Hi all
I have a problem when counting pending orders using C trade. It opens too many pending orders instead of one. If you know what the problem is, please help.
The CTrade class cannot read pending orders. You are confusing something.
Pending orders are counted using the COrderInfo trading class.
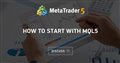
- 2020.03.12
- www.mql5.com
The CTrade class cannot read pending orders. You are confusing something.
If I use the function below, too many pending orders open still. How do i open just one pending order?
int OrdersPending() { int Pending=0; for(int b=OrdersTotal()-1;b>=0;b--) { string OrderSymbol=OrderGetString(ORDER_SYMBOL); int Magic=(int)OrderGetInteger(ORDER_MAGIC); bool Select=OrderSelect(OrderGetTicket(b)); if(OrderSymbol==Symbol()&&Magic==MagicNumber) { ENUM_ORDER_TYPE Type=(ENUM_ORDER_TYPE)OrderGetInteger(ORDER_TYPE); if(Type==ORDER_TYPE_BUY_STOP||Type==ORDER_TYPE_SELL_STOP) Pending++; } } return(Pending); }
Or you can generally use this function:
//+------------------------------------------------------------------+ //| Is pending orders exists | //+------------------------------------------------------------------+ bool IsPendingOrdersExists(void) { for(int i=OrdersTotal()-1; i>=0; i--) // returns the number of current orders if(m_order.SelectByIndex(i)) // selects the pending order by index for further access to its properties if(m_order.Symbol()==m_symbol.Name() && m_order.Magic()==InpMagic) return(true); //--- return(false); }
if it returns 'true', it means there are pending orders in the market.
Or you can generally use this function:
if it returns 'true', it means there are pending orders in the market.
I tried both count pending functions but it still opens too many pending orders. Here is my full code, maybe their might be a problem in my code.
#include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> #include <Trade\PositionInfo.mqh> #include <Trade\AccountInfo.mqh> #include <Trade\OrderInfo.mqh> #include <Trade\DealInfo.mqh> CTrade trade; CTrade m_trade; CPositionInfo m_position; CSymbolInfo m_symbol; COrderInfo m_order; CDealInfo m_deal; input int MagicNumber=01; //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ bool OrdersPending(void) { for(int b=OrdersTotal()-1;b>=0;b--) if(m_order.SelectByIndex(b)) if(m_order.Symbol()==m_symbol.Name()&&m_order.Magic()==MagicNumber) return(true); return(false); } void Buy() { double Pips=0; double TickSize=SymbolInfoDouble(Symbol(),SYMBOL_TRADE_TICK_SIZE); if(TickSize==0.00001||TickSize==0.001) Pips=TickSize*10; else Pips=TickSize; double Ask=SymbolInfoDouble(Symbol(),SYMBOL_ASK); double Bid=SymbolInfoDouble(Symbol(),SYMBOL_BID); double CloseBar=iClose(NULL,0,1); double CloseBarNext=iClose(NULL,0,2); double OpenBar=iOpen(NULL,0,1); double OpenBarNext=iOpen(NULL,0,2); double HighBar=iHigh(NULL,0,1); double HighBarNext=iHigh(NULL,0,2); double LowBar=iLow(NULL,0,1); double LowBarNext=iLow(NULL,0,2); double EngulfingBuy=LowBar<LowBarNext&&HighBar>HighBarNext&&CloseBar>OpenBarNext&&OpenBar<CloseBar&&OpenBarNext>CloseBarNext; double HaramiBuy=OpenBarNext>CloseBarNext&&CloseBar>OpenBar&&HighBarNext>HighBar&&OpenBarNext>CloseBar&&LowBarNext<LowBar; if(HaramiBuy) { trade.BuyStop(0.01,Ask+2*Pips,NULL,0,0,0,0,"JackBuda"); } else if(EngulfingBuy) { trade.BuyStop(0.01,Ask+2*Pips,NULL,0,0,0,0,"JackBuda"); } } void Sell() { double Pips=0; double TickSize=SymbolInfoDouble(Symbol(),SYMBOL_TRADE_TICK_SIZE); if(TickSize==0.00001||TickSize==0.001) Pips=TickSize*10; else Pips=TickSize; double Ask=SymbolInfoDouble(Symbol(),SYMBOL_ASK); double Bid=SymbolInfoDouble(Symbol(),SYMBOL_BID); double CloseBar=iClose(NULL,0,1); double CloseBarNext=iClose(NULL,0,2); double OpenBar=iOpen(NULL,0,1); double OpenBarNext=iOpen(NULL,0,2); double HighBar=iHigh(NULL,0,1); double HighBarNext=iHigh(NULL,0,2); double LowBar=iLow(NULL,0,1); double LowBarNext=iLow(NULL,0,2); double EngulfingSell=LowBar<LowBarNext&&HighBar>HighBarNext&&CloseBar<OpenBarNext&&OpenBar>CloseBar&&OpenBarNext<CloseBarNext; double HaramiSell=OpenBarNext<CloseBarNext&&CloseBar<OpenBar&&HighBarNext<HighBar&&OpenBarNext<CloseBar&&LowBarNext>LowBar; if(HaramiSell) { trade.SellStop(0.01,Bid-2*Pips,NULL,0,0,0,0,"JackBuda"); } else if(EngulfingSell) { trade.SellStop(0.01,Bid-2*Pips,NULL,0,0,0,0,"JackBuda"); } } void OnTick() { double AccountBalance=AccountInfoDouble(ACCOUNT_BALANCE); if(AccountBalance>10&&OrdersPending()<1){Buy();} if(AccountBalance>10&&OrdersPending()<1){Sell();} for(int Loop=PositionsTotal()-1;Loop>=0;Loop--) { if(m_position.SelectByIndex(Loop)) if(m_position.Symbol()==Symbol()) { double ProfitPips=PositionGetDouble(POSITION_VOLUME)*200; double Profit2=PositionGetDouble(POSITION_PROFIT); int Type=(int)OrderGetInteger(ORDER_TYPE); switch(Type) { case ORDER_TYPE_BUY:if(Profit2>ProfitPips) m_trade.PositionClose(m_position.Ticket()); case ORDER_TYPE_SELL:if(Profit2>ProfitPips) m_trade.PositionClose(m_position.Ticket()); } } } }
Where is the OnInit () function ???
I usually do not use it. Should I insert the count pending function in there?
You MUST wash your hands before eating. The advisor MUST have an OnInit () function. These are two laws.
You MUST wash your hands before eating. The advisor MUST have an OnInit () function. These are two laws.
Note taken. So for the count pending orders, it still opens too many orders.
#include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> #include <Trade\PositionInfo.mqh> #include <Trade\AccountInfo.mqh> #include <Trade\OrderInfo.mqh> #include <Trade\DealInfo.mqh> CTrade trade; CTrade m_trade; CPositionInfo m_position; CSymbolInfo m_symbol; COrderInfo m_order; CDealInfo m_deal; input int MagicNumber=01; //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ int OnInit(void) { return(0); } bool OrdersPending(void) { for(int b=OrdersTotal()-1;b>=0;b--) if(m_order.SelectByIndex(b)) if(m_order.Symbol()==m_symbol.Name()&&m_order.Magic()==MagicNumber) return(true); return(false); } void Buy() { double Pips=0; double TickSize=SymbolInfoDouble(Symbol(),SYMBOL_TRADE_TICK_SIZE); if(TickSize==0.00001||TickSize==0.001) Pips=TickSize*10; else Pips=TickSize; double Ask=SymbolInfoDouble(Symbol(),SYMBOL_ASK); double Bid=SymbolInfoDouble(Symbol(),SYMBOL_BID); double CloseBar=iClose(NULL,0,1); double CloseBarNext=iClose(NULL,0,2); double OpenBar=iOpen(NULL,0,1); double OpenBarNext=iOpen(NULL,0,2); double HighBar=iHigh(NULL,0,1); double HighBarNext=iHigh(NULL,0,2); double LowBar=iLow(NULL,0,1); double LowBarNext=iLow(NULL,0,2); double EngulfingBuy=LowBar<LowBarNext&&HighBar>HighBarNext&&CloseBar>OpenBarNext&&OpenBar<CloseBar&&OpenBarNext>CloseBarNext; double HaramiBuy=OpenBarNext>CloseBarNext&&CloseBar>OpenBar&&HighBarNext>HighBar&&OpenBarNext>CloseBar&&LowBarNext<LowBar; if(HaramiBuy) { trade.BuyStop(0.01,Ask+2*Pips,NULL,0,0,0,0,"JackBuda"); } else if(EngulfingBuy) { trade.BuyStop(0.01,Ask+2*Pips,NULL,0,0,0,0,"JackBuda"); } } void Sell() { double Pips=0; double TickSize=SymbolInfoDouble(Symbol(),SYMBOL_TRADE_TICK_SIZE); if(TickSize==0.00001||TickSize==0.001) Pips=TickSize*10; else Pips=TickSize; double Ask=SymbolInfoDouble(Symbol(),SYMBOL_ASK); double Bid=SymbolInfoDouble(Symbol(),SYMBOL_BID); double CloseBar=iClose(NULL,0,1); double CloseBarNext=iClose(NULL,0,2); double OpenBar=iOpen(NULL,0,1); double OpenBarNext=iOpen(NULL,0,2); double HighBar=iHigh(NULL,0,1); double HighBarNext=iHigh(NULL,0,2); double LowBar=iLow(NULL,0,1); double LowBarNext=iLow(NULL,0,2); double EngulfingSell=LowBar<LowBarNext&&HighBar>HighBarNext&&CloseBar<OpenBarNext&&OpenBar>CloseBar&&OpenBarNext<CloseBarNext; double HaramiSell=OpenBarNext<CloseBarNext&&CloseBar<OpenBar&&HighBarNext<HighBar&&OpenBarNext<CloseBar&&LowBarNext>LowBar; if(HaramiSell) { trade.SellStop(0.01,Bid-2*Pips,NULL,0,0,0,0,"JackBuda"); } else if(EngulfingSell) { trade.SellStop(0.01,Bid-2*Pips,NULL,0,0,0,0,"JackBuda"); } } void OnTick() { double AccountBalance=AccountInfoDouble(ACCOUNT_BALANCE); if(AccountBalance>10&&OrdersPending()<1){Buy();} if(AccountBalance>10&&OrdersPending()<1){Sell();} for(int Loop=PositionsTotal()-1;Loop>=0;Loop--) { if(m_position.SelectByIndex(Loop)) if(m_position.Symbol()==Symbol()) { double ProfitPips=PositionGetDouble(POSITION_VOLUME)*200; double Profit2=PositionGetDouble(POSITION_PROFIT); int Type=(int)OrderGetInteger(ORDER_TYPE); switch(Type) { case ORDER_TYPE_BUY:if(Profit2>ProfitPips) m_trade.PositionClose(m_position.Ticket()); case ORDER_TYPE_SELL:if(Profit2>ProfitPips) m_trade.PositionClose(m_position.Ticket()); } } } }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all
I have a problem when counting pending orders using C trade. It opens too many pending orders instead of one. If you know what the problem is, please help.