-
if(dow != 6 && dow != 0) return((datetime)i);
This fails as it depends on the timezone of the broker.Brokers use a variety of time zones. Their local time, with or without Daylight Savings Time (DST), London, UTC, London+2, UTC+2, NY+7.
Only with NY+7 does the broker's 00:00 equals 5 PM ET and the start of a daily bar (and H4) is the start of a new FX day.
GMT/BST brokers, means there is a 1 or 2 hour D1/H4 bar on Sunday (depending on NY DST), and a short Friday bar. (Problems with indicators based off bars.)
- Then there are market holiday (country and broker specific), requires knowledge of when your broker stops and starts (not necessary the same as the market.)
"Free-of-Holes" Charts - MQL4 Articles (2006)
No candle if open = close ? - MQL4 programming forum (2010)
- This fails as it depends on the timezone of the broker.
- Then there are market holiday (country and broker specific), requires knowledge of when your broker stops and starts (not necessary the same as the market.)
"Free-of-Holes" Charts - MQL4 Articles (2006)
No candle if open = close ? - MQL4 programming forum (2010)
Hi William, yes you are correct of course. Thank you for the important feedback.
- For this point, while researching possible approaches, I happened on Carl Schreiber/gooly's articles on similar matters:
https://www.mql5.com/en/articles/9929
He provides some functionality and including his work may allow smoother methods to manage this (server times and daylight times). There might be a "dumb" but acceptable method if I pass a string with "exclusion days" to the function to allow for Sunday trading (right now, my trading preference is to avoid Sundays)// exclusion days passed to functions... eh, I think there must be a more elegant way. pass a pipe sep string like "6" or "0|1|5|6" in the exclude variable to create the trading exclusion days // int TradingDayOfMonth(datetime datetime_in=0, string exclude="0|6") { if(datetime_in <= 3) datetime_in = TimeSource((int)datetime_in); int first = (int)FirstDateOfMonth(datetime_in); int t_days = 0; datetime datetime_end = datetime_in; return(TDOMLoop(first, datetime_end, t_days, exclude)); } // // // int TDOMLoop(int first_in, datetime datetime_end_in, int t_days_in, string exclude="0|6") { int t_days = t_days_in; int day = 86400; // a day's worth of seconds for(int i = first_in; i <= datetime_end_in; i = i+day) { int dow = DayOfWeek(i); if(MemberOfSet(dow,exclude)!=1 && MemberOfSet(dow,exclude)!=-1) t_days++; } return(t_days); } // // // int MemberOfSet(int this_value_in, string ints_as_string_in) { string set_arr[]; string sep = "|"; ushort u_sep = StringGetCharacter(sep,0); StringSplit(ints_as_string_in,u_sep,set_arr); if(ArraySize(set_arr)<1) { Print("Error: weekend exclusion days are blank"); return(-1); } // 0-6 are chars 48-54 for(int i = 0; i < ArraySize(set_arr); i++) { int test = (StringGetCharacter(set_arr[i],0)>=48 && StringGetCharacter(set_arr[i],0) <=54) ? (int)StringToInteger(set_arr[i]) : -1; if(test < 0 || test > 6) { Print("Error: Numbers outside of weekdays range (0 through 6), or wrong character"); return(-1); } if(this_value_in==test) return(1); // a member of the set } return(0); // not a member of the set }
- Yes, holidays. I have some coarse management for holidays elsewhere in my code (somewhat outside the scope of this exercise above, but compatible.) It works, but I am not completely happy with what I have. There is this article from Dennis K, which looks interesting and may provide some better guidance,
https://www.mql5.com/en/articles/9874
But I am guessing that there will always be some gaps.
Another main challenge for me is that I have a regular day-job too, and I am always pinched for time. There is also my "bigger" project, my own EAs I am developing for myself, I don't want to get too far afield of what's needed to complete them. I must pick and choose very carefully what I work on next.
But, for sure I will have to look into solutions to both of your points in more detail. I don't want my personal preferences to keep me naive on these issues.
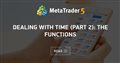
- www.mql5.com
By the way, William R - how do you get the color coding in the code section?
it would definitely make my code blocks easier to read, but I don't see a control for it. I just used the general code styling
By using the built-in code pasting functionality instead of selecting the "Code" Style — [Alt-S] instead of [Ctrl-S].
By using the built-in code pasting functionality instead of selecting the "Code" Style — [Alt-S] instead of [Ctrl-S].
Fernando! Simple but brilliant, thank you for saving me from eternal embarrassment! :D
I have updated the code blocks to make it easier on the eyes. Happy New Year!Hi there, thank you for sharing this very handy set of time and date functions! As a fellow coder just getting into MQL, I really appreciate you posting your code and detailed explanations.
The trading day of month calculation is super useful. I've also struggled with trying to properly account for weekends and holidays when doing date math in MQL. Your loop approach to iterate day by day makes total sense.
Some other features I like are the easy TimeLocal vs TimeGMT switching, date range checking, and first/last day of month. Lots of practical usage here!
I tested out a few scenarios and didn't catch any obvious bugs. The logic looks solid. Some minor suggestions:
- Add comments above each function explaining its purpose
- Use more descriptive parameter names like start_date and end_date instead of datetime_start etc.
- Consider saving into an include file to easily calendar across EAs
Hi there, thank you for sharing this very handy set of time and date functions! As a fellow coder just getting into MQL, I really appreciate you posting your code and detailed explanations.
The trading day of month calculation is super useful. I've also struggled with trying to properly account for weekends and holidays when doing date math in MQL. Your loop approach to iterate day by day makes total sense.
Some other features I like are the easy TimeLocal vs TimeGMT switching, date range checking, and first/last day of month. Lots of practical usage here!
I tested out a few scenarios and didn't catch any obvious bugs. The logic looks solid. Some minor suggestions:
- Add comments above each function explaining its purpose
- Use more descriptive parameter names like start_date and end_date instead of datetime_start etc.
- Consider saving into an include file to easily calendar across EAs
Hi helin, I am very glad you found them useful. I don't often get the chance to help others on the forum.
I have made some mods to these and improved readability. But yes - for my own use, they live in a separate include file, and I often update my own comments (to remind myself what I did the last time... months ago!) and I have changed some of the param/variable names several times to help myself. It's a learning process for me. :)
Feel free to modify them for your uses as you will. I am not a pro coder, and I will not be updating these as a library for others to use. I just shared them for folks like you to pick them up if helpful.
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I wanted to try Larry Williams' Trading Day of Month strategies, but I had a hard time figuring out how to calculate the current trading day of the month accurately (basically similar in concept to business day calculations in Excel). I could not find code on this site for it ... I am SURE someone has already done it, although I may not have used the right search terms. So after searching around unsuccessfully, I made my own based on some C code on stackexchange. While I was at it I made several other short-cut functions for my own work. I am sharing it here with everyone in case others want to use it as a starting point.
My favorite functionalities here are:
I use TimeLocal() in my code because my workstation is set to EST, and that's how I track my times, so my default value in most of the functions is to use TimeLocal() if no datetime is passed to the function.
EDITED: Updated the functions somewhat to allow for:
Choice of times: still defaults to TimeLocal(), but can easily select TimeCurrent, TimeTradeServer, TimeGMT
Concision (somewhat): reduced some redundancy, simplified some functions
Enjoy. Happy New Year!
While I tested compilation in MT4 and MT5 on my instances, I cannot vouch for it being bullet proof... only that I did some QA on it and I cannot find obvious errors. But - if you find errors in it, please shout out and I will fix as best as I can. No promise that this is the most efficient code possible, I admit to some code duplication and clunky looking string management. Otherwise, the usual disclaimers: you are responsible for your own trading, no promise of fitness for use or functionality in trading, blah blah, yadda yadda. My apologies in advance if my coding is crap or if my naming conventions suck: I'm using MQL as my excuse to learn to code. I'm a level 2 n00b