If you write one.
You can use this:
datetime timeCurrent = TimeCurrent();
int thisHour = TimeHour(timeCurrent), thisMinute = TimeMinute(timeCurrent);
And then use thisMinute in if-else condition:
if(thisMinute == 10){
//Do place order.
}
- Please edit your (original) post and use the CODE
button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - OP didn't ask anything about opening on ten minutes past the hour.
For BT purposes only on MT4
2. Not built-in, you need to program it. See SymbolInfoSessionTrade() if you need to determine market close by code.
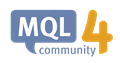
- docs.mql4.com
- Nothing to do with ticks. When something changes, the server sends an update, that is called a tick. The EA won't see it during the OrderSend,
but other charts will.
OrderSend sends the request, network delivers, placed in the server queue. Once it reaches the top of the queue, you get filled or not, and the result delivered back to the terminal. Normally it is the 20-200 milliseconds the network takes to deliver is the limiting factor, but it can take minutes to do a trade because of the servers (during news.)
- Write one. Compute when and then send.
datetime nextBar = Time[0] + PeriodSeconds(); datetime closeMinus10min = nextBar - 10*60; if(TimeCurrent() >= closeMinus10min){ Print("10 mins before close"); …}
- Nothing to do with ticks. When something changes, the server sends an update, that is called a tick. The EA won't see it during the OrderSend, but other charts will.
OrderSend sends the request, network delivers, placed in the server queue. Once it reaches the top of the queue, you get filled or not, and the result delivered back to the terminal. Normally it is the 20-200 milliseconds the network takes to deliver is the limiting factor, but it can take minutes to do a trade because of the servers (during news.)
- Write one. Compute when and then send.
Thanks. I added the code above to my EA, but when I BT it, there are no trades. I do get trades when the time requirement is removed. Not sure why this is happening. Can someone take a look? I know I have unmatched data errors. Still looking into solving that.
//+------------------------------------------------------------------+ //| Final.mq4 | //| Test | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Test" #property link "https://www.mql5.com" #property version "1.00" #property strict extern int Lb = 10; //input parameter for the indicator int ticket = 0; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { datetime nextBar = Time [0] + PeriodSeconds (); //Time[0] is the open time of the defined bar in seconds. //add 86400 secs for a day from PeriodSeconds //as markets are open 24 hours. If you use a //different time frame it will be different seconds. //Then you get end of day time with nextBar datetime closeMinus10secs = nextBar - 10; if (TimeCurrent() >= closeMinus10secs && OrdersTotal () == 0) //Check to see if is the last 10 secs before close and if there is an existing order. { double v1 = iCustom(NULL,0,"SSL_Channel_Chart",Lb,0,0); double v2 = iCustom(NULL,0,"SSL_Channel_Chart",Lb,1,0); double barv1 = iCustom(NULL,0,"SSL_Channel_Chart",Lb,0,1); double barv2 = iCustom(NULL,0,"SSL_Channel_Chart",Lb,1,1); if(v2 > v1 && barv2 <= barv1) // set buy criteria from indicator { ticket = OrderSend(NULL, OP_BUY, 1.0, Ask, 0, Ask - (iATR(NULL, 0, 14, 0)), Ask + (iATR(NULL, 0, 14,0)), NULL, 0, Green); // Open a buy order if(ticket > 0) Alert("The order number is: ", string(ticket)); } if (v2 < v1 && barv2 >= barv1) // set sell criteria from indicator { ticket = OrderSend (NULL, OP_SELL, 1.0, Bid, 0, Bid + (iATR(NULL, 0, 14,0)), Bid - (iATR(NULL, 0, 14, 0)) , NULL, 0, Red); //Open a sell order if(ticket > 0) Alert("The order number is: ", string(ticket)); } } }
ticket = OrderSend (NULL, OP_SELL, 1.0, Bid, 0, Bid + (iATR(NULL, 0, 14,0)), Bid - (iATR(NULL, 0, 14, 0)) , NULL, 0, Red); //Open a sell order if(ticket > 0) Alert("The order number is: ", string(ticket));
- You would know why, if you Check your return codes for errors, and report them including GLE/LE. Don't look at it unless you have an error.
Don't just silence the compiler, it is trying to help you.
What are Function return values ? How do I use them ? - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles - Don't use NULL.
- You can use NULL in place of _Symbol only in those calls that the documentation specially says you can. iHigh does, iCustom does, MarketInfo does not. OrderSend does not.
- Don't use NULL (except for pointers where you explicitly check for it.) Use _Symbol and _Period, that is minimalist as possible and more efficient.
- Zero is the same as PERIOD_CURRENT which means _Period. Don't hard code numbers.
- MT4: No need for a function call with iHigh(NULL,0,s) just use the predefined arrays, i.e. High[].
- You are also assuming that there will be a tick in the last 10 seconds. Code fails if there isn't. Use a timer and check there.
- What is the difference between opening in the last 10 seconds, vs waiting for the start of a new bar? Why complicate your code?
- You originally said 10 minutes, not 10 seconds.
Thanks. I added the code above to my EA, but when I BT it, there are no trades. I do get trades when the time requirement is removed. Not sure why this is happening. Can someone take a look? I know I have unmatched data errors. Still looking into solving that.
#property copyright "Test" #property link "https://www.mql5.com" #property version "1.00" #property strict extern int Lb = 10; //input parameter for the indicator int ticket = 0; bool result = false; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { datetime nextBar = Time [0] + PeriodSeconds (); //Time[0] is the open time of the defined bar in seconds. //add 86400 secs for a day from PeriodSeconds //as markets are open 24 hours. If you use a //different time frame it will be different seconds. //Then you get end of day time with nextBar datetime closeMinus10secs = nextBar - (60*1200); Print ("Current Time: ", TimeCurrent()); Print ("closeMinus10secs: ", closeMinus10secs); if (TimeCurrent() >= closeMinus10secs) //Check to see if is the last "X Time" before close and if there is an existing order. OrdersTotal() == 0 TimeCurrent() >= closeMinus10secs { result = True; Print ("Time Req Met? ", result); double v1 = iCustom(NULL,PERIOD_CURRENT,"SSL_Channel_Chart",Lb,0,0); double v2 = iCustom(NULL,PERIOD_CURRENT,"SSL_Channel_Chart",Lb,1,0); double barv1 = iCustom(NULL,PERIOD_CURRENT,"SSL_Channel_Chart",Lb,0,1); double barv2 = iCustom(NULL,PERIOD_CURRENT,"SSL_Channel_Chart",Lb,1,1); if(v2 > v1 && barv2 <= barv1) // set buy criteria from indicator { ticket = OrderSend(Symbol(), OP_BUY, 1.0, Ask, PERIOD_CURRENT, Ask - (iATR(NULL, 0, 14, 0)), Ask + (iATR(NULL, 0, 14,0)), NULL, 0, Green); // Open a buy order if(ticket > 0) Print("The ticket number is: ", string(ticket)); else Print ("Order Send failed, error # ", GetLastError()); } if (v2 < v1 && barv2 >= barv1) // set sell criteria from indicator { ticket = OrderSend (Symbol(), OP_SELL, 1.0, Bid, PERIOD_CURRENT, Bid + (iATR(NULL, 0, 14,0)), Bid - (iATR(NULL, 0, 14, 0)) , NULL, 0, Red); //Open a sell order if(ticket > 0) Print("The ticket number is: ", string(ticket)); else Print ("Order Send failed, error # ", GetLastError()); } } } //+------------------------------------------------------------------+
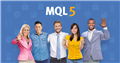
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use