Please initialize your buffers.
PlotIndexSetDouble(index, PLOT_EMPTY_VALUE, 0);
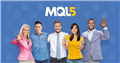
- www.mql5.com
Thank you for your suggestion, I have initialized the buffers like you said, but the indicator still flatten.
int OnInit() { string IndicatorShortName = " "; SetIndexBuffer(0,iATRBuffer,INDICATOR_DATA); SetIndexBuffer(1,TemaBuffer,INDICATOR_DATA); SetIndexBuffer(2,Ema,INDICATOR_CALCULATIONS); SetIndexBuffer(3,EmaOfEma,INDICATOR_CALCULATIONS); SetIndexBuffer(4,EmaOfEmaOfEma,INDICATOR_CALCULATIONS); PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0); Han_ATR = iATR(_Symbol,PERIOD_CURRENT,Inp_ATR_Period); //--- sets first bar from what index will be drawn PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,0); PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,0); PlotIndexSetInteger(0,PLOT_SHOW_DATA,ShowSignalData); PlotIndexSetInteger(1,PLOT_SHOW_DATA,ShowSignalData); if(ShowSignalData) { PlotIndexSetString(0,PLOT_LABEL,"ATR "+string(Inp_ATR_Period)); PlotIndexSetString(1,PLOT_LABEL,"ATR-TEMA "+string(Inp_Period_TEMA)); IndicatorShortName = "TEMA-ATR "+string(Inp_ATR_Period)+" "+string(Inp_Period_TEMA); } IndicatorSetString(INDICATOR_SHORTNAME,IndicatorShortName); if(Han_ATR==INVALID_HANDLE) { PrintFormat("Failed to create handle of the iATR indicator for the symbol %s/%s, error code %d", _Symbol, EnumToString(PERIOD_CURRENT), GetLastError()); return(INIT_FAILED); } return(INIT_SUCCEEDED); }
Any other suggestions?
Please don't create topics randomly in any section. It has been moved to the section: Technical Indicators
Thanks for the suggestion, this is my first time posting a question in this forum. I'll pay attention to it next time
You have five buffers. Initialize all of them. I have not tested your code. This could help
I have tried it, but the result remains the same Here is my new code according to your suggestion: int OnInit()
{
string IndicatorShortName = " ";
SetIndexBuffer(0,iATRBuffer,INDICATOR_DATA);
SetIndexBuffer(1,TemaBuffer,INDICATOR_DATA);
SetIndexBuffer(2,Ema,INDICATOR_CALCULATIONS);
SetIndexBuffer(3,EmaOfEma,INDICATOR_CALCULATIONS);
SetIndexBuffer(4,EmaOfEmaOfEma,INDICATOR_CALCULATIONS);
PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(2,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(3,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(4,PLOT_EMPTY_VALUE,0.0);
Han_ATR = iATR(_Symbol,PERIOD_CURRENT,Inp_ATR_Period);
PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,0);
PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,0);
PlotIndexSetInteger(0,PLOT_SHOW_DATA,ShowSignalData);
PlotIndexSetInteger(1,PLOT_SHOW_DATA,ShowSignalData);
if(ShowSignalData)
{
PlotIndexSetString(0,PLOT_LABEL,"ATR "+string(Inp_ATR_Period));
PlotIndexSetString(1,PLOT_LABEL,"ATR-TEMA "+string(Inp_Period_TEMA));
IndicatorShortName = "TEMA-ATR "+string(Inp_ATR_Period)+" "+string(Inp_Period_TEMA);
}
IndicatorSetString(INDICATOR_SHORTNAME,IndicatorShortName);
if(Han_ATR==INVALID_HANDLE)
{
PrintFormat("Failed to create handle of the iATR indicator for the symbol %s/%s, error code %d",
_Symbol,
EnumToString(PERIOD_CURRENT),
GetLastError());
return(INIT_FAILED);
}
return(INIT_SUCCEEDED);
}
And here is the result, there is something weird with the data:
Any suggestion ?
-
PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(2,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(3,PLOT_EMPTY_VALUE,0.0); PlotIndexSetDouble(4,PLOT_EMPTY_VALUE,0.0);
That is not initializing buffers. It is just setting what value will be used for do not show.
-
int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]){ int iBar = rates_total - MathMax(FastLength, prev_calculated); while(iBar > 0){ --iBar; iATRBuffer[iBar] = TemaBuffer[iBar] = Ema[iBar] = EmaOfEma[iBar] = EmaOfEmaOfEma[iBar] = EMPTY_VALUE; // Initialize buffers // Compute buffers } // iBar //---- #define REDRAW_BAR_LAST (iBar == 0) // Redraw bar last? return rates_total-iBar-REDRAW_BAR_LAST; } // OnCalculate
If you do not set all buffers per candle, initialize each candle.
-
That is not initializing buffers. It is just setting what value will be used for do not show.
- If you do not set all buffers per candle, initialize each candle.
Hi, thank you for your reply. I finally figured out the problem based on your reply specifically this line :
int iBar = rates_total - MathMax(FastLength, prev_calculated);
So, I changed this line in my code :
ExponentialMAOnBuffer(rates_total,prev_calculated,0,Inp_Period_TEMA,iATRBuffer,Ema);
To this :
ExponentialMAOnBuffer(rates_total,prev_calculated,Inp_Period_TEMA+1,Inp_Period_TEMA,iATRBuffer,Ema);
That solved the problem for me, and this case is officially closed. Thank you

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi folks,
I need some help with my indicator, because the indicator drawing is flatten in weekly and monthly chart as shown below :
This is typically how it looks like in time frame H1 :
And here is my code :
I have completely no clue what is wrong with my code and i did not see any error messages after compiling. I have arround 600 bars in my monthly chart and 2000 bars in my weekly chart, so i think the data is more than enough to plot the indicator as it only uses 10 periods of the chart.
Please help folks, thank you.