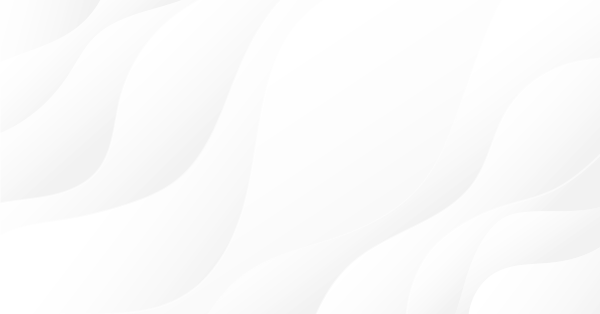
Guia prático MQL5: Desenvolvimento de um Indicador de Símbolos Múltiplos para Análise de Divergência de Preço
Introdução
Neste artigo, vamos considerar o desenvolvimento de um indicador de símbolos múltiplos para análise de divergência de preço dentro de um período de tempo determinado. Os temas centrais já foram discutidas no artigo anterior sobre programação de indicadores de múltiplas moedas: "Guia prático do MQL5: Desenvolvimento de um Indicador de Símbolos Múltiplos em MQL5". Então, desta vez vamos focar apenas nas novas características e funções que foram alteradas drasticamente. Se você é novo em programação de indicadores de múltiplas moedas, primeiro eu recomendo a leitura do artigo anterior.
Neste artigo vamos considerar as seguintes questões:
- Alterar propriedades do gráfico.
- Manusear os eventos CHARTEVENT_OBJECT_DRAG (arrastando um objeto gráfico) e CHARTEVENT_CHART_CHANGE (redimensionar gráfico ou modificar as propriedades de gráfico usando as propriedades da janela de diálogo).
- Renderizar buffers do Indicador usando mais de uma cor.
- Definir máximas e mínimas em buffers do indicador dentro da área de visibilidade para definir um gráfico de máxima/mínima (high/low).
- Inversão de uma série.
O valor resultante de código para o nosso indicador é bastante grande, cerca de 1500 linhas. Portanto, vamos distribuir todas as funções em arquivos separados e ligá-los ao arquivo de projeto principal. Haverá três categorias de funções para os arquivos externos:
- Checks.mqh - Funções para executar várias verificações e para fazer o download dos dados disponíveis.
- Objects.mqh - Funções para gerenciamento de objetos gráficos.
- Chart.mqh - Funções de gestão de propriedades do gráfico.
Todas as funções que não pertencem às categorias acima serão deixadas no arquivo principal.
Desenvolvimento do Indicador
Próximo passo é a programação do indicador. Primeiro precisamos criar um novo projeto. Para isto, abrir uma pasta com o nome do nosso indicador no seguinte caminho de diretório: Metatrader 5\MQL5\Indicators. Na pasta Include vamos colocar os arquivos de inclusão. Apos este procedimento, vamos criar o arquivo principal na pasta do indicador. Isto pode ser realizado manualmente escrevendo um arquivo de texto com a extensão *.mq5 ou usando um modelo do Assistente MQL5. Além das funções básicas do programa OnInit(), OnDeinit() e OnCalculate(), também usamos a OnChartEvent() e OnTimer().
Assim como no artigo anterior, além do símbolo (ativo) corrente, nos exibiremos dados para 5 símbolos determinados nos parâmetros externos. Mas desta vez, em vez de valores calculados através de uma fórmula, a saída de dados será pelos preços brutos sobre o gráfico. Usuário é livre para escolher o tipo de representação de dados nos parâmetros externos a partir da lista do menu drop-down: Linha, Barras ou Candles.
Se quisermos apenas exibir dados como linhas de uma cor, então será o suficiente para determinar o número de buffers igual ao número de símbolos nas propriedades do indicador (#property). Mas uma vez que existem dois modos para desenhar série como barras e candles precisamos de mais buffers para o modo de duas cores: quatro buffers para renderizar cada série e um buffer para definir a cor (dependendo da condição) para cada elemento numa série gráfica.
Para cada série é necessário especificar as cores na seção propriedades do programa. Para fazer isso, basta listá-los separados por vírgulas. Em primeiro lugar utilizar o modo uma cor. O modo duas cores é usado para obter as barras/candles. A segunda cor será usada apenas no modo de duas cores para as barras/candles de baixa.
Os códigos de todos estes parâmetros estão definidos abaixo:
#property indicator_chart_window // Indicator is in the main window #property indicator_buffers 25 // Number of buffers for indicator calculation #property indicator_plots 5 // Number of plotting series //--- Indicator buffers colors #property indicator_color1 clrDodgerBlue,C'0,50,100' #property indicator_color2 clrLimeGreen,C'20,80,20' #property indicator_color3 clrGold,C'160,140,0' #property indicator_color4 clrAqua,C'0,140,140' #property indicator_color5 clrMagenta,C'130,0,130'
Com a diretiva #define vamos declarar as contantes e usar a linha de comando #include, vamos incluir arquivos com funções que já foram descritas acima e uma classe da biblioteca padrão para trabalhar com "canvas":
//--- Constants #define RESET 0 // Returning the indicator recalculation command to the terminal #define SYMBOLS_COUNT 5 // Number of symbols //--- Include the class for working with the canvas #include <Canvas\Canvas.mqh> //--- Include the class for working with the canvas #include "Include/Checks.mqh" #include "Include/Chart.mqh" #include "Include/Objects.mqh"
Adicionar enumeradores ENUM_DRAWTYPE e ENUM_START_POINT para criar menus drop-down que permitirão a seleção do tipo de desenho dos dados dos preços e modo de ponto de partida das divergências dos preços nos parâmetros externos:
//--- Drawing type of the price data enum ENUM_DRAWTYPE { LINE =0, // Line BARS =1, // Bars CANDLES=2 // Candlesticks }; //--- Mode of the price divergence starting point enum ENUM_START_POINT { VERTICAL_LINE=0, // Vertical line MONTH =1, // Month WEEK =2, // Week DAY =3, // Day HOUR =4 // Hour };
Os tipos de renderização de dados já estão descritos acima, agora vamos falar um pouco mais sobre o que é o modo de ponto de partida das divergências dos preços.
Ao todo serão cinco modos: Linha vertical, Mês, Semana, Dia e Hora. O modo Linha Vertical será adicionado ao carregar o indicador no gráfico. Arrastando esta linha na barra determinada, os preços de todos os símbolos vão se reunir num único ponto. O preço de abertura da barra especificada para o símbolo corrente será considerada como um ponto de referência desta reunião. É o modo de se dizer ao programa que os preços devem encontrar-se no início do período determinado. Ou seja, no início de cada mês, no começo de cada semana, no início de cada dia ou no início de cada hora.
Abaixo você pode encontrar a lista dos parâmetros de entrada do indicador:
//--- External parameters input ENUM_DRAWTYPE DrawType =CANDLES; // Drawing type input ENUM_START_POINT StartPriceDivergence =VERTICAL_LINE; // Start of price divergence input bool TwoColor =false; // Two-color bars/candlesticks sinput string dlm01=""; //- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - input string Symbol02 ="GBPUSD"; // Symbol 2 input bool Inverse02 =false; // Inverse symbol 2 input string Symbol03 ="AUDUSD"; // Symbol 3 input bool Inverse03 =false; // Inverse symbol 3 input string Symbol04 ="NZDUSD"; // Symbol 4 input bool Inverse04 =false; // Inverse symbol 4 input string Symbol05 ="USDCAD"; // Symbol 5 input bool Inverse05 =false; // Inverse symbol 5 input string Symbol06 ="USDCHF"; // Symbol 6 input bool Inverse06 =false; // Inverse symbol 6
Símbolos são numerados a partir de 2, uma vez que 1 é o símbolo vigente na tabela.
Inversão pode ser aplicada para cada símbolo incluído. Inversão significa que os dados do símbolo serão processados de cabeça para baixo. Isso pode ser útil quando a lista dos símbolos analisados inclui pares em que uma mesma moeda (por exemplo, dólar dos EUA) pode ser ao mesmo tempo a base de uma e a contraparte de outra. Por exemplo, no par de moedas EURUSD, o dólar é a contraparte do ativo, e no par USDCHF é a base do ativo. Se o símbolo atual no gráfico é EURUSD, então você pode ativar a inversão para USDCHF, o que fará a representação dos preços mais conveniente para a análise.
Abaixo está a lista das variáveis globais e arrays:
//--- Structure of the indicator buffers arrays struct buffers { double open[]; // Open prices buffer double high[]; // High prices buffer double low[]; // Low prices buffer double close[]; // Close prices buffer double icolor[]; // Buffer to determine the color of element }; buffers buffer_data[SYMBOLS_COUNT]; //--- Load the class CCanvas canvas; //--- Variables/arrays for copying data from OnCalculate() int OC_rates_total =0; // Size of input time series int OC_prev_calculated =0; // Bars processed at the previous call datetime OC_time[]; // Opening time double OC_open[]; // Open prices double OC_high[]; // High prices double OC_low[]; // Low prices double OC_close[]; // Close prices long OC_tick_volume[]; // Tick volumes long OC_volume[]; // Real volumes int OC_spread[]; // Spread //--- For the purpose of storing and checking the time of the first bar in the terminal datetime series_first_date[SYMBOLS_COUNT]; datetime series_first_date_last[SYMBOLS_COUNT]; //--- Time array of the bar from which we will start drawing datetime limit_time[SYMBOLS_COUNT]; //--- Symbol names array string symbol_names[SYMBOLS_COUNT]; //--- Array of symbol inverse flags bool inverse[SYMBOLS_COUNT]; //--- Colors of indicator lines color line_colors[SYMBOLS_COUNT]={clrDodgerBlue,clrLimeGreen,clrGold,clrAqua,clrMagenta}; //--- String representing the lack of the symbol string empty_symbol="EMPTY"; //--- Chart properties int window_number =WRONG_VALUE; // Indicator window number int chart_width =0; // Chart width int chart_height =0; // Chart height int last_chart_width =0; // Last saved chart width int last_chart_height =0; // Last saved chart height int chart_center_x =0; // Horizontal center of chart int chart_center_y =0; // Vertical center of chart color color_bar_up =clrRed; // Up bar color color color_bar_down =C'100,0,0'; // Down bar color string indicator_shortname ="MS_PriceDivergence"; // Short name of the indicator string prefix =indicator_shortname+"_"; // Prefix for objects //--- Name of vertical line of the price divergence starting point string start_price_divergence=prefix+"start_price_divergence"; //--- Canvas properties string canvas_name =prefix+"canvas"; // Canvas name color canvas_background =clrBlack; // Canvas background color uchar canvas_opacity =190; // Opacity int font_size =16; // Font size string font_name ="Calibri"; // Font ENUM_COLOR_FORMAT clr_format =COLOR_FORMAT_ARGB_RAW; // Color components should be correctly set by the user //--- Canvas messages string msg_prepare_data ="Preparing data! Please wait..."; string msg_not_synchronized ="Unsynchronized data! Please wait..."; string msg_load_data =""; string msg_sync_update =""; string msg_last =""; //--- ENUM_TIMEFRAMES timeframe_start_point =Period(); // Timeframe for the price divergence starting point datetime first_period_time =NULL; // Time of the first specified period on chart double divergence_price =0.0; // Price of the price divergence starting point datetime divergence_time =NULL; // Time of the price divergence starting point double symbol_difference[SYMBOLS_COUNT]; // Difference in price relative to the current symbol double inverse_difference[SYMBOLS_COUNT]; // Difference that is formed when calculating inversion
A seguir, vamos considerar as funções que são usados durante a inicialização do indicador. Em geral, não há grandes mudanças em comparação com a função OnInit() do artigo anterior.
Vamos adicionar a verificação de onde o indicador é utilizado. O fato é que atualmente os desenvolvedores do terminal não implementaram todas as características de controle das propriedades do gráfico no Testador de Estratégia, então nós restringimos nosso indicador a ser usado apenas fora de Testador de Estratégia. Para implementar isso, vamos escrever uma função simples - CheckTesterMode(). Ela será localizada no arquivo Checks.mqh :
//+------------------------------------------------------------------+ //| Checks if indicator is used in Strategy Tester | //+------------------------------------------------------------------+ bool CheckTesterMode() { //--- Report that indicator is not intended to be used in Strategy Tester if(MQLInfoInteger(MQL_TESTER) || MQLInfoInteger(MQL_VISUAL_MODE) || MQLInfoInteger(MQL_OPTIMIZATION)) { Comment("Currently, the <- "+MQLInfoString(MQL_PROGRAM_NAME)+" -> indicator is not intended to be used in Strategy Tester!"); return(false); } //--- return(true); }
Outra nova função SetBarsColors() pretende definir as cores para barras/candles do símbolo vigente. Ela está localizada no arquivo Chart.mqh.
//+------------------------------------------------------------------+ //| Sets colors for the current symbol bars | //+------------------------------------------------------------------+ void SetBarsColors() { //--- Color for the up bar, shadows and body borders of bull candlesticks ChartSetInteger(0,CHART_COLOR_CHART_UP,color_bar_up); //--- Body color of a bull candlestick ChartSetInteger(0,CHART_COLOR_CANDLE_BULL,color_bar_up); //--- Line chart color and color of "Doji" Japanese candlesticks ChartSetInteger(0,CHART_COLOR_CHART_LINE,color_bar_up); //--- For two-color mode if(TwoColor) { //--- Color for the down bar, shadows and body borders of bear candlesticks ChartSetInteger(0,CHART_COLOR_CHART_DOWN,color_bar_down); //--- Body color of a bear candlestick ChartSetInteger(0,CHART_COLOR_CANDLE_BEAR,color_bar_down); } //--- If two-color mode is turned off else { //--- Color for the down bar, shadows and body borders of bear candlesticks ChartSetInteger(0,CHART_COLOR_CHART_DOWN,color_bar_up); //--- Body color of a bear candlestick ChartSetInteger(0,CHART_COLOR_CANDLE_BEAR,color_bar_up); } }
Durante a inicialização é preciso determinar o modo que será selecionado o parâmetro externo StartPriceDivergence. Se a linha vertical for selecionado, então a variável global timeframe_start_point será atribuída com o valor padrão, ou seja, o timeframe atual. Caso contrário o timeframe selecionado será aplicado. Para isso vamos escrever a função InitStartPointTF():
//+------------------------------------------------------------------+ //| Identifies timeframe for the price starting point mode | //+------------------------------------------------------------------+ void InitStartPointTF() { //--- Exit if vertical line mode is selected if(StartPriceDivergence==VERTICAL_LINE) return; //--- Otherwise define the timeframe switch(StartPriceDivergence) { case MONTH : timeframe_start_point=PERIOD_MN1; break; case WEEK : timeframe_start_point=PERIOD_W1; break; case DAY : timeframe_start_point=PERIOD_D1; break; case HOUR : timeframe_start_point=PERIOD_H1; break; } }
A função CheckInputParameters() diferentemente do artigo anterior agora se parece com isso:
//+------------------------------------------------------------------+ //| Checks input parameters for correctness | //+------------------------------------------------------------------+ bool CheckInputParameters() { //--- For all other modes except the 'Vertical Line' if(StartPriceDivergence!=VERTICAL_LINE) { //--- If the current period is greater than or equal to the specified period of the price divergence starting point, report of it and exit if(PeriodSeconds()>=PeriodSeconds(timeframe_start_point)) { Print("Current timeframe should be less than one specified in the Start Price Divergence parameter!"); Comment("Current timeframe should be less than one specified in the Start Price Divergence parameter!"); return(false); } } //--- return(true); }
Arrays são inicializados como no artigo anterior. Apenas os nomes e o número das arrays foram alteradas.
//+------------------------------------------------------------------+ //| First initialization of arrays | //+------------------------------------------------------------------+ void InitArrays() { ArrayInitialize(limit_time,NULL); ArrayInitialize(symbol_difference,0.0); ArrayInitialize(inverse_difference,0.0); ArrayInitialize(series_first_date,NULL); ArrayInitialize(series_first_date_last,NULL); //--- for(int s=0; s<SYMBOLS_COUNT; s++) { ArrayInitialize(buffer_data[s].open,EMPTY_VALUE); ArrayInitialize(buffer_data[s].high,EMPTY_VALUE); ArrayInitialize(buffer_data[s].low,EMPTY_VALUE); ArrayInitialize(buffer_data[s].close,EMPTY_VALUE); ArrayInitialize(buffer_data[s].icolor,EMPTY_VALUE); } } //+------------------------------------------------------------------+ //| Initializes array of symbols | //+------------------------------------------------------------------+ void InitSymbolNames() { symbol_names[0]=AddSymbolToMarketWatch(Symbol02); symbol_names[1]=AddSymbolToMarketWatch(Symbol03); symbol_names[2]=AddSymbolToMarketWatch(Symbol04); symbol_names[3]=AddSymbolToMarketWatch(Symbol05); symbol_names[4]=AddSymbolToMarketWatch(Symbol06); } //+------------------------------------------------------------------+ //| Initializes array of inversions | //+------------------------------------------------------------------+ void InitInverse() { inverse[0]=Inverse02; inverse[1]=Inverse03; inverse[2]=Inverse04; inverse[3]=Inverse05; inverse[4]=Inverse06; }
Alterações significativas foram feitas para a função SetIndicatorProperties(). Na verdade é uma nova função. Agora, dependendo do modo de como a renderização de dados é selecionada, as propriedades correspondentes são definidas durante a inicialização.
//+------------------------------------------------------------------+ //| Sets indicator properties | //+------------------------------------------------------------------+ void SetIndicatorProperties() { //--- Set the short name IndicatorSetString(INDICATOR_SHORTNAME,indicator_shortname); //--- Set the number of decimal digits IndicatorSetInteger(INDICATOR_DIGITS,_Digits); //--- In the 'Line' mode we need only one buffers that displays the open price if(DrawType==LINE) { for(int s=0; s<SYMBOLS_COUNT; s++) SetIndexBuffer(s,buffer_data[s].close,INDICATOR_DATA); } //--- In other modes we use all prices for drawing // bars/candlesticks and additional buffer for the two-color mode else if(DrawType==BARS || DrawType==CANDLES) { for(int s=0; s<SYMBOLS_COUNT; s++) { static int buffer_number=0; SetIndexBuffer(buffer_number,buffer_data[s].open,INDICATOR_DATA); buffer_number++; SetIndexBuffer(buffer_number,buffer_data[s].high,INDICATOR_DATA); buffer_number++; SetIndexBuffer(buffer_number,buffer_data[s].low,INDICATOR_DATA); buffer_number++; SetIndexBuffer(buffer_number,buffer_data[s].close,INDICATOR_DATA); buffer_number++; SetIndexBuffer(buffer_number,buffer_data[s].icolor,INDICATOR_COLOR_INDEX); buffer_number++; } } //--- Set labels for the current timeframe // In the 'Line' mode only opening price is used if(DrawType==LINE) { for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetString(s,PLOT_LABEL,symbol_names[s]+",Close"); } //--- In other modes all prices of bars/candlesticks // ";" is used as a separator else if(DrawType==BARS || DrawType==CANDLES) { for(int s=0; s<SYMBOLS_COUNT; s++) { PlotIndexSetString(s,PLOT_LABEL, symbol_names[s]+",Open;"+ symbol_names[s]+",High;"+ symbol_names[s]+",Low;"+ symbol_names[s]+",Close"); } } //--- Set the type of lines for indicator buffers //--- Line if(DrawType==LINE) for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetInteger(s,PLOT_DRAW_TYPE,DRAW_LINE); //--- Bars if(DrawType==BARS) for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetInteger(s,PLOT_DRAW_TYPE,DRAW_COLOR_BARS); //--- Candlesticks if(DrawType==CANDLES) for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetInteger(s,PLOT_DRAW_TYPE,DRAW_COLOR_CANDLES); //--- Set the type of lines for data of current symbol //--- Line if(DrawType==LINE) ChartSetInteger(0,CHART_MODE,CHART_LINE); //--- Bars if(DrawType==BARS) ChartSetInteger(0,CHART_MODE,CHART_BARS); //--- Candlesticks if(DrawType==CANDLES) ChartSetInteger(0,CHART_MODE,CHART_CANDLES); //--- Set the line width for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetInteger(s,PLOT_LINE_WIDTH,1); //--- Set the line color for the 'Line' mode if(DrawType==LINE) for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetInteger(s,PLOT_LINE_COLOR,line_colors[s]); //--- Display data in Data Window only for existing symbols for(int s=0; s<SYMBOLS_COUNT; s++) { if(symbol_names[s]!=empty_symbol) PlotIndexSetInteger(s,PLOT_SHOW_DATA,true); else PlotIndexSetInteger(s,PLOT_SHOW_DATA,false); } //--- Empty value for plotting where nothing will be drawn for(int s=0; s<SYMBOLS_COUNT; s++) PlotIndexSetDouble(s,PLOT_EMPTY_VALUE,EMPTY_VALUE); }
E finalmente, uma outra nova função, a SetDivergenceLine(), para ser usada em OnInit(). Ele define a linha vertical verde para manipular o ponto de partida de divergência dos preços no modo linha Vertical.
//+------------------------------------------------------------------+ //| Sets vertical line for price divergence starting point | //+------------------------------------------------------------------+ void SetDivergenceLine() { //--- If there is no vertical line yet, set it if(StartPriceDivergence==VERTICAL_LINE && ObjectFind(0,start_price_divergence)<0) //--- Place a vertical line on the true bar CreateVerticalLine(0,0,TimeCurrent()+PeriodSeconds(),start_price_divergence, 2,STYLE_SOLID,clrGreenYellow,true,true,false,"","\n"); //--- For all other modes except the 'Vertical Line' if(StartPriceDivergence!=VERTICAL_LINE) DeleteObjectByName(start_price_divergence); }
Abaixo está a representação de toda a descrição acima dentro da função OnInit(). Quando tudo é dividido em arquivos e funções separadas se torna muito mais conveniente para ler o código do programa.
//+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Check if indicator is currently being used in Strategy Tester if(!CheckTesterMode()) return(INIT_FAILED); //--- Set the color for bars/candlesticks SetBarsColors(); //--- Define the timeframe for the price divergence starting point InitStartPointTF(); //--- Check input parameters for correctness if(!CheckInputParameters()) return(INIT_PARAMETERS_INCORRECT); //--- Set the timer at 1-second intervals EventSetMillisecondTimer(1000); //--- Set the font to be displayed on the canvas canvas.FontSet(font_name,font_size,FW_NORMAL); //--- Initialization of arrays InitArrays(); //--- Initialize the array of symbols InitSymbolNames(); //--- Initialize the array of inversions InitInverse(); //--- Set indicator properties SetIndicatorProperties(); //--- Set vertical line of the price divergence start SetDivergenceLine(); //--- Clear the comment Comment(""); //--- Refresh the chart ChartRedraw(); //--- Initialization completed successfully return(INIT_SUCCEEDED); }
Na função OnCalculate(), o código do programa manteve-se praticamente inalterado. No artigo anterior, depois de todas as verificações sobre a disponibilidade dos dados terem sido realizados, o programa preencheu primeiro as arrays auxiliares e só então preencheu os buffers do indicador com os dados preparado. Desta vez, vamos tentar organizar tudo num único loop.
Fiz funções de validação e carregamento de dados mais rigorosos. Agora, cada valor que você deseja obter passa por determinado número de tentativas. Se o valor é obtido, o loop é interrompido. E já que agora temos os modos necessários para determinar o início de um período (mês, semana, dia, hora), então vamos obter o tempo de início do período através de um timeframe superior. Por isso eu criei uma função adicional semelhante a LoadAndFormData() que também é similar no nome, LoadAndFormDataHighTF(). Seu código é muito semelhante ao original, por isso não vou postá-lo aqui.
Verificação da disponibilidade de dados para os timeframes atuais e superiores foi implantado na função CheckAvailableData():
//+------------------------------------------------------------------+ //| Checks the amount of available data for all symbols | //+------------------------------------------------------------------+ bool CheckAvailableData() { int attempts=100; //--- for(int s=0; s<SYMBOLS_COUNT; s++) { //--- If this symbol is available if(symbol_names[s]!=empty_symbol) { datetime time[]; // Array for checking the number of bars int total_period_bars =0; // Number of bars of the current period datetime terminal_first_date =NULL; // First date of the current time frame data available in the terminal //--- Get the first date of the current time frame data in the terminal terminal_first_date=(datetime)SeriesInfoInteger(symbol_names[s],Period(),SERIES_TERMINAL_FIRSTDATE); //--- Get the number of available bars from the date specified total_period_bars=Bars(symbol_names[s],Period(),terminal_first_date,TimeCurrent()); //--- Check the readiness of bar data for(int i=0; i<attempts; i++) { //--- Copy the specified amount of data if(CopyTime(symbol_names[s],Period(),0,total_period_bars,time)) { //--- If the required amount has been copied, terminate the loop if(ArraySize(time)>=total_period_bars) break; } } //--- If the amount of data copied is not sufficient, one more attempt is required if(ArraySize(time)==0 || ArraySize(time)<total_period_bars) { msg_last=msg_prepare_data; ShowCanvasMessage(msg_prepare_data); OC_prev_calculated=0; return(false); } } } //--- Exit if current mode is vertical line of the price divergence starting point if(StartPriceDivergence==VERTICAL_LINE) return(true); else { datetime time[]; // Array for checking the number of bars int total_period_bars =0; // Number of bars of the current period datetime terminal_first_date =NULL; // First date of the current time frame data available in the terminal //--- Get the first date of the current time frame data in the terminal for(int i=0; i<attempts; i++) if((terminal_first_date=(datetime)SeriesInfoInteger(Symbol(),Period(),SERIES_FIRSTDATE))>0) break; //--- Get the number of available bars from the date specified for(int i=0; i<attempts; i++) if((total_period_bars=(int)SeriesInfoInteger(Symbol(),timeframe_start_point,SERIES_BARS_COUNT))>0) break; //--- Check the readiness of bar data for(int i=0; i<attempts; i++) //--- Copy the specified amount of data if(CopyTime(Symbol(),timeframe_start_point, terminal_first_date+PeriodSeconds(timeframe_start_point),TimeCurrent(),time)>0) break; //--- If the amount of data copied is not sufficient, one more attempt is required if(ArraySize(time)<=0 || total_period_bars<=0) { msg_last=msg_prepare_data; ShowCanvasMessage(msg_prepare_data); OC_prev_calculated=0; return(false); } } //--- return(true); }
A função FillIndicatorBuffers() foi significativamente complicada para a tarefa atual. Isto se deve ao fato de que agora existem vários modos e cada um deles requer suas próprias ações. Na verdade, tudo pode ser dividido em quatro etapas:
- Obter os dados do símbolo determinado.
- Obter os dados do timeframe maior, determinar o tempo e o nível de preços onde os preços de todos os símbolos são reunidos.
- Calcular os valores e preencher o buffer do indicador.
- Verificação de cálculos dos valores.
O código da função é fornecido com comentários detalhados para sua consideração:
//+------------------------------------------------------------------+ //| Fills indicator buffers | //+------------------------------------------------------------------+ void FillIndicatorBuffers(int i,int s,datetime const &time[]) { MqlRates rates[]; // Data structure double period_open[]; // Opening price for bar at the price divergence starting point datetime period_time[]; // Time of the price divergence starting point int attempts=100; // Number of copying attempts datetime high_tf_time=NULL; // Time of higher timeframe's bar //--- Exit if we are out of "true" bars zone if(time[i]<limit_time[s]) return; //--- Reset the last error ResetLastError(); //--- Get data of current bar for the specified symbol for(int j=0; j<attempts; j++) if(CopyRates(symbol_names[s],Period(),time[i],1,rates)==1) { ResetLastError(); break; } //--- Exit if failed to get data if(ArraySize(rates)<1 || GetLastError()!=0) return; //--- If the current time is before the first timeframe's time or // bar time is not equal to the bar time of the current symbol or // empty values are fetched if(rates[0].time==NULL || time[i]!=rates[0].time || time[i]<first_period_time || rates[0].low==EMPTY_VALUE || rates[0].open==EMPTY_VALUE || rates[0].high==EMPTY_VALUE || rates[0].close==EMPTY_VALUE) { //--- Write empty value if(DrawType!=LINE) { buffer_data[s].low[i] =EMPTY_VALUE; buffer_data[s].open[i] =EMPTY_VALUE; buffer_data[s].high[i] =EMPTY_VALUE; } buffer_data[s].close[i]=EMPTY_VALUE; return; } //--- If current mode is vertical line of the price divergence starting point if(StartPriceDivergence==VERTICAL_LINE) { //--- Get the time of the line divergence_time=(datetime)ObjectGetInteger(0,start_price_divergence,OBJPROP_TIME); //--- Get the time of the first bar first_period_time=time[0]; } //--- For all other modes, we will keep track the beginning of period else { //--- If we are here for the first time, store data of the first bar of higher timeframe if(divergence_time==NULL) { ResetLastError(); //--- Get opening time of the first bar of higher timeframe for(int j=0; j<attempts; j++) if(CopyTime(Symbol(),timeframe_start_point,time[0]+PeriodSeconds(timeframe_start_point),1,period_time)==1) { ResetLastError(); break; } //--- Exit if failed to get price/time if(ArraySize(period_time)<1 || GetLastError()!=0) return; //--- Otherwise store time of the first bar of higher timeframe else first_period_time=period_time[0]; } //--- If current bar's time on the current timeframe is before the first bar's time on higher timeframe if(time[i]<first_period_time) high_tf_time=first_period_time; //--- Otherwise we will receive data of the last bar of the higher timeframe with respect to the current bar on the current timeframe else high_tf_time=time[i]; //--- Reset the last error ResetLastError(); //--- Get the opening price of the first bar of the higher timeframe for(int j=0; j<attempts; j++) if(CopyOpen(Symbol(),timeframe_start_point,high_tf_time,1,period_open)==1) { ResetLastError(); break; } //--- Get opening time of the first bar of higher timeframe for(int j=0; j<attempts; j++) if(CopyTime(Symbol(),timeframe_start_point,high_tf_time,1,period_time)==1) { ResetLastError(); break; } //--- Exit if failed to get price/time if(ArraySize(period_open)<1 || ArraySize(period_time)<1 || GetLastError()!=0) return; //--- If the current timeframe's time is before the first period's time or // time of specified period is not equal to the one in memory if(time[i]<first_period_time || divergence_time!=period_time[0]) { symbol_difference[s] =0.0; // Zero out difference in symbol prices inverse_difference[s] =0.0; // Zero our difference of inversion //--- Store time of the price divergence starting point divergence_time=period_time[0]; //--- Store price of the price divergence starting point divergence_price=period_open[0]; //--- Set vertical line in the beginning of the price divergence start CreateVerticalLine(0,0,period_time[0],start_price_divergence+"_"+TimeToString(divergence_time), 2,STYLE_SOLID,clrWhite,false,false,true,TimeToString(divergence_time),"\n"); } } //--- If current mode is 'Vertical Line' and bar's time is less than line's time if(StartPriceDivergence==VERTICAL_LINE && time[i]<divergence_time) { //--- Keep zero values of difference symbol_difference[s] =0.0; inverse_difference[s] =0.0; //--- For the 'Line' drawing mode only opening price is used if(DrawType==LINE) buffer_data[s].close[i]=rates[0].close-symbol_difference[s]; //--- For all other modes all prices are used else { buffer_data[s].low[i] =rates[0].low-symbol_difference[s]; buffer_data[s].open[i] =rates[0].open-symbol_difference[s]; buffer_data[s].high[i] =rates[0].high-symbol_difference[s]; buffer_data[s].close[i] =rates[0].close-symbol_difference[s]; //--- Set color for the current element of indicator buffer SetBufferColorIndex(i,s,rates[0].close,rates[0].open); } } //--- For all other modes else { //--- If inversion of symbol data is required if(inverse[s]) { //--- If new period has started, recalculate variables if(symbol_difference[s]==0.0) { //--- For the 'Vertical Line' mode if(StartPriceDivergence==VERTICAL_LINE) { //--- Calculate the difference symbol_difference[s] =rates[0].open-OC_open[i]; inverse_difference[s] =OC_open[i]-(-OC_open[i]); } //--- For all other modes else { //--- Calculate the difference symbol_difference[s] =rates[0].open-divergence_price; inverse_difference[s] =divergence_price-(-divergence_price); } } //--- In the 'Line' mode only opening price is used if(DrawType==LINE) buffer_data[s].close[i]=-(rates[0].close-symbol_difference[s])+inverse_difference[s]; //--- For all other modes all prices are used else { buffer_data[s].low[i] =-(rates[0].low-symbol_difference[s])+inverse_difference[s]; buffer_data[s].open[i] =-(rates[0].open-symbol_difference[s])+inverse_difference[s]; buffer_data[s].high[i] =-(rates[0].high-symbol_difference[s])+inverse_difference[s]; buffer_data[s].close[i] =-(rates[0].close-symbol_difference[s])+inverse_difference[s]; //--- Set color for the current element of indicator buffer SetBufferColorIndex(i,s,rates[0].close,rates[0].open); } } //--- If inversion is not used, then we need to calculate only the difference between symbol prices at the beginning of period else { //--- If new period has started if(symbol_difference[s]==0.0) { //--- For the 'Vertical Line' mode if(StartPriceDivergence==VERTICAL_LINE) symbol_difference[s]=rates[0].open-OC_open[i]; //--- For all other modes else symbol_difference[s]=rates[0].open-divergence_price; } //--- For the 'Line' drawing mode only opening price is used if(DrawType==LINE) buffer_data[s].close[i]=rates[0].close-symbol_difference[s]; //--- For all other modes all prices are used else { buffer_data[s].low[i] =rates[0].low-symbol_difference[s]; buffer_data[s].open[i] =rates[0].open-symbol_difference[s]; buffer_data[s].high[i] =rates[0].high-symbol_difference[s]; buffer_data[s].close[i] =rates[0].close-symbol_difference[s]; //--- Set color for the current element of indicator buffer SetBufferColorIndex(i,s,rates[0].close,rates[0].open); } } } //--- Verification of the calculated values // In the 'Line' mode only opening price is used if(DrawType==LINE) { //--- If the current time is before the first timeframe's time or // bar time is not equal to the bar time, write empty value if(time[i]!=rates[0].time || time[i]<first_period_time) buffer_data[s].close[i]=EMPTY_VALUE; } //--- For all other modes all prices are used else { //--- If the current time is before the first timeframe's time or // bar time is not equal to the bar time of the current symbol or // empty values are fetched if(rates[0].time==NULL || time[i]!=rates[0].time || time[i]<first_period_time || rates[0].low==EMPTY_VALUE || rates[0].open==EMPTY_VALUE || rates[0].high==EMPTY_VALUE || rates[0].close==EMPTY_VALUE) { //--- Write empty value buffer_data[s].low[i] =EMPTY_VALUE; buffer_data[s].open[i] =EMPTY_VALUE; buffer_data[s].high[i] =EMPTY_VALUE; buffer_data[s].close[i] =EMPTY_VALUE; } } }
Ao estudar a função acima, você deve notar outra função personalizada, a SetBufferColorIndex(). Esta função define a cor no buffer de cor do indicador.
//+------------------------------------------------------------------+ //| Sets the color for buffer element by condition | //+------------------------------------------------------------------+ void SetBufferColorIndex(int i,int symbol_number,double close,double open) { //--- For two-color mode, check condition if(TwoColor) { //--- If the closing price is more than the opening price, this is up bar, so we use the first color if(close>open) buffer_data[symbol_number].icolor[i]=0; //--- otherwise it is down bar, so we use the second color else buffer_data[symbol_number].icolor[i]=1; } //--- For one-color mode we use the first color for all bars/candlesticks else buffer_data[symbol_number].icolor[i]=0; }
Uma vez que os buffers de indicador estão cheios, precisamos determinar a máxima e a mínima de todos os valores que estão atualmente disponíveis na janela do gráfico. MQL5 permite obter a primeira barra visível numa janela de gráfico e o número de barras visíveis. Vamos nos beneficiar destes recursos em outra função personalizada, a CorrectChartMaxMin(). O fluxo do código na função pode ser dividido em várias etapas:
- Determinar os números da primeira e da última barra visível.
- Determinar a máxima e a mínima das barras visíveis para o símbolo vigente.
- Determinar a máxima e a mínima entre todas as arrays de símbolos.
- Defenir a máxima e a mínima nas propriedades do gráfico.
Abaixo está o código da função CorrectChartMaxMin() localizada no arquivo Chart.mqh.
//+------------------------------------------------------------------+ //| Corrects chart's high/low with respect to all buffers | //+------------------------------------------------------------------+ void CorrectChartMaxMin() { double low[]; // Array of lows double high[]; // Array of highs int attempts =10; // Number of attempts int array_size =0; // Array size for drawing int visible_bars =0; // Number of visible bars int first_visible_bar =0; // Number of the first visible bar int last_visible_bar =0; // Number of the last visible bar double max_price =0.0; // Highest price double min_price =0.0; // Lowest price double offset_max_min =0.0; // Offset from chart's high/low //--- Reset the last error ResetLastError(); //--- Number of visible bars visible_bars=(int)ChartGetInteger(0,CHART_VISIBLE_BARS); //--- Number of the first visible bar first_visible_bar=(int)ChartGetInteger(0,CHART_FIRST_VISIBLE_BAR); //--- Number of the last visible bar last_visible_bar=first_visible_bar-visible_bars; //--- Exit in case of error if(GetLastError()!=0) return; //--- Fix incorrect value if(last_visible_bar<0) last_visible_bar=0; //--- Get the current symbol high/low in visible area of chart for(int i=0; i<attempts; i++) if(CopyHigh(Symbol(),Period(),last_visible_bar,visible_bars,high)==visible_bars) break; for(int i=0; i<attempts; i++) if(CopyLow(Symbol(),Period(),last_visible_bar,visible_bars,low)==visible_bars) break; //--- Exit if failed to get data if(ArraySize(high)<=0 || ArraySize(low)<=0) return; //--- If succeeded to get data, identify high and low in the current symbol arrays else { min_price=low[ArrayMinimum(low)]; max_price=high[ArrayMaximum(high)]; } //--- Get high and low prices in all price arrays for(int s=0; s<SYMBOLS_COUNT; s++) { //--- If current symbol is not present, go to the next one if(symbol_names[s]==empty_symbol) continue; //--- datetime time[]; // Time array int bars_count=0; // Number of bars for calculation //--- Set zero size for arrays ArrayResize(high,0); ArrayResize(low,0); //--- Get the time of the first bar visible on chart for(int i=0; i<attempts; i++) if(CopyTime(Symbol(),Period(),last_visible_bar,visible_bars,time)==visible_bars) break; //--- Exit if the amount of data is less than number of visible bars on chart if(ArraySize(time)<visible_bars) return; //--- If time of the first "true" bar is greater than // time of the first visible bar on the chart, then // get available number of bars of the current symbol in loop if(limit_time[s]>time[0]) { //--- Get the array size array_size=ArraySize(time); //--- Get the number of bars from the first "true" one if((bars_count=Bars(Symbol(),Period(),limit_time[s],time[array_size-1]))<=0) return; } //--- Else get number of visible bars on chart else bars_count=visible_bars; //--- Index elements in indicator buffers as timeseries ArraySetAsSeries(low,true); ArraySetAsSeries(high,true); //--- Copy data from the indicator buffer // All modes except 'Line' if(DrawType!=LINE) { ArrayCopy(low,buffer_data[s].low); ArrayCopy(high,buffer_data[s].high); } //--- For the 'Line' mode else { ArrayCopy(low,buffer_data[s].close); ArrayCopy(high,buffer_data[s].close); } //--- Get the array size array_size=ArraySize(high); //--- Fill empty values, // so they are not considered when calculating high/low for(int i=0; i<array_size; i++) { if(high[i]==EMPTY_VALUE) high[i]=max_price; if(low[i]==EMPTY_VALUE) low[i]=min_price; } //--- Get high/low with respect to inversion if(inverse[s]) { //--- If no errors occur, store values if(ArrayMaximum(high,last_visible_bar,bars_count)>=0 && ArrayMinimum(low,last_visible_bar,bars_count)>=0) { max_price=fmax(max_price,low[ArrayMaximum(low,last_visible_bar,bars_count)]); min_price=fmin(min_price,high[ArrayMinimum(high,last_visible_bar,bars_count)]); } } else { //--- If no errors occur, store values if(ArrayMinimum(low,last_visible_bar,bars_count)>=0 && ArrayMaximum(high,last_visible_bar,bars_count)>=0) { min_price=fmin(min_price,low[ArrayMinimum(low,last_visible_bar,bars_count)]); max_price=fmax(max_price,high[ArrayMaximum(high,last_visible_bar,bars_count)]); } } } //--- Calculate offset (3%) form chart's top and bottom offset_max_min=((max_price-min_price)*3)/100; //--- Turn on the fixed chart scale mode. ChartSetInteger(0,CHART_SCALEFIX,true); //--- Set high/low ChartSetDouble(0,CHART_FIXED_MAX,max_price+offset_max_min); ChartSetDouble(0,CHART_FIXED_MIN,min_price-offset_max_min); //--- Refresh the chart ChartRedraw(); }
A função descrita acima será usada durante o processamento de eventos para arrastar a linha vertical (e para o cálculo dos valores dos indicadores em OnCalculate, é claro):
//+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- Event of dragging a graphical object if(id==CHARTEVENT_OBJECT_DRAG) { //--- If current mode is vertical line for the price divergence starting point, then update indicator buffers if(StartPriceDivergence==VERTICAL_LINE) OnCalculate(OC_rates_total, 0, OC_time, OC_open, OC_high, OC_low, OC_close, OC_tick_volume, OC_volume, OC_spread); } //--- Event of resizing the chart or modifying the chart properties using the properties dialog window. if(id==CHARTEVENT_CHART_CHANGE) //--- Correct the maximum and minimum of chart with respect to the indicator buffers' values CorrectChartMaxMin(); }
Todas as funções estão prontas. Você pode estudar o código totalmente comentado e anexo a este artigo.
Vamos demonstrar o que finalmente conseguimos. Os símbolos (ativos) padrao GBPUSD, AUDUSD, NZDUSD, USDCAD, USDCHF estão determinados nos parâmetros externos. Na imagem abaixo você pode ver o gráfico semanal para o EURUSD no modo Linha Vertical com inversão desativada:
Fig. 1 - Timeframe semanal no modo "linha vertical"
Na imagem abaixo você pode ver o timeframe M30 no modo Diário, onde a inversão de tempo está habilitada para símbolos com o USD como a moeda base. Nesta situação temos os pares USDCAD (candles azul claro) e USDCHF (candles roxo).
Fig. 2 - Time frame M30 no modo "Dia"
Conclusão
Eu acho que nós criamos uma ferramenta muito interessante e informativa para análise de divergência dos preços de moedas múltiplas. Este indicador pode ser infinitamente melhorado.
Obrigado pelo seu tempo!
Traduzido do russo pela MetaQuotes Ltd.
Artigo original: https://www.mql5.com/ru/articles/754





- Aplicativos de negociação gratuitos
- 8 000+ sinais para cópia
- Notícias econômicas para análise dos mercados financeiros
Você concorda com a política do site e com os termos de uso