i =Bars-counted_bars-1
You should also have the calculation outside the loop, maybe:
int counted_bars=MathMin(Bars-IndicatorCounted(),Bars-1); for(int i=counted_bars; i>=0; i--)
Frequency changes with timeframe because it's based on timeframe index, not time itself.
If you are going to draw more complicated things (you should, sine waves solely by themselves are stupid) you may want to build a template with this:
https://www.mql5.com/en/docs/standardlibrary/canvasgraphics/ccanvas
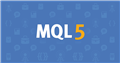
- www.mql5.com
You've updated from init/start to the new event handlers.
Now update from IndicatorCounted to the values in OnCalculate.
How to do
your lookbacks correctly.
Thanks for the interest. I would just like to create sinusoidal waves that do not change frequency based on the timeframe. If I change the timeframe the sine wave must remain the same. I have not found in CCanvas the possibility of creating sonusoidal waves. Could someone give me some examples?
Hi guys, I could calculate my sine wave. Now I should graph it. I would like the wave to be drawn only once at boot time. Is it better to create an EA, an indicator or a script? Which Event Handling Functions should I use?
Thank you.int start()
{
double radianti;
int gradi;
double y;
for (gradi=0;gradi<=360;gradi++)
{
radianti=gradi*(M_PI/180.0);
y=MathArcsin(radianti);
bool grafico=ObjectCreate(0,"seno",OBJ_ARROW,0,radianti,y);
ObjectSetInteger(0,"seno",OBJPROP_ARROWCODE,158);
}
return(0);
}
I can not get what I want. I would like an indicator where you can insert sine waves and then add them with the possibility to change phase and amplitude frequency.
If you specify a sine wave in timeframe H1 if I move in m5 the sine wave must not change frequency. Am I willing to pay for this how much could it cost me?
Thank you
Try maybe by storing data value into a text file and when you change TF, try recreate the graph using the stored values from the text file, that can help you solve your problem.
hello
did you get your sine wave indicator

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use