I don't really understand what you try to get. :(
This
{ Buffer2[5+i] = High[5+i]; //Set indicator value at Candlestick High if(i == 1 && Time[1] != time_alert) myAlert("indicator", "Sell"); //Alert on next bar open time_alert = Time[1]; } else { Buffer2[i] = EMPTY_VALUE; }
looks strange. A value is assigned to Buffer2[ 5+i ] or otherwise Buffer2[ i ] = EMPTY_VALUE;
It looks a bit like fractals do you know it...
Another example, i want to compare the current SAR value with the last Sar value to say:
if(first SAR value > first last SAR value)
CopyBuffer() is what you are lo0oking for - I guess.
Look at the example and imagine iSAR in stead if iMA.
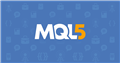
- www.mql5.com
CopyBuffer() is what you are lo0oking for - I guess.
Look at the example and imagine iSAR in stead if iMA.
I tried to do this with ZigZag indicator by this function, but it is failed!
double ZagZag(int Q) { int First=0; for(int i=0;i<7777;i++) { if(ZigZag_handle!=0)First++; if(First==1&&Q==0&&ZigZag_handle!=0){return(ZigZag_handle);} if(First==2&&Q==1&&ZigZag_handle!=0){return(ZigZag_handle);} if(First==3&&Q==2&&ZigZag_handle!=0){return(ZigZag_handle);} if(First==4&&Q==3&&ZigZag_handle!=0){return(ZigZag_handle);} if(First==5&&Q==4&&ZigZag_handle!=0){return(ZigZag_handle);} if(First==6&&Q==5&&ZigZag_handle!=0){return(ZigZag_handle);} } return(0); }
I told you to look at the example there - you didn't!
What you did is just a mess.
One handle in OnInit and CopyBuffer in OnTick (or even in OnCalculate) where is that structure?
May you should read this:
Step-by-step for beginners: https://www.mql5.com/en/articles/100
quickentry: https://www.mql5.com/en/articles/496
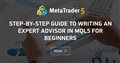
- www.mql5.com
I told you to look at the example there - you didn't!
What you did is just a mess.
One handle in OnInit and CopyBuffer in OnTick (or even in OnCalculate) where is that structure?
May you should read this:
Step-by-step for beginners: https://www.mql5.com/en/articles/100
quickentry: https://www.mql5.com/en/articles/496
Here i made CopyBuffer for SAR
#property indicator_chart_window #property indicator_buffers 2 #property indicator_plots 2 #property indicator_type1 DRAW_ARROW #property indicator_width1 3 #property indicator_color1 0xFF901E #property indicator_label1 "Buy" #property indicator_type2 DRAW_ARROW #property indicator_width2 3 #property indicator_color2 0xFF00FF #property indicator_label2 "Sell" //--- indicator buffers double Buffer1[]; double Buffer2[]; double myPoint; //initialized in OnInit double Open[]; double Close[]; double Low[]; double High[]; int SAR_handle; double SAR[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { SetIndexBuffer(0, Buffer1); PlotIndexSetDouble(0, PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetInteger(0, PLOT_ARROW, 161); SetIndexBuffer(1, Buffer2); PlotIndexSetDouble(1, PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetInteger(1, PLOT_ARROW, 161); //initialize myPoint myPoint = Point(); if(Digits() == 5 || Digits() == 3) { myPoint *= 10; } SAR_handle = iSAR(NULL, PERIOD_CURRENT, 0.02, 0.2); if(SAR_handle < 0) { Print("The creation of iSAR has failed: SAR_handle=", INVALID_HANDLE); Print("Runtime error = ", GetLastError()); return(INIT_FAILED); } return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime& time[], const double& open[], const double& high[], const double& low[], const double& close[], const long& tick_volume[], const long& volume[], const int& spread[]) { int limit = rates_total - prev_calculated; //--- counting from 0 to rates_total ArraySetAsSeries(Buffer1, true); ArraySetAsSeries(Buffer2, true); //--- initial zero if(prev_calculated < 1) { ArrayInitialize(Buffer1, EMPTY_VALUE); ArrayInitialize(Buffer2, EMPTY_VALUE); } else limit++; datetime Time[]; if(BarsCalculated(SAR_handle) <= 0) return(0); if(CopyBuffer(SAR_handle, 0, 0, rates_total, SAR) <= 0) return(rates_total); ArraySetAsSeries(SAR, true); if(CopyOpen(Symbol(), PERIOD_CURRENT, 0, rates_total, Open) <= 0) return(rates_total); ArraySetAsSeries(Open, true); if(CopyClose(Symbol(), PERIOD_CURRENT, 0, rates_total, Close) <= 0) return(rates_total); ArraySetAsSeries(Close, true); if(CopyLow(Symbol(), PERIOD_CURRENT, 0, rates_total, Low) <= 0) return(rates_total); ArraySetAsSeries(Low, true); if(CopyHigh(Symbol(), PERIOD_CURRENT, 0, rates_total, High) <= 0) return(rates_total); ArraySetAsSeries(High, true); if(CopyTime(Symbol(), Period(), 0, rates_total, Time) <= 0) return(rates_total); ArraySetAsSeries(Time, true); //--- main loop for(int i = limit-1; i >= 0; i--) { if (i >= MathMin(200000-1, rates_total-1-50)) continue; //omit some old rates to prevent "Array out of range" or slow calculation //Indicator Buffer 1 if(true ) { Buffer1[5+i] = Low[5+i]; //Set indicator value at Candlestick Low } else { Buffer1[i] = EMPTY_VALUE; } //Indicator Buffer 2 if(true ) { Buffer2[5+i] = High[5+i]; //Set indicator value at Candlestick High } else { Buffer2[i] = EMPTY_VALUE; } } return(rates_total); } //+------------------------------------------------------------------+
So how can i write this condition :
if(first SAR value > first last SAR value)
Can you read or do you only know how to copy and paste?
Here is decribed that CopyBuffer fills an array with values, now you have to access the values in the filled array. This is described and explained in the a.m. articles:
Step-by-step for beginners: https://www.mql5.com/en/articles/100
quickentry: https://www.mql5.com/en/articles/496
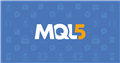
- www.mql5.com
Can you read or do you only know how to copy and paste?
Here is decribed that CopyBuffer fills an array with values, now you have to access the values in the filled array. This is described and explained in the a.m. articles:
Step-by-step for beginners: https://www.mql5.com/en/articles/100
quickentry: https://www.mql5.com/en/articles/496
There are 3 variants of function calls:
Call by the first position and the number of required elements
int CopyBuffer( |
Call by the start date and the number of required elements
int CopyBuffer( |
Call by the start and end dates of a required time interval
int CopyBuffer( |
---------------
I guess what I'm asking is not in line with these three.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello, i have an indicator that gives me signals (buy and sell).
I want to determine if the current signal is buy and the preview signal is sell and the preview signal is buy, drawing lines between these signals as the picture to link the last 2 signals with the current one.
How can i do this simply?