- Need help to finish my EA
- [ARCHIVE] Any rookie question, so as not to clutter up the forum. Professionals, don't pass by. Nowhere without you - 3.
- newbie help
Here is the file
Use the code styler tool in your MetaEditor. Tools->Styler
It will indent your code to improve readability. You have some misarranged braces '{...}' so that order is modified/closed under wrong conditions.
Also you don't need to check 'if(res==true)' or 'if(res==false)' - just put 'if(res)' or 'if(!res)'.
I Take it you want the orders to close on the first tick of a new bar, correct ?
- Please edit your (original) post and use the CODE
button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - You should be able to read your code out loud and have it make sense. You would never write if( (2+2 == 4) == true) would you? if(2+2 == 4) is sufficient. So don't write if(bool == true), just use if(bool) or if(!bool). Code becomes self documenting when you use meaningful variable names, like bool isLongEnabled where as Long_Entry sounds like a trigger price or a ticket number and "if long entry" is an incomplete sentence.
-
static int ticket = 0; int start (){ { static bool IsFirstTick = true; if(IsFirstTick == true) { IsFirstTick = false; bool res; res = OrderSelect(ticket, SELECT_BY_TICKET);
On the first tick, ticket is zero. When will this OrderSelect ever be true? EAs must be coded to recover. If the power fails, OS crashes, terminal or chart is accidentally closed, on the next tick, any static/global ticket variables will have been lost. You will have an open order but don't know it, so the EA will never try to close it, trail SL, etc. How are you going to recover? Use a OrderSelect loop to recover, or persistent storage (GV+flush or files) of ticket numbers required. -
ticket = OrderSend(Symbol(),OP_BUY, Lots, Ask, 0, 0, 0, "Set by Open Close System"); ⋮ res1 = OrderModify(ticket, 0,Bid-StopLoss*Point,Bid+TakeProfit*Point,0);
There is no need to open an order and then set the stops. Simplify your code - do it in one step. TP/SL on OrderSend has been fine for years.
Build 500 № 9 2013.05.09
Need help me mql4 guru add take profit to this EA - Take Profit - MQL4 programming forum 2017.09.27 -
int init(){ lastbar=Time[1];
Global and static variables work exactly the same way in MT4/MT5/C/C++.- They are initialized once on program load.
- They don't update unless you assign to them.
- In C/C++ you can only initialize them with constants, and they default to zero.
- In MTx you should only initialize them with constants.
There is no
default in MT5 (or MT4 with strict
which you should always use.)
MT4/MT5 actually compiles with non-constants, but the order that they are initialized is unspecified and don't try to use any price or server related functions in OnInit (or on load,) as there may be no connection/chart yet:
- Terminal starts.
- Indicators/EAs are loaded. Static and globally declared variables are initialized. (Do not depend on a specific order.)
- OnInit is called.
- For indicators OnCalculate is called with any existing history.
- Human may have to enter password, connection to server begins.
- New history is received, OnCalculate called again.
- New tick is received, OnCalculate/OnTick is called. Now TickValue, TimeCurrent, account information and prices are valid.
- Unlike indicators, EAs are not reloaded on chart change so you must reinitialize them, if necessary.
external static variable - Inflation - MQL4 programming forum
There's several issues with your code. Also it's unclear what you want to achieve and this would mean not only fix the obvious errors but also make a guess on your strategy.
I suggest to get someone fix it according to your needs. https://www.mql5.com/en/job
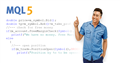
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use