This was already discussed on another of your threads — SYMBOL_TRADE_TICK_VALUE_LOSS vs SYMBOL_TRADE_TICK_VALUE_PROFIT - How to calculate position size based on entry price and stop loss price
Consider using OrderCalcProfit instead.
EDIT: If however, the OrderCalcProfit function does not work correctly either, then contact the broker. But make sure, it is not a bug in your code.Forum on trading, automated trading systems and testing trading strategies
SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_VALUE) sometimes zero
Fernando Carreiro, 2022.08.23 17:41
You can! These are the steps I take. I supply the function with a lot size equal to the “Max Lot Size” allowed for the symbol in question, then calculate the ratio needed to achieve the fractional risk that I wish to apply, to get the correct volume for the order. I then align that with the “Lot Step” and finally check it against both the maximum and minimum allowed lots for the symbol.
The reason I use the “maximum” lots instead of just “1.0” lots as a reference value is because there is no guarantee that the value of 1.0 is within the minimum and maximum values allowed. Given that using 1.0, or the maximum, gives equivalent results anyway (by using the ratio method), I choose to use the “max lots” as the reference point which also offers the most precision for the calculation.
Something like this ...
// This code will not compile. It is only a example reference if( OrderCalcProfit( eOrderType, _Symbol, dbLotsMax, dbPriceOpen, dbPriceStopLoss, dbProfit ) ) { dbOrderLots = fmin( fmax( round( dbRiskMax * dbLotsMax / ( -dbProfit * dbLotsStep ) ) * dbLotsStep, dbLotsMin ), dbLotsMax ); // the rest of the code ... };
This was already discussed on another of your threads — SYMBOL_TRADE_TICK_VALUE_LOSS vs SYMBOL_TRADE_TICK_VALUE_PROFIT - How to calculate position size based on entry price and stop loss price
Consider using OrderCalcProfit instead.
EDIT: If however, the OrderCalcProfit function does not work correctly either, then contact the broker. But make sure, it is not a bug in your code.
Thanks but although the titles are similar they don't point to the same issue. That other thread was about the values being off by amounts so small that it barely affected your lot size. This issue now is that the value returns a number as if the contract size was 1 but the actual contract size is 10 so the tick value returned is 10 times too small and produces lot size calculations that are 10 times too large. I think OrderCalcProfit works correctly though, it's really just the tick value property that's wrong. I could change my code to use that instead, now that I can't trust the broker to enter the correct information, I am strongly considering it.
edit: to be clear, this issue affects all tick value properties for these symbols with that broker (SYMBOL_TRADE_TICK_VALUE, SYMBOL_TRADE_TICK_VALUE_LOSS and SYMBOL_TRADE_TICK_PROFIT). So it's as if the tick value returned is forgetting that the contract size is 10 instead of 1.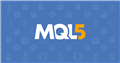
- www.mql5.com
I wrote to the broker but no response yet. I know we can't discuss specific brokers here so I don't think I can give you access to credentials to test the issue (they have a demo account made for people to go see their spreads in MT5) but if I'm wrong and you'd like to test your code with them then let me know.
I wrote to the broker but no response yet. I know we can't discuss specific brokers here so I don't think I can give you access to credentials to test the issue (they have a demo account made for people to go see their spreads in MT5) but if I'm wrong and you'd like to test your code with them then let me know.
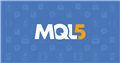
- www.mql5.com
True, right now I'm converting the code to use OrderCalcProfit with the max_volume to get more precision. It's nice to know that all that time I spent last year understanding the formulas for tick value calculations have been a complete waste of time lol! Oh well... You would think that tick_value should always equal to the value of 1 tick on 1 lot, but apparently that's not the case with my new broker, at least not for their CFDINDEX calc mode (the way it's setup there is that the tick value is the value of 1 tick on one unit instead of the tick value on one contract). It could also be a mistake on their end or an issue with MQL5 documentation, but in the end, all that matters is that my code runs smoothly so I will convert everything to avoid busting an account because of a calculation error.
Is there any documentation that tells us what SYMBOL_TRADE_TICK_VALUE is supposed to represent? I know it says "Calculated tick price for a losing position" but that doesn't tell me about the size of the position...
I mean... I always thought it was "the value of a 1 tick price change for a 1 lot position, in the account's currency".
But is there any documentation that confirms this or are we just assuming this is the case? Because that's clearly not what it represents with the CFDINDEX instruments at my current broker so are they the ones making a mistake or am I the one making a wrong assumption?
PS: SYMBOL_CALC_MODE_CFDINDEX instruments at my current broker use SYMBOL_TRADE_TICK_VALUE to represent "the value of a 1 tick price change per unit of the base currency for a given position, in the account's currency", so for example with a contract size of 10 units per lot, the value of a 1 tick price change on a 1 lot position = 10 * SYMBOL_TRADE_TICK_VALUE.
Is there any documentation that tells us what SYMBOL_TRADE_TICK_VALUE is supposed to represent? I know it says "Calculated tick price for a losing position" but that doesn't tell me about the size of the position...
I mean... I always thought it was "the value of a 1 tick price change for a 1 lot position, in the account's currency".
But is there any documentation that confirms this or are we just assuming this is the case? Because that's clearly not what it represents with the CFDINDEX instruments at my current broker so are they the ones making a mistake or am I the one making a wrong assumption?
PS: SYMBOL_CALC_MODE_CFDINDEX instruments at my current broker use SYMBOL_TRADE_TICK_VALUE to represent "the value of a 1 tick price change per unit of the base currency for a given position, in the account's currency", so for example with a contract size of 10 units per lot, the value of a 1 tick price change on a 1 lot position = 10 * SYMBOL_TRADE_TICK_VALUE.
Ok thanks, I will use OrderCalcProfit to calculate the values I need instead of using SYMBOL_TRADE_TICK_VALUE_LOSS or SYMBOL_TRADE_TICK_VALUE_PROFIT. It seems much more reliable. Already made the changes and it fixed the issue.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Alain Verleyen once wrote in another thread that some brokers provide the wrong value. I think my broker is doing this. What should I do? Should I tell them or should I change my code to adapt to it?
What happens is the EA calculates lot sizes for a position based on the tick value returned by SYMBOL_TRADE_TICK_VALUE_LOSS, but the value this variable returns is 10 times too small so it increases the lot size by 10x which is a really big problem (lucky to have spotted it).
Basically, on their US indices (CFD INDEX), their contract size is 10 and their tick size is 0.01, but the tick value returned by SYMBOL_TRADE_TICK_VALUE_LOSS is 0.01
I'm not going crazy am I? SYMBOL_TRADE_TICK_VALUE_LOSS should return 0.1 right? Basically contract size x tick size. (account currency is same as quote currency for these indices)
I checked and when you open a trade the tick value really is 0.1
Am I wrong? Is this what Alain was taking about when he said that some brokers provide the wrong value?
What are we supposed to do when that happens?
Thanks in advance