is used if you want an EA. OnStart is used when you want a Script.
My suggestion to you was to move
CheckAndCloseTrade();
to the OnTick() function, and remove the OnStart() function.
You can add return(0); at end of OnTick() function, however, it is not needed.
The remainder of your posts, are confusing. I recommend that you pay a coder on Freelance to do it.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
Logic i am looking for :
If floating Balance is less than 1% in negative of account balance => Close the trade (OR) if floating balance is less than $100 in negative => Close the trade
Code :
Error : Balance, Equity and Floating balance do not change in Real time. Instead it takes the initial value when installing the EA and the values remains same.