...
Improperly formatted code removed by moderator. Please EDIT your post and use the CODE button (Alt-S) when inserting code.
Hover your mouse over your post and select "edit" ...
//+------------------------------------------------------------------+ //| Returns true 1-2 hours before Fx market closing on Friday | //+------------------------------------------------------------------+ bool TimeFridayEOW() { MqlDateTime dt; TimeToStruct(TimeGMT(), dt); //--- skip opening new trades after 8:00 pm (GMT) to have enough time for exit if(dt.day_of_week == (ENUM_DAY_OF_WEEK)FRIDAY && dt.hour >= 20) // FX closes Fri, 10:00 PM UTC in winter (and Fri, 09:00 PM UTC in summer) { return(true); } return(false); }
The Forex week lasts from Sun. 17:00 - Fri. 17:00 (NY time) that is in seconds:
#define FXOneWeek 432000 // Sun. 22:00 - Fri. 22:00 = 5*24*60*60 = 432000
So detect the first forex trading hour of the actual week of the the broker time (TimeCurrent()) and
add to this time (5*24*60*60 - 2*3600) seconds to get the timestamp of two hours before the forex session closes,
no matter whether DST of NY, your broker, or the location of the pc/server is 'on' or 'out' .
PS: It is better to use EURUSD for this, as gold can have different settings depending on the broker!
The Forex week lasts from Sun. 17:00 - Fri. 17:00 (NY time) that is in seconds:
#define FXOneWeek 432000 // Sun. 22:00 - Fri. 22:00 = 5*24*60*60 = 432000
So detect the first forex trading hour of the actual week of the the broker time (TimeCurrent()) and
add to this time (5*24*60*60 - 2*3600) seconds to get the timestamp of two hours before the forex session closes,
no matter whether DST of NY, your broker, or the location of the pc/server is 'on' or 'out' .
PS: It is better to use EURUSD for this, as gold can have different settings depending on the broker!
One can also look at this tutorial https://www.youtube.com/watch?v=mQtluW5hgC8 for a professional approach to close trade(s) at weekend.
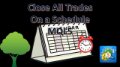
- 2023.02.24
- www.youtube.com
One can also look at this tutorial https://www.youtube.com/watch?v=mQtluW5hgC8 for a professional approach to close trade(s) at weekend.
For more precise control of the weekend:
//+-------------------------------------------------------------------+ //| Time remaining in seconds till the next weekend. | //| Returns 0 remaining sec, if called during the weekend. | //+-------------------------------------------------------------------+ int SecTillWeekend(void) { datetime now = TimeGMT(); datetime sunday = now-(now+345600)%604800; // Begin of Week 2017.08.03 11:30 => Sun, 2017.07.30 00:00 //--- US summer starts on the second Sunday of March //--- and ends before the first Sunday of November. MqlDateTime dt; TimeToStruct(sunday, dt); datetime dst_start = StringToTime((string)dt.year+".03.08"); datetime dst_end = StringToTime((string)dt.year+".11.01"); int hour = (sunday>=dst_start && sunday<dst_end) ? 21 : 22; datetime open = sunday + hour*3600; // FX opens Sun, 22:00 UTC in winter (and Sun, 21:00 UTC in summer) datetime close = open + 5*24*3600; // FX closes Fri, 22:00 UTC in winter (and Fri, 21:00 UTC in summer) int remaining = 0; if(now >= open && now < close) { remaining = (int)(close - now) - 1; } return(remaining); }
possible uses:
input int pHoursBefore = 2; // Hours before weekend input int pMinutesBefore = 0; // Minutes before weekend void OnTick() // or OnTimer() { //--- close before the weekend if(SecTillWeekend() <= pHoursBefore*3600 + pMinutesBefore*60) { // CloseAll(); } //--- get the start of the next weekend if(SecTillWeekend() != 0) { Print("Start of weekend is ", TimeToString(TimeCurrent() + SecTillWeekend())); } }
Based on SecTillWeekend():
//+------------------------------------------------------------------+ //| Returns true if time now is pHour and pMinute before weekend. | //+------------------------------------------------------------------+ bool CheckBeforeWeekend(int pHoursBefore, int pMinutesBefore) { int secs = SecTillWeekend(); return(secs > 0 && secs <= pHoursBefore*3600 + pMinutesBefore*60); }
Usage:
void OnTick() // or OnTimer() { //--- close before the weekend if(CheckBeforeWeekend(pHoursBefore, pMinutesBefore)) { CloseAll(); } }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone!
Now I want to send to all the method to close all positions trade at time end of weekness. Detail as below:
RESULT:
BEFORE:
AFTER: