Error 1: Missing Definitions
- The code references functions like TimeCurrent() , gmtime() , PositionsTotal() , PositionGet() , OrderClose() , Print() , GetLastError() , EMPTY , and RET_OK without their definitions. These functions are part of the MQL5 standard library and need to be properly included.
Error 2: Incorrect Function Usage
- The usage of gmtime() is incorrect. In MQL5, you should use TimeToStruct() to convert a time_t value to a datetime structure.
Error 3: Undefined Variables
- Variables like position_info , position , and ticket are not defined in the code. You need to define these structures or use the appropriate MQL5 functions to access position information.
Error 4: Incorrect Condition
- The condition position.bid ? position.bid : position.ask is not valid. You should use position.bid or position.ask directly based on your logic.
Error 5: Incorrect Print Statement
- The Print() function should have a proper format for error messages. Consider using Print("Error closing position: ", GetLastError()); for correct output.
For more detailed guidance you can explore https://www.mql5.com/en/articles/100
Good luck with your coding experiments with AI under the hood!
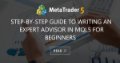
- www.mql5.com
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)
ChatGPT (the worst), “Bots Builder”, “EA builder”, “EA Builder Pro”, EATree, “Etasoft forex generator”, “Forex Strategy Builder”, ForexEAdvisor (aka. ForexEAdvisor STRATEGY BUILDER, and Online Forex Expert Advisor Generator), ForexRobotAcademy.com, forexsb, “FX EA Builder”, fxDreema, Forex Generator, FxPro, “LP-MOBI”, Molanis, “Octa-FX Meta Editor”, Strategy Builder FX, “Strategy Quant”, “Visual Trader Studio”, “MQL5 Wizard”, etc., are all the same. You will get something quick, but then you will spend a much longer time trying to get it right, than if you learned the language up front, and then just wrote it.
Since you haven't learned MQL4/5, therefor there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into yours.
We are willing to HELP you when you post your attempt (using Code button) and state the nature of your problem, but we are not going to debug your hundreds of lines of code. You are essentially going to be on your own.
ChatGPT |
|
bot builder | Creating two OnInit() functions. * |
EA builder | |
EATree | Uses objects on chart to save values — not persistent storage (files or GV+Flush.) No recovery (crash/power failure.) |
ForexEAdvisor |
|
FX EA Builder |
|
hi guys,
i wanted an EA that closes trades at a fixed time, so I asked AI to write me a mql5 code for that.
the problem is that when i put it into meta editor and hit compile, it returns with errors... errors i have no idea what they mean or how to fix them.
im hoping someone can look at the errors and fix it please!
this is the code written by AI
Never use generators or ChatGpt for coding, it will not be complete, Chat gpt does not understand the syntax, if you give us an Ai generated code we will not be able to assist you. We can see when its hand written, and when its Ai generated.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi guys,
i wanted an EA that closes trades at a fixed time, so I asked AI to write me a mql5 code for that.
the problem is that when i put it into meta editor and hit compile, it returns with errors... errors i have no idea what they mean or how to fix them.
im hoping someone can look at the errors and fix it please!
this is the code written by AI