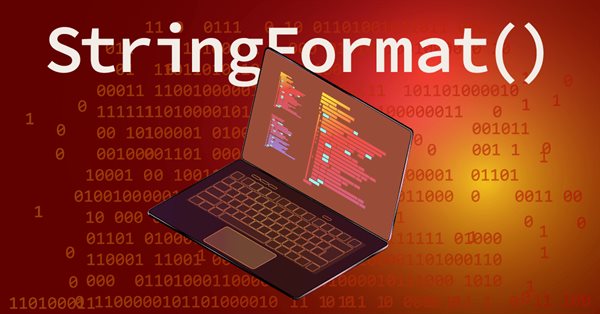
StringFormat(). Visão geral, exemplos de uso prontos
Conteúdo
- Introdução
- StringFormat(). Visão geral concisa
- Formatação de strings
- Saída formatada das propriedades do símbolo
- Obtendo e exibindo as propriedades inteiras do símbolo
- Atraso de dados
- Setor da economia
- Setor da indústria
- Símbolo personalizado
- Cor de fundo na Observação do Mercado
- Preço para construção de barras
- Existência do símbolo
- Selecionado na Observação do Mercado
- Visível na Observação do Mercado
- Número de negociações na sessão atual
- Número de ordens de compra
- Número de ordens de venda
- Volume na última negociação
- Volume máximo no dia
- Volume mínimo no dia
- Horário da última cotação
- Hora da última cotação em milissegundos
- Dígitos
- Spread
- Spread flutuante
- Número de ordens no livro de ofertas
- Modo de cálculo do tamanho do contrato
- Modo de execução das ordens
- Data de início da negociação
- Data de término das negociações
- Nível de Stops
- Nível de Congelamento
- Modo de Execução de Ordens
- Modelo de Cálculo de Swap
- Dia de Rollover de Triplo Swap
- Modo de Cálculo de Margem de Hedge
- Flags dos Modos de Expiração de Ordens
- Flags dos Modos de Preenchimento de Ordens
- Flags dos Tipos de Ordens Permitidos
- Prazo de Validade das Ordens Stop Loss e Take Profit
- Tipo de Opção
- Obtenção e exibição das propriedades de string do símbolo
- Preço de Bid
- Maior Bid do Dia
- Menor Bid do Dia
- Preço de Ask
- Maior Ask do Dia
- Menor Ask do Dia
- Preço de Última Negociação
- Maior Último do Dia
- Menor Último do Dia
- Volume da Última Negociação
- Maior Volume do Dia
- Menor Volume do Dia
- Preço de Execução da Opção
- Ponto
- Valor do Tick de Negociação
- Valor do Tick de Negociação para Posições Lucrativas
- Valor do Tick de Negociação para Posições com perdas
- Menor Alteração de Preço
- Tamanho do Contrato de Negociação
- Juros Acumulados
- Valor Nominal
- Taxa de Liquidez
- Volume Mínimo
- Volume Máximo
- Incremento Mínimo no Volume
- Volume máximo de posição e ordens em uma direção
- Swap de Compra
- Swap de Venda
- Taxa de swap
- Taxa de Swap de Segunda para Terça
- Taxa de Swap de Terça para Quarta
- Taxa de Swap de Quarta para Quinta
- Taxa de Swap de Quinta para Sexta
- Taxa de Swap de Sexta para Sábado
- Taxa de Swap de Sábado para Domingo
- Margem Inicial
- Margem de Manutenção
- Volume de Negociação na Sessão Atual
- Volume de Negociação na Sessão Atual
- Volume de Posições em Aberto
- Volume de Ordens de Compra
- Volume de Ordens de Venda
- Preço de Abertura da Sessão
- Preço de Fechamento da Sessão
- Preço Médio Ponderado da Sessão
- Preço de Liquidação da Sessão
- Preço Mínimo na Sessão
- Preço Máximo na Sessão
- Tamanho do Contrato para uma Posição Hedge
- Variação Atual da Cotação em Percentagem
- Volatilidade da Cotação em Percentagem
- Preço Teórico da Opção
- Delta da Opção/Warrant
- Theta da Opção/Warrant
- Gamma da Opção/Warrant
- Vega da Opção/Warrant
- Rho da Opção/Warrant
- Omega da Opção/Warrant
- Obtenção e exibição das propriedades de string do símbolo
- Nome do Ativo Subjacente
- Categoria ou Setor do Instrumento
- País do Instrumento Financeiro
- Setor da Economia
- Indústria da Economia
- Moeda Base
- Moeda de Lucro
- Moeda de Margem
- Fonte de Cotação
- Descrição do Símbolo
- Nome da Bolsa
- Fórmula do Símbolo Personalizado
- Nome ISIN no Sistema
- Endereço da Página na Internet
- Caminho na Árvore de Símbolos
- Função que imprime no log todos os dados do símbolo
- Considerações finais
Introdução
Ao imprimir informações estruturadas e formatadas no log usando PrintFormat(), simplesmente organizamos os dados em um formato especificado e os exibimos no log do terminal. Essas informações formatadas deixam de estar disponíveis para utilização posterior. Para reimprimi-las, é necessário formatá-las novamente usando PrintFormat(). Seria bom ter a capacidade de reutilizar dados formatados uma vez no formato desejado. E essa capacidade existe na função StringFormat(). Ao contrário de seu "parente" PrintFormat(), que simplesmente imprime os dados no log, esta função retorna uma string formatada. Isso permite o uso posterior dessa string no programa, já que as informações formatadas como desejado não são perdidas. Isso é muito mais conveniente, porque você pode armazenar strings formatadas em variáveis ou arrays e usá-las novamente. Isso é muito mais flexível e conveniente.
Este artigo será mais como um material de referência expandido, com ênfase em modelos de funções prontas para uso, em vez de algo educacional.
StringFormat(). Visão geral concisa
A função StringFormat() formata os parâmetros recebidos e retorna uma string formatada. As regras de formatação da string são exatamente as mesmas que as da função PrintFormat(). Essas regras foram detalhadamente discutidas no artigo "Aprendendo PrintFormat() e usando exemplos prontos". Primeiro, é passada uma string com especificadores de texto e formato, seguida dos dados necessários separados por vírgula que devem substituir os especificadores na string. Cada especificador deve ter seu próprio conjunto de dados em ordem estrita.
A função permite que você reutilize uma string formatada várias vezes no programa. Hoje, usaremos essa capacidade ao criar modelos para exibir as propriedades do símbolo no log. Para cada propriedade, criaremos duas funções: a primeira irá formatar e retornar uma string, e a segunda irá imprimir a string recebida no log.
Formatação de strings
Para conhecer as regras de formatação de strings, você pode ler a seção sobre todos os especificadores no artigo sobre PrintFormat(). Para entender o funcionamento dos especificadores que indicam o tamanho dos dados (h | l | ll | I32 | I64), você pode usar um script que mostra claramente a diferença entre a saída de dados de diferentes tamanhos:
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- long x = (long)0xFFFFFFFF * 1000; Print("\nDEC: ", typename(x)," x=",x,"\n---------"); Print("%hu: ", StringFormat("%hu",x)); Print("%u: ", StringFormat("%u",x)); Print("%I64u: ",StringFormat("%I64u",x)); Print("%llu: ", StringFormat("%llu",x)); Print("\nHEX: ", typename(x)," x=",StringFormat("%llx",x),"\n---------"); Print("%hx: ", StringFormat("%hx",x)); Print("%x: ", StringFormat("%x",x)); Print("%I64x: ",StringFormat("%I64x",x)); Print("%llx: ", StringFormat("%llx",x)); }
Resultado
2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) DEC: long x=4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hu: 64536 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %u: 4294966296 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64u: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llu: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) HEX: long x=3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hx: fc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %x: fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64x: 3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llx: 3e7fffffc18
Provavelmente, este é o único especificador que não foi totalmente abordado no artigo sobre o PrintFormat().
Saída formatada das propriedades do símbolo
Para os propósitos deste artigo, precisaremos escrever duas funções para cada propriedade do símbolo. A primeira função formatará a string no formato adequado para impressão, enquanto a segunda imprimirá no log a string obtida pela primeira função. Para seguir o mesmo princípio de formatação de strings que foi adotado no artigo sobre o PrintFormat, também passaremos a margem esquerda e a largura do campo do cabeçalho para cada função. Dessa forma, manteremos uma formatação consistente para a saída no log das propriedades da conta e do símbolo.
Para obter o tempo em milissegundos, criaremos uma função que retornará uma string no formato Date Time.Msc com base na função discutida no artigo anterior sobre o PrintFormat:
//+------------------------------------------------------------------+ //| Accept a date in ms, return time in Date Time.Msc format | //+------------------------------------------------------------------+ string TimeMSC(const long time_msc) { return StringFormat("%s.%.3hu",string((datetime)time_msc / 1000),time_msc % 1000); /* Sample output: 2023.07.13 09:31:58.177 */ }
Diferentemente da função criada anteriormente, aqui retornaremos apenas a data e a hora em milissegundos na string. O restante da formatação será realizado nas funções que exibem os dados nesse formato de tempo.
Então, começaremos com os seguintes itens: propriedades inteiras, propriedades de ponto flutuante e propriedades de texto do símbolo. Escreveremos as funções na ordem em que as propriedades são listadas na seção de ajuda "Informações sobre o instrumento".
Obtendo e exibindo as propriedades inteiras do símbolo ENUM_SYMBOL_INFO_INTEGER
Atraso de dados (SYMBOL_SUBSCRIPTION_DELAY)
Indica que os dados do símbolo estão sendo fornecidos com atraso. Essa propriedade só pode ser solicitada para símbolos selecionados na Observação do Mercado (SYMBOL_SELECT = true). Para símbolos não selecionados, será gerado um erro ERR_MARKET_NOT_SELECTED (4302).
Retorna o valor da propriedade como uma string com um cabeçalho. Para a string formatada, você pode especificar um recuo da margem esquerda e a largura do campo do cabeçalho:
//+------------------------------------------------------------------+ //| Return the flag | //} of symbol data latency as a string | //+------------------------------------------------------------------+ string SymbolSubscriptionDelay(const string symbol,const uint header_width=0,const uint indent=0) { //--- Create a variable to store the error text //string error_str; //--- If symbol does not exist, return the error text if(!SymbolInfoInteger(symbol,SYMBOL_EXIST)) return StringFormat("%s: Error. Symbol '%s' is not exist",__FUNCTION__,symbol); //--- If a symbol is not selected in MarketWatch, try to select it if(!SymbolInfoInteger(symbol,SYMBOL_SELECT)) { //--- If unable to select a symbol in MarketWatch, return the error text if(!SymbolSelect(symbol,true)) return StringFormat("%s: Failed to select '%s' symbol in MarketWatch. Error %lu",__FUNCTION__,symbol,GetLastError()); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Subscription Delay:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SUBSCRIPTION_DELAY) ? "Yes" : "No"); /* Sample output: Subscription Delay: No */ }
Aqui, antes de solicitar os dados, primeiro verificamos se o símbolo existe e, em caso de verificação bem-sucedida, selecionamos o símbolo na janela Observação do Mercado. Se o símbolo não existir ou não puder ser selecionado, a função retornará um texto com a descrição do erro.
Ele imprime no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flag | //| of the symbol data latency in the journal | //+------------------------------------------------------------------+ void SymbolSubscriptionDelayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSubscriptionDelay(symbol,header_width,indent)); }
Setor da economia (SYMBOL_SECTOR)
O setor da economia ao qual o símbolo está relacionado.
//+------------------------------------------------------------------+ //| Return the economic sector as a string | //| a symbol belongs to | //+------------------------------------------------------------------+ string SymbolSector(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the economic sector ENUM_SYMBOL_SECTOR symbol_sector=(ENUM_SYMBOL_SECTOR)SymbolInfoInteger(symbol,SYMBOL_SECTOR); //--- "Cut out" the sector name from the string obtained from enum string sector=StringSubstr(EnumToString(symbol_sector),7); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(sector.Lower()) sector.SetChar(0,ushort(sector.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(sector,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,sector); /* Sample output: Sector: Currency */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Economy sector the symbol belongs to in the journal | //+------------------------------------------------------------------+ void SymbolSectorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSector(symbol,header_width,indent)); }
Setor da indústria (SYMBOL_INDUSTRY)
Setor da indústria ou ramo da economia ao qual o símbolo está relacionado.
//+------------------------------------------------------------------+ //| Return the industry type or economic sector as a string | //| the symbol belongs to | //+------------------------------------------------------------------+ string SymbolIndustry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get industry type value ENUM_SYMBOL_INDUSTRY symbol_industry=(ENUM_SYMBOL_INDUSTRY)SymbolInfoInteger(symbol,SYMBOL_INDUSTRY); //--- "Cut out" the industry type from the string obtained from enum string industry=StringSubstr(EnumToString(symbol_industry),9); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(industry.Lower()) industry.SetChar(0,ushort(industry.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(industry,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,industry); /* Sample output: Industry: Undefined */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the industry type or economic sector | //| the symbol belongs to | //+------------------------------------------------------------------+ void SymbolIndustryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustry(symbol,header_width,indent)); }
Símbolo personalizado (SYMBOL_CUSTOM)
Indica se o símbolo é personalizado, ou seja, criado artificialmente com base em outros símbolos da Observação do Mercado e/ou fontes de dados externas.
//+------------------------------------------------------------------+ //| Return the flag | //| of a custom symbol | //+------------------------------------------------------------------+ string SymbolCustom(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Custom symbol:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_CUSTOM) ? "Yes" : "No"); /* Sample output: Custom symbol: No */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flag | //| of a custom symbol | //+------------------------------------------------------------------+ void SymbolCustomPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCustom(symbol,header_width,indent)); }
Cor de fundo na Observação do Mercado (SYMBOL_BACKGROUND_COLOR)
A cor de fundo que destaca o símbolo na Observação do Mercado.
//+------------------------------------------------------------------+ //| Return the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ string SymbolBackgroundColor(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Background color:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the symbol background color in Market Watch color back_color=(color)SymbolInfoInteger(symbol,SYMBOL_BACKGROUND_COLOR); //--- Return the property value with a header having the required width and indentation //--- If a default color is set for a symbol (0xFF000000), return 'Default', otherwise - return its string description return StringFormat("%*s%-*s%-s",indent,"",w,header,back_color==0xFF000000 ? "Default" : ColorToString(back_color,true)); /* Sample output: Background color: Default */ }
Para a cor de fundo, pode ser definida uma cor totalmente transparente, que o ColorToString() retornará como preto (0, 0, 0). Mas isso não é verdade. Isso é chamado de cor padrão: 0xFF000000 — CLR_DEFAULT. Tem um valor RGB de zero, que corresponde à cor preta, mas é totalmente transparente. A cor padrão possui um canal alfa que define a total transparência do fundo como 0xFF000000. Portanto, antes de exibir o nome da cor usando o ColorToString(), verificamos se ela é igual a CLR_DEFAULT.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Displays the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ void SymbolBackgroundColorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBackgroundColor(symbol,header_width,indent)); }
Preço para construção de barras (SYMBOL_CHART_MODE)
O tipo de preço para a construção das barras - Bid ou Last.
//+------------------------------------------------------------------+ //| Return the price type for building bars as a string | //+------------------------------------------------------------------+ string SymbolChartMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get price type used for generating bars ENUM_SYMBOL_CHART_MODE symbol_chart_mode=(ENUM_SYMBOL_CHART_MODE)SymbolInfoInteger(symbol,SYMBOL_CHART_MODE); //--- "Cut out" price type from the string obtained from enum string chart_mode=StringSubstr(EnumToString(symbol_chart_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(chart_mode.Lower()) chart_mode.SetChar(0,ushort(chart_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Chart mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,chart_mode); /* Sample output: Chart mode: Bid */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Log the price type for building bars | //+------------------------------------------------------------------+ void SymbolChartModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolChartMode(symbol,header_width,indent)); }
Existência do símbolo (SYMBOL_EXIST)
Indica se um símbolo com esse nome existe.
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ string SymbolExists(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exists:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_EXIST) ? "Yes" : "No"); /* Sample output: Exists: Yes */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ void SymbolExistsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExists(symbol,header_width,indent)); }
Selecionado na Observação do Mercado (SYMBOL_SELECT)
Indica se o símbolo está selecionado na Observação do Mercado.
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is selected in Market Watch | //+------------------------------------------------------------------+ string SymbolSelected(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Selected:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SELECT) ? "Yes" : "No"); /* Sample output: Selected: Yes */ }
Exibe no log o valor retornado pela primeira função:
//+--------------------------------------------------------------------------------+ //| Display the flag, that the symbol is selected in Market Watch, in the journal | //+--------------------------------------------------------------------------------+ void SymbolSelectedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSelected(symbol,header_width,indent)); }
Visível na Observação do Mercado (SYMBOL_VISIBLE)
Indica se o símbolo selecionado é visível na Observação do Mercado.
Alguns símbolos (geralmente, cruzamentos de moedas necessários para cálculos de margem e lucro na moeda base) são selecionados automaticamente, mas podem não ser visíveis na Observação do Mercado. Para torná-los visíveis, esses símbolos devem ser selecionados explicitamente.
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ string SymbolVisible(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Visible:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_VISIBLE) ? "Yes" : "No"); /* Sample output: Visible: Yes */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ void SymbolVisiblePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVisible(symbol,header_width,indent)); }
Número de negociações na sessão atual (SYMBOL_SESSION_DEALS)
//+------------------------------------------------------------------+ //| Return the number of deals in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionDeals(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session deals:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_DEALS)); /* Sample output: Session deals: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the number of deals in the current session in the journal| //+------------------------------------------------------------------+ void SymbolSessionDealsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionDeals(symbol,header_width,indent)); }
Número de ordens de compra (SYMBOL_SESSION_BUY_ORDERS)
O número total de ordens de compra no momento.
//+------------------------------------------------------------------+ //| Return the total number | //| of current buy orders as a string | //+------------------------------------------------------------------+ string SymbolSessionBuyOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Buy orders: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the total number of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrders(symbol,header_width,indent)); }
Número de ordens de venda (SYMBOL_SESSION_SELL_ORDERS)
O número total de ordens de venda no momento.
//+------------------------------------------------------------------+ //| Return the total number | //| of current sell orders as a string | //+------------------------------------------------------------------+ string SymbolSessionSellOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Sell orders: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the total number of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrders(symbol,header_width,indent)); }
Volume na última negociação (SYMBOL_VOLUME)
Volume - o volume na última negociação.
//+------------------------------------------------------------------+ //| Return the last trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUME)); /* Sample output: Volume: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the last trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolume(symbol,header_width,indent)); }
Volume máximo no dia (SYMBOL_VOLUMEHIGH)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume high:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMEHIGH)); /* Sample output: Volume high: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHigh(symbol,header_width,indent)); }
Volume mínimo no dia (SYMBOL_VOLUMELOW)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMELOW)); /* Sample output: Volume low: 0 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLow(symbol,header_width,indent)); }
Hora da última cotação (SYMBOL_TIME)
//+------------------------------------------------------------------+ //| Return the last quote time as a string | //+------------------------------------------------------------------+ string SymbolTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(string)(datetime)SymbolInfoInteger(symbol,SYMBOL_TIME)); /* Sample output: Time: 2023.07.13 21:05:12 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the last quote time in the journal | //+------------------------------------------------------------------+ void SymbolTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTime(symbol,header_width,indent)); }
Hora da última cotação em milissegundos (SYMBOL_TIME_MSC)
Hora da última cotação em milissegundos desde 1970.01.01.
//+------------------------------------------------------------------+ //| Return the last quote time as a string in milliseconds | //+------------------------------------------------------------------+ string SymbolTimeMSC(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time msc:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,TimeMSC(SymbolInfoInteger(symbol,SYMBOL_TIME_MSC))); /* Sample output: Time msc: 2023.07.13 21:09:24.327 */ }
Aqui, para obter a string de data e hora com milissegundos, usamos a função TimeMSC(), que foi criada no início.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the last quote time in milliseconds in the journal | //+------------------------------------------------------------------+ void SymbolTimeMSCPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTimeMSC(symbol,header_width,indent)); }
Dígitos (SYMBOL_DIGITS)
Número de casas decimais.
//+------------------------------------------------------------------+ //| Return the number of decimal places as a string | //+------------------------------------------------------------------+ string SymbolDigits(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Digits:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_DIGITS)); /* Sample output: Digits: 5 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the number of decimal places in the journal | //+------------------------------------------------------------------+ void SymbolDigitsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDigits(symbol,header_width,indent)); }
Spread (SYMBOL_SPREAD)
Tamanho do spread em pontos.
//+------------------------------------------------------------------+ //| Return the spread size in points as a string | //+------------------------------------------------------------------+ string SymbolSpread(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SPREAD)); /* Sample output: Spread: 7 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the spread size in points in the journal | //+------------------------------------------------------------------+ void SymbolSpreadPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpread(symbol,header_width,indent)); }
Spread flutuante (SYMBOL_SPREAD_FLOAT)
Indica se o spread é flutuante.
//+------------------------------------------------------------------+ //| Return the floating spread flag as a string | //+------------------------------------------------------------------+ string SymbolSpreadFloat(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Floating Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SPREAD_FLOAT) ? "Yes" : "No"); /* Sample output: Spread float: Yes */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the floating spread flag in the journal | //+------------------------------------------------------------------+ void SymbolSpreadFloatPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpreadFloat(symbol,header_width,indent)); }
Número de ordens no livro de ofertas (SYMBOL_TICKS_BOOKDEPTH)
Número máximo de ordens exibidas no livro de ofertas. Para instrumentos que não têm fila de ordens, o valor é 0.
//+------------------------------------------------------------------+ //| Return the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ string SymbolTicksBookDepth(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ticks book depth:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_TICKS_BOOKDEPTH)); /* Sample output: Ticks book depth: 10 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ void SymbolTicksBookDepthPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTicksBookDepth(symbol,header_width,indent)); }
Modo de cálculo do tamanho do contrato (SYMBOL_TRADE_CALC_MODE)
Modo de cálculo do tamanho do contrato.
//+------------------------------------------------------------------+ //| Return the contract price calculation mode as a string | //+------------------------------------------------------------------+ string SymbolTradeCalcMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_CALC_MODE symbol_trade_calc_mode=(ENUM_SYMBOL_CALC_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_CALC_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_calc_mode=StringSubstr(EnumToString(symbol_trade_calc_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_calc_mode.Lower()) trade_calc_mode.SetChar(0,ushort(trade_calc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(trade_calc_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade calculation mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_calc_mode); /* Sample output: Trade calculation mode: Forex */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the contract price calculation mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeCalcModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeCalcMode(symbol,header_width,indent)); }
Modo de execução das ordens (SYMBOL_TRADE_MODE)
//+------------------------------------------------------------------+ //| Return the order execution type as a string | //+------------------------------------------------------------------+ string SymbolTradeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_MODE symbol_trade_mode=(ENUM_SYMBOL_TRADE_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_mode=StringSubstr(EnumToString(symbol_trade_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_mode.Lower()) trade_mode.SetChar(0,ushort(trade_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_mode); /* Sample output: Trade mode: Full */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the order execution type in the journal | //+------------------------------------------------------------------+ void SymbolTradeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeMode(symbol,header_width,indent)); }
Data de início da negociação (SYMBOL_START_TIME)
Data de início da negociação do instrumento (geralmente usada para futuros).
//+------------------------------------------------------------------+ //| Return the trading start date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolStartTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Start time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_START_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Start time: 1970.01.01 00:00:00 (Not used) */ }
Se o valor não estiver definido (igual a zero), ele será exibido como 1970.01.01.00:00:00. Isso pode ser um pouco confuso. Por isso, nessa situação, após o valor da data e hora, é exibida a explicação "Não utilizado".
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the trading start date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolStartTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolStartTime(symbol,header_width,indent)); }
Data de término das negociações
Data de término das negociações do instrumento (geralmente usada para futuros).
//+------------------------------------------------------------------+ //| Return the trading end date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolExpirationTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Expiration time: 1970.01.01 00:00:00 (Not used) */ }
Se o valor não estiver definido (igual a zero), uma explicação "Não utilizado" é exibida após o valor da data e hora.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the trading end date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolExpirationTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationTime(symbol,header_width,indent)); }
Nível de Stops (SYMBOL_TRADE_STOPS_LEVEL)
A distância mínima em pontos a partir do preço de fechamento atual para definir ordens de Stop.
//+--------------------------------------------------------------------------+ //| Return the minimum indentation | //| from the current close price in points to place Stop orders as a string | //+--------------------------------------------------------------------------+ string SymbolTradeStopsLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Stops level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int stops_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL); string descr=(stops_level==0 ? " (By Spread)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,stops_level,descr); /* Sample output: Stops level: 0 (By Spread) */ }
Se o valor for igual a zero, isso não significa um nível de Stops zero ou sua ausência. Isso significa que o Nível de Stops depende do valor do Spread. Normalmente, é 2 * Spread, e uma nota explicativa "Por Spread" é exibida.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum indentation in points from the previous | //| close price for setting Stop orders in the journal | //+------------------------------------------------------------------+ void SymbolTradeStopsLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeStopsLevel(symbol,header_width,indent)); }
Nível de Congelamento (SYMBOL_TRADE_FREEZE_LEVEL)
A distância de congelamento das operações (em pontos).
//+------------------------------------------------------------------+ //| Return the distance of freezing | //| trading operations in points as a string | //+------------------------------------------------------------------+ string SymbolTradeFreezeLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Freeze level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int freeze_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_FREEZE_LEVEL); string descr=(freeze_level==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,freeze_level,descr); /* Sample output: Freeze level: 0 (Not used) */ }
Se o valor for igual a zero, isso significa que o Nível de Congelamento não é usado, e uma nota explicativa "Não utilizado" é exibida.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------------------+ //| Display the distance of freezing trading operations in points in the journal | //+------------------------------------------------------------------------------+ void SymbolTradeFreezeLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFreezeLevel(symbol,header_width,indent)); }
Modo de Execução de Ordens (SYMBOL_TRADE_EXEMODE)
//+------------------------------------------------------------------+ //| Return the trade execution mode as a string | //+------------------------------------------------------------------+ string SymbolTradeExeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_EXECUTION symbol_trade_exemode=(ENUM_SYMBOL_TRADE_EXECUTION)SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_exemode=StringSubstr(EnumToString(symbol_trade_exemode),23); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_exemode.Lower()) trade_exemode.SetChar(0,ushort(trade_exemode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade Execution mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_exemode); /* Sample output: Trade Execution mode: Instant */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the trade execution mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeExeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeExeMode(symbol,header_width,indent)); }
Modelo de Cálculo de Swap (SYMBOL_SWAP_MODE)
//+------------------------------------------------------------------+ //| Return the swap calculation model as a string | //+------------------------------------------------------------------+ string SymbolSwapMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_SYMBOL_SWAP_MODE symbol_swap_mode=(ENUM_SYMBOL_SWAP_MODE)SymbolInfoInteger(symbol,SYMBOL_SWAP_MODE); //--- "Cut out" the swap calculation model from the string obtained from enum string swap_mode=StringSubstr(EnumToString(symbol_swap_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_mode.Lower()) swap_mode.SetChar(0,ushort(swap_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(swap_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_mode); /* Sample output: Swap mode: Points */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap calculation model to the journal | //+------------------------------------------------------------------+ void SymbolSwapModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMode(symbol,header_width,indent)); }
Dia de Rollover de Triplo Swap (SYMBOL_SWAP_ROLLOVER3DAYS)
O dia da semana para o cálculo do Triplo Swap.
//+---------------------------------------------------------------------+ //| Return the day of the week for calculating triple swap as a string | //+---------------------------------------------------------------------+ string SymbolSwapRollover3Days(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_DAY_OF_WEEK symbol_swap_rollover3days=(ENUM_DAY_OF_WEEK)SymbolInfoInteger(symbol,SYMBOL_SWAP_ROLLOVER3DAYS); //--- Convert enum into a string with the name of the day string swap_rollover3days=EnumToString(symbol_swap_rollover3days); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_rollover3days.Lower()) swap_rollover3days.SetChar(0,ushort(swap_rollover3days.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Rollover 3 days:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_rollover3days); /* Sample output: Swap Rollover 3 days: Wednesday */ }
Exibe no log o valor retornado pela primeira função:
//+--------------------------------------------------------------------------+ //| Display the day of the week for calculating triple swap in the journal | //+--------------------------------------------------------------------------+ void SymbolSwapRollover3DaysPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapRollover3Days(symbol,header_width,indent)); }
Modo de Cálculo de Margem de Hedge (SYMBOL_MARGIN_HEDGED_USE_LEG)
O modo de cálculo de margem de hedge com base no maior lado (Comprar ou Vender).
//+------------------------------------------------------------------+ //| Return the calculation mode | //| of hedged margin using the larger leg as a string | //+------------------------------------------------------------------+ string SymbolMarginHedgedUseLeg(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged use leg:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_MARGIN_HEDGED_USE_LEG) ? "Yes" : "No"); /* Sample output: Margin hedged use leg: No */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the mode for calculating the hedged | //| margin using the larger leg in the journal | //+------------------------------------------------------------------+ void SymbolMarginHedgedUseLegPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedgedUseLeg(symbol,header_width,indent)); }
Flags dos Modos de Expiração de Ordens (SYMBOL_EXPIRATION_MODE)
Se o símbolo tiver a flag SYMBOL_EXPIRATION_SPECIFIED, ao enviar uma ordem pendente, você pode especificar até quando essa ordem pendente é válida.
//+------------------------------------------------------------------+ //| Return the flags of allowed order expiration modes as a string | //+------------------------------------------------------------------+ string SymbolExpirationMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order expiration modes int exp_mode=(int)SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((exp_mode&SYMBOL_EXPIRATION_GTC)==SYMBOL_EXPIRATION_GTC) flags+=(flags.Length()>0 ? "|" : "")+"GTC"; if((exp_mode&SYMBOL_EXPIRATION_DAY)==SYMBOL_EXPIRATION_DAY) flags+=(flags.Length()>0 ? "|" : "")+"DAY"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED)==SYMBOL_EXPIRATION_SPECIFIED) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED_DAY)==SYMBOL_EXPIRATION_SPECIFIED_DAY) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED_DAY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Expiration mode: GTC|DAY|SPECIFIED */ }
Exibe no log o valor retornado pela primeira função:
//+--------------------------------------------------------------------+ //| Display the flags of allowed order expiration modes in the journal | //+--------------------------------------------------------------------+ void SymbolExpirationModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationMode(symbol,header_width,indent)); }
Flags dos Modos de Preenchimento de Ordens (SYMBOL_FILLING_MODE)
As flags dos modos de preenchimento de ordens permitidos.
Ao enviar uma ordem, você pode especificar a política de preenchimento do volume solicitado na ordem de negociação. Para cada instrumento, não apenas um modo pode ser configurado, mas vários através da combinação de flags. A combinação de flags é expressa pela operação lógica OR (|), por exemplo, SYMBOL_FILLING_FOK|SYMBOL_FILLING_IOC. O tipo de preenchimento ORDER_FILLING_RETURN é permitido em qualquer modo de execução, exceto no modo "Execução de Mercado" (SYMBOL_TRADE_EXECUTION_MARKET).//+------------------------------------------------------------------+ //| Return the flags of allowed order filling modes as a string | //+------------------------------------------------------------------+ string SymbolFillingMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order filling modes int fill_mode=(int)SymbolInfoInteger(symbol,SYMBOL_FILLING_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Filling mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((fill_mode&ORDER_FILLING_FOK)==ORDER_FILLING_FOK) flags+=(flags.Length()>0 ? "|" : "")+"FOK"; if((fill_mode&ORDER_FILLING_IOC)==ORDER_FILLING_IOC) flags+=(flags.Length()>0 ? "|" : "")+"IOC"; if((fill_mode&ORDER_FILLING_BOC)==ORDER_FILLING_BOC) flags+=(flags.Length()>0 ? "|" : "")+"BOC"; if(SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE)!=SYMBOL_TRADE_EXECUTION_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"RETURN"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Filling mode: FOK|IOC|RETURN */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flags of allowed order filling modes in the journal | //+------------------------------------------------------------------+ void SymbolFillingModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFillingMode(symbol,header_width,indent)); }
Flags dos Tipos de Ordens Permitidos (SYMBOL_ORDER_MODE)
//+------------------------------------------------------------------+ //| Return flags of allowed order types as a string | //+------------------------------------------------------------------+ string SymbolOrderMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order types int order_mode=(int)SymbolInfoInteger(symbol,SYMBOL_ORDER_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse type flags into components string flags=""; if((order_mode&SYMBOL_ORDER_MARKET)==SYMBOL_ORDER_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"MARKET"; if((order_mode&SYMBOL_ORDER_LIMIT)==SYMBOL_ORDER_LIMIT) flags+=(flags.Length()>0 ? "|" : "")+"LIMIT"; if((order_mode&SYMBOL_ORDER_STOP)==SYMBOL_ORDER_STOP) flags+=(flags.Length()>0 ? "|" : "")+"STOP"; if((order_mode&SYMBOL_ORDER_STOP_LIMIT )==SYMBOL_ORDER_STOP_LIMIT ) flags+=(flags.Length()>0 ? "|" : "")+"STOP_LIMIT"; if((order_mode&SYMBOL_ORDER_SL)==SYMBOL_ORDER_SL) flags+=(flags.Length()>0 ? "|" : "")+"SL"; if((order_mode&SYMBOL_ORDER_TP)==SYMBOL_ORDER_TP) flags+=(flags.Length()>0 ? "|" : "")+"TP"; if((order_mode&SYMBOL_ORDER_CLOSEBY)==SYMBOL_ORDER_CLOSEBY) flags+=(flags.Length()>0 ? "|" : "")+"CLOSEBY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the flags of allowed order types in the journal | //+------------------------------------------------------------------+ void SymbolOrderModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderMode(symbol,header_width,indent)); }
Prazo de Validade das Ordens Stop Loss e Take Profit (SYMBOL_ORDER_GTC_MODE)
Prazo de validade das ordens Stop Loss e Take Profit quando SYMBOL_EXPIRATION_MODE=SYMBOL_EXPIRATION_GTC (Válida até cancelar).
//+------------------------------------------------------------------+ //| Return StopLoss and TakeProfit validity periods as a string | //+------------------------------------------------------------------+ string SymbolOrderGTCMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the validity period of pending, StopLoss and TakeProfit orders ENUM_SYMBOL_ORDER_GTC_MODE symbol_order_gtc_mode=(ENUM_SYMBOL_ORDER_GTC_MODE)SymbolInfoInteger(symbol,SYMBOL_ORDER_GTC_MODE); //--- Set the validity period description as 'GTC' string gtc_mode="GTC"; //--- If the validity period is not GTC if(symbol_order_gtc_mode!=SYMBOL_ORDERS_GTC) { //--- "Cut out" pending, StopLoss and TakeProfit order validity periods from the string obtained from enum StringSubstr(EnumToString(symbol_order_gtc_mode),14); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(gtc_mode.Lower()) gtc_mode.SetChar(0,ushort(gtc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(gtc_mode,"_"," "); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order GTC mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,gtc_mode); /* Sample output: Order GTC mode: GTC */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display StopLoss and TakeProfit validity periods in the journal | //+------------------------------------------------------------------+ void SymbolOrderGTCModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderGTCMode(symbol,header_width,indent)); }
Tipo de Opção (SYMBOL_OPTION_MODE)
//+------------------------------------------------------------------+ //| Return option type as a string | //+------------------------------------------------------------------+ string SymbolOptionMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the option type model ENUM_SYMBOL_OPTION_MODE symbol_option_mode=(ENUM_SYMBOL_OPTION_MODE)SymbolInfoInteger(symbol,SYMBOL_OPTION_MODE); //--- "Cut out" the option type from the string obtained from enum string option_mode=StringSubstr(EnumToString(symbol_option_mode),19); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_mode.Lower()) option_mode.SetChar(0,ushort(option_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_mode); /* Sample output: Option mode: European */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option type in the journal | //+------------------------------------------------------------------+ void SymbolOptionModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionMode(symbol,header_width,indent)); }
Direito da Opção (SYMBOL_OPTION_RIGHT)
//+------------------------------------------------------------------+ //| Return the option right as a string | //+------------------------------------------------------------------+ string SymbolOptionRight(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the option right value ENUM_SYMBOL_OPTION_RIGHT symbol_option_right=(ENUM_SYMBOL_OPTION_RIGHT)SymbolInfoInteger(symbol,SYMBOL_OPTION_RIGHT); //--- "Cut out" the option right from the string obtained from enum string option_right=StringSubstr(EnumToString(symbol_option_right),20); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_right.Lower()) option_right.SetChar(0,ushort(option_right.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_right,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option right:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_right); /* Sample output: Option right: Call */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option right in the journal | //+------------------------------------------------------------------+ void SymbolOptionRightPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionRight(symbol,header_width,indent)); }
Obtém e exibe as propriedades de ponto flutuante do símbolo ENUM_SYMBOL_INFO_DOUBLE
Preço de Bid (SYMBOL_BID)
Bid — a melhor oferta de venda.
//+------------------------------------------------------------------+ //| Return the Bid price as a string | //+------------------------------------------------------------------+ string SymbolBid(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BID)); /* Sample output: Bid: 1.31017 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Bid price in the journal | //+------------------------------------------------------------------+ void SymbolBidPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBid(symbol,header_width,indent)); }
Maior Bid do Dia (SYMBOL_BIDHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDHIGH)); /* Sample output: Bid High: 1.31422 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidHigh(symbol,header_width,indent)); }
Menor Bid do Dia (SYMBOL_BIDLOW)
//+------------------------------------------------------------------+ //| Return the minimum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDLOW)); /* Sample output: Bid Low: 1.30934 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidLow(symbol,header_width,indent)); }
Preço de Ask (SYMBOL_ASK)
Ask — a melhor oferta de compra.
//+------------------------------------------------------------------+ //| Return the Ask price as a string | //+------------------------------------------------------------------+ string SymbolAsk(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASK)); /* Sample output: Ask: 1.31060 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Ask price in the journal | //+------------------------------------------------------------------+ void SymbolAskPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAsk(symbol,header_width,indent)); }
Maior Ask do Dia (SYMBOL_ASKHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKHIGH)); /* Sample output: Ask High: 1.31427 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskHigh(symbol,header_width,indent)); }
Menor Ask do Dia (SYMBOL_ASKLOW)
//+------------------------------------------------------------------+ //| Return the minimum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKLOW)); /* Sample output: Ask Low: 1.30938 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskLow(symbol,header_width,indent)); }
Preço de Última Negociação (SYMBOL_LAST)
Preço da última negociação efetuada.
//+------------------------------------------------------------------+ //| Return the Last price as a string | //+------------------------------------------------------------------+ string SymbolLast(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LAST)); /* Sample output: Last: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Last price in the journal | //+------------------------------------------------------------------+ void SymbolLastPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLast(symbol,header_width,indent)); }
Maior Último do Dia (SYMBOL_LASTHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTHIGH)); /* Sample output: Last High: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastHigh(symbol,header_width,indent)); }
Menor Último do Dia (SYMBOL_LASTLOW)
//+------------------------------------------------------------------+ //| Return the minimum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTLOW)); /* Sample output: Last Low: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastLow(symbol,header_width,indent)); }
Volume da Última Negociação (SYMBOL_VOLUME_REAL)
Volume da última negociação realizada.
//+------------------------------------------------------------------+ //| Return the last executed trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUME_REAL)); /* Sample output: Volume real: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the last executed trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeReal(symbol,header_width,indent)); }
Maior Volume do Dia (SYMBOL_VOLUMEHIGH_REAL)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHighReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume High real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMEHIGH_REAL)); /* Sample output: Volume High real: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHighReal(symbol,header_width,indent)); }
Menor Volume do Dia (SYMBOL_VOLUMELOW_REAL)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLowReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume Low real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMELOW_REAL)); /* Sample output: Volume Low real: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLowReal(symbol,header_width,indent)); }
Preço de Execução da Opção (SYMBOL_OPTION_STRIKE)
Preço de execução da opção. Este é o preço pelo qual o comprador da opção pode comprar (em opções de compra) ou vender (em opções de venda) o ativo subjacente, e o vendedor da opção é obrigado a vender ou comprar a quantidade correspondente do ativo subjacente.
//+------------------------------------------------------------------+ //| Return the strike price as a string | //+------------------------------------------------------------------+ string SymbolOptionStrike(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option strike:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_OPTION_STRIKE)); /* Sample output: Option strike: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option strike price in the journal | //+------------------------------------------------------------------+ void SymbolOptionStrikePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionStrike(symbol,header_width,indent)); }
Ponto (SYMBOL_POINT)
O valor de um ponto.
//+------------------------------------------------------------------+ //| Return the point value as a string | //+------------------------------------------------------------------+ string SymbolPoint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Point:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_POINT)); /* Sample output: Point: 0.00001 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the point value in the journal | //+------------------------------------------------------------------+ void SymbolPointPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPoint(symbol,header_width,indent)); }
Valor do Tick de Negociação (SYMBOL_TRADE_TICK_VALUE)
Valor do Tick de Negociação para Posições Lucrativas (SYMBOL_TRADE_TICK_VALUE_PROFIT)
//+------------------------------------------------------------------+ //| Return the tick price as a string | //+------------------------------------------------------------------+ string SymbolTradeTickValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE)); /* Sample output: Tick value: 1.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the tick price in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValue(symbol,header_width,indent)); }
Valor do Tick de Negociação para Posições Lucrativas (SYMBOL_TRADE_TICK_VALUE_PROFIT)
Valor calculado do tick de negociação para posições lucrativas.
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a profitable position | //+------------------------------------------------------------------+ string SymbolTradeTickValueProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_PROFIT)); /* Sample output: Tick value profit: 1.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a profitable position | //+------------------------------------------------------------------+ void SymbolTradeTickValueProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueProfit(symbol,header_width,indent)); }
Valor do Tick de Negociação para Posições com Perdas (SYMBOL_TRADE_TICK_VALUE_LOSS)
Valor calculado do tick de negociação para posições com perdas.
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a losing position | //+------------------------------------------------------------------+ string SymbolTradeTickValueLoss(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value loss:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_LOSS)); /* Sample output: Tick value loss: 1.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a losing position | //+------------------------------------------------------------------+ void SymbolTradeTickValueLossPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueLoss(symbol,header_width,indent)); }
Menor Alteração de Preço (SYMBOL_TRADE_TICK_SIZE)
//+------------------------------------------------------------------+ //| Return the minimum price change as a string | //+------------------------------------------------------------------+ string SymbolTradeTickSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_SIZE)); /* Sample output: Tick size: 0.00001 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum price change in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickSize(symbol,header_width,indent)); }
Tamanho do Contrato de Negociação (SYMBOL_TRADE_CONTRACT_SIZE)
//+------------------------------------------------------------------+ //| Return the trading contract size as a string | //+------------------------------------------------------------------+ string SymbolTradeContractSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Contract size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_CONTRACT_SIZE),SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE)); /* Sample output: Contract size: 100000.00 GBP */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the trading contract size in the journal | //+------------------------------------------------------------------+ void SymbolTradeContractSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeContractSize(symbol,header_width,indent)); }
Juros Acumulados (SYMBOL_TRADE_ACCRUED_INTEREST)
Juros acumulados - parte dos juros de cupom de um título, calculada proporcionalmente ao número de dias desde a data de emissão do título de cupom ou da data do pagamento do cupom anterior.
//+------------------------------------------------------------------+ //| Return accrued interest as a string | //+------------------------------------------------------------------+ string SymbolTradeAccruedInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Accrued interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_ACCRUED_INTEREST)); /* Sample output: Accrued interest: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display accrued interest in the journal | //+------------------------------------------------------------------+ void SymbolTradeAccruedInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeAccruedInterest(symbol,header_width,indent)); }
Valor Nominal (SYMBOL_TRADE_FACE_VALUE)
Valor nominal - o valor inicial do título determinado pelo emitente.
//+------------------------------------------------------------------+ //| Return the face value as a string | //+------------------------------------------------------------------+ string SymbolTradeFaceValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Face value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_FACE_VALUE)); /* Sample output: Face value: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the face value in the journal | //+------------------------------------------------------------------+ void SymbolTradeFaceValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFaceValue(symbol,header_width,indent)); }
Taxa de Liquidez (SYMBOL_TRADE_LIQUIDITY_RATE)
Taxa de liquidez - a parcela do valor do ativo que pode ser usada como garantia.
//+------------------------------------------------------------------+ //| Return the liquidity rate as a string | //+------------------------------------------------------------------+ string SymbolTradeLiquidityRate(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Liquidity rate:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_LIQUIDITY_RATE)); /* Sample output: Liquidity rate: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the rate liquidity in the journal | //+------------------------------------------------------------------+ void SymbolTradeLiquidityRatePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeLiquidityRate(symbol,header_width,indent)); }
Volume Mínimo (SYMBOL_VOLUME_MIN)
Volume mínimo necessário para efetuar uma negociação.
//+------------------------------------------------------------------+ //| Return the minimum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MIN)); /* Sample output: Volume min: 0.01 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMin(symbol,header_width,indent)); }
Volume Máximo (SYMBOL_VOLUME_MAX)
Volume máximo permitido para efetuar uma negociação.
//+------------------------------------------------------------------+ //| Return the maximum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MAX)); /* Sample output: Volume max: 500.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMax(symbol,header_width,indent)); }
Incremento Mínimo no Volume (SYMBOL_VOLUME_STEP)
O menor incremento permitido no volume para efetuar uma negociação.
//+------------------------------------------------------------------+ //| Return the minimum volume change step as a string | //+------------------------------------------------------------------+ string SymbolVolumeStep(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume step:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)); /* Sample output: Volume step: 0.01 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the minimum volume change step in the journal | //+------------------------------------------------------------------+ void SymbolVolumeStepPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeStep(symbol,header_width,indent)); }
Limite de Volume (SYMBOL_VOLUME_LIMIT)
O volume total permitido para uma posição aberta e ordens pendentes na mesma direção (compra ou venda) para este símbolo. Por exemplo, com um limite de 5 lotes, você pode ter uma posição aberta para compra de 5 lotes e colocar uma ordem pendente Sell Limit de 5 lotes. No entanto, não é possível colocar uma ordem pendente Buy Limit (pois excederia o limite na mesma direção) ou colocar uma Sell Limit com mais de 5 lotes.
//+----------------------------------------------------------------------------+ //| Return the maximum acceptable | //| total volume of open position and pending orders for a symbol as a string | //+----------------------------------------------------------------------------+ string SymbolVolumeLimit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume limit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_LIMIT)); /* Sample output: Volume limit: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+----------------------------------------------------------------------------+ //| Display the maximum acceptable | //| total volume of open position and pending orders for a symbol as a string | //+----------------------------------------------------------------------------+ void SymbolVolumeLimitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLimit(symbol,header_width,indent)); }
Swap de Compra (SYMBOL_SWAP_LONG)
//+------------------------------------------------------------------+ //| Return the Buy swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapLong(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap long:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_LONG)); /* Sample output: Swap long: -0.20 */ }
Como o valor desse atributo pode ser negativo, é necessário ajustar os valores negativos uma posição à esquerda para que o sinal de "menos" fique à esquerda das outras colunas de dígitos no formato. Para fazer isso, diminuímos a largura do campo em 1 e definimos um espaço no especificador de formato, que desloca a linha uma posição à direita para valores não negativos, compensando a largura reduzida do campo em um caractere.
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Buy swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapLongPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapLong(symbol,header_width,indent)); }
Swap de Venda (SYMBOL_SWAP_SHORT)
//+------------------------------------------------------------------+ //| Return the Sell swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapShort(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap short:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_SHORT)); /* Sample output: Swap short: -2.20 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the Sell swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapShortPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapShort(symbol,header_width,indent)); }
Taxa de Swap no Domingo (SYMBOL_SWAP_SUNDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição do domingo para o próximo dia. Pode ter os seguintes valores:
0 - sem swaps,
1 - swap único,
3 - triplo swap.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ string SymbolSwapSunday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap sunday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SUNDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap sunday: 0 (no swap is charged) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ void SymbolSwapSundayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSunday(symbol,header_width,indent)); }
Taxa de Swap de Segunda para Terça (SYMBOL_SWAP_MONDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição de segunda-feira para terça-feira.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ string SymbolSwapMonday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap monday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_MONDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap monday: 1 (single swap) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ void SymbolSwapMondayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMonday(symbol,header_width,indent)); }
Taxa de Swap de Terça para Quarta (SYMBOL_SWAP_TUESDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição de terça-feira para quarta-feira.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ string SymbolSwapTuesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Tuesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_TUESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Tuesday: 1 (single swap) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ void SymbolSwapTuesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapTuesday(symbol,header_width,indent)); }
Taxa de Swap de Quarta para Quinta (SYMBOL_SWAP_WEDNESDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição de quarta-feira para quinta-feira.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ string SymbolSwapWednesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Wednesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_WEDNESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Wednesday: 3 (triple swap) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ void SymbolSwapWednesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapWednesday(symbol,header_width,indent)); }
Taxa de Swap de Quinta para Sexta (SYMBOL_SWAP_THURSDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição de quinta-feira para sexta-feira.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ string SymbolSwapThursday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Thursday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_THURSDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Thursday: 1 (single swap) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ void SymbolSwapThursdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapThursday(symbol,header_width,indent)); }
Taxa de Swap de Sexta para Sábado (SYMBOL_SWAP_FRIDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição da sexta-feira para o sábado.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ string SymbolSwapFriday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Friday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_FRIDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Friday: 1 (single swap) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ void SymbolSwapFridayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapFriday(symbol,header_width,indent)); }
Taxa de Swap de Sábado para Domingo (SYMBOL_SWAP_SATURDAY)
A taxa de swap (SYMBOL_SWAP_LONG ou SYMBOL_SWAP_SHORT) ao transferir uma posição do sábado para o domingo.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ string SymbolSwapSaturday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Saturday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SATURDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Saturday: 0 (no swap is charged) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ void SymbolSwapSaturdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSaturday(symbol,header_width,indent)); }
No total, para exibir a taxa de swap para cada dia da semana, temos 7 * 2 funções. No entanto, é possível criar uma função universal que retorne uma string com a descrição da taxa de swap para o dia da semana especificado e outra função para imprimir no log o resultado retornado pela primeira função. Vamos escrever:
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| for a specified day of the week | //+------------------------------------------------------------------+ string SymbolSwapByDay(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Get a text description of a day of the week string day=EnumToString(day_week); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(day.Lower()) day.SetChar(0,ushort(day.GetChar(0)-0x20)); //--- Create the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header=StringFormat("Swap %s:",day); uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Declare the property that needs to be requested in SymbolInfoDouble() int prop=SYMBOL_SWAP_SUNDAY; //--- Depending on the day of the week, indicate the requested property switch(day_week) { case MONDAY : prop=SYMBOL_SWAP_MONDAY; break; case TUESDAY : prop=SYMBOL_SWAP_TUESDAY; break; case WEDNESDAY : prop=SYMBOL_SWAP_WEDNESDAY; break; case THURSDAY : prop=SYMBOL_SWAP_THURSDAY; break; case FRIDAY : prop=SYMBOL_SWAP_FRIDAY; break; case SATURDAY : prop=SYMBOL_SWAP_SATURDAY; break; default/*SUNDAY*/ : prop=SYMBOL_SWAP_SUNDAY; break; } //--- Get the ratio by a selected property and define its description string double swap_coeff=SymbolInfoDouble(symbol,(ENUM_SYMBOL_INFO_DOUBLE)prop); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Sunday: 0 (no swap is charged) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| for a specified day of the week | //+------------------------------------------------------------------+ void SymbolSwapByDayPrint(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapByDay(symbol,day_week,header_width,indent)); }
Margem Inicial (SYMBOL_MARGIN_INITIAL)
A margem inicial refere-se ao valor necessário em moeda marginal para abrir uma posição de um lote. É usado para verificar os fundos do cliente ao entrar no mercado. Para obter informações sobre o tamanho da margem cobrada, dependendo do tipo e direção da ordem, use a função SymbolInfoMarginRate().
//+------------------------------------------------------------------+ //| Return the initial margin as a string | //+------------------------------------------------------------------+ string SymbolMarginInitial(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin initial:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_INITIAL),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin initial: 0.00 GBP */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the initial margin in the journal | //+------------------------------------------------------------------+ void SymbolMarginInitialPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginInitial(symbol,header_width,indent)); }
Margem de Manutenção (SYMBOL_MARGIN_MAINTENANCE)
A margem de manutenção para o instrumento. Se especificada, indica o tamanho da margem em moeda marginal do instrumento retida por lote. É usado para verificar os fundos do cliente ao alterar o estado da conta do cliente. Se a margem de manutenção for igual a 0, a margem inicial será usada. Para obter informações sobre o tamanho da margem cobrada, dependendo do tipo e direção da ordem, use a função SymbolInfoMarginRate().
//+------------------------------------------------------------------+ //| Return the maintenance margin for an instrument as a string | //+------------------------------------------------------------------+ string SymbolMarginMaintenance(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin maintenance:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_MAINTENANCE),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin maintenance: 0.00 GBP */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maintenance margin for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolMarginMaintenancePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginMaintenance(symbol,header_width,indent)); }
Volume de Negociação na Sessão Atual (SYMBOL_SESSION_VOLUME)
Volume total de negociações na sessão atual.
//+--------------------------------------------------------------------------+ //| Returns the total volume of trades in the current session as a string | //+--------------------------------------------------------------------------+ string SymbolSessionVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_VOLUME)); /* Sample output: Session volume: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+---------------------------------------------------------------------------+ //| Display the total volume of trades in the current session in the journal | //+---------------------------------------------------------------------------+ void SymbolSessionVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionVolume(symbol,header_width,indent)); }
Volume de Negociação na Sessão Atual (SYMBOL_SESSION_TURNOVER)
Volume total de negociações na sessão atual.
//+------------------------------------------------------------------+ //| Return the total turnover in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionTurnover(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session turnover:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_TURNOVER)); /* Sample output: Session turnover: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------------+ //| Displays the total turnover during the current session in the journal | //+------------------------------------------------------------------------+ void SymbolSessionTurnoverPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionTurnover(symbol,header_width,indent)); }
Volume de Posições em Aberto (SYMBOL_SESSION_INTEREST)
Volume total de posições em aberto.
//+------------------------------------------------------------------+ //| Return the total volume of open positions as a string | //+------------------------------------------------------------------+ string SymbolSessionInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_INTEREST)); /* Sample output: Session interest: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the total volume of open positions in the journal | //+------------------------------------------------------------------+ void SymbolSessionInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionInterest(symbol,header_width,indent)); }
Volume de Ordens de Compra (SYMBOL_SESSION_BUY_ORDERS_VOLUME)
O volume total das ordens de compra no momento atual.
//+------------------------------------------------------------------+ //| Return the current total volume of buy orders | //+------------------------------------------------------------------+ string SymbolSessionBuyOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_BUY_ORDERS_VOLUME)); /* Sample output: Session Buy orders volume: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the total volume of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrdersVolume(symbol,header_width,indent)); }
Volume de Ordens de Venda (SYMBOL_SESSION_SELL_ORDERS_VOLUME)
O volume total das ordens de venda no momento atual.
//+------------------------------------------------------------------+ //| Return the current total volume of sell orders | //+------------------------------------------------------------------+ string SymbolSessionSellOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_SELL_ORDERS_VOLUME)); /* Sample output: Session Sell orders volume: 0.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the total volume of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrdersVolume(symbol,header_width,indent)); }
Preço de Abertura da Sessão (SYMBOL_SESSION_OPEN)
//+------------------------------------------------------------------+ //| Return the session open price as a string | //+------------------------------------------------------------------+ string SymbolSessionOpen(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Open:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_OPEN)); /* Sample output: Session Open: 1.31314 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the session open price in the journal | //+------------------------------------------------------------------+ void SymbolSessionOpenPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionOpen(symbol,header_width,indent)); }
Preço de Fechamento da Sessão (SYMBOL_SESSION_CLOSE)
//+------------------------------------------------------------------+ //| Return the session close price as a string | //+------------------------------------------------------------------+ string SymbolSessionClose(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Close:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_CLOSE)); /* Sample output: Session Close: 1.31349 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the session close price in the journal | //+------------------------------------------------------------------+ void SymbolSessionClosePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionClose(symbol,header_width,indent)); }
Preço Médio Ponderado da Sessão (SYMBOL_SESSION_AW)
//+------------------------------------------------------------------+ //| Return the session average weighted price as a string | //+------------------------------------------------------------------+ string SymbolSessionAW(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session AW:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_AW)); /* Sample output: Session AW: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the weighted average session price in the journal | //+------------------------------------------------------------------+ void SymbolSessionAWPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionAW(symbol,header_width,indent)); }
Preço de Liquidação da Sessão (SYMBOL_SESSION_PRICE_SETTLEMENT)
//+------------------------------------------------------------------+ //| Return the settlement price of the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceSettlement(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price settlement:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_SETTLEMENT)); /* Sample output: Session price settlement: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+--------------------------------------------------------------------+ //| Display the settlement price of the current session in the journal | //+--------------------------------------------------------------------+ void SymbolSessionPriceSettlementPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceSettlement(symbol,header_width,indent)); }
Preço Mínimo na Sessão (SYMBOL_SESSION_PRICE_LIMIT_MIN)
O valor mínimo permitido para o preço na sessão.
//+------------------------------------------------------------------+ //| Return the minimum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MIN)); /* Sample output: Session price limit min: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Displays the minimum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMin(symbol,header_width,indent)); }
Preço Máximo na Sessão (SYMBOL_SESSION_PRICE_LIMIT_MAX)
O valor máximo permitido para o preço na sessão.
//+------------------------------------------------------------------+ //| Return the maximum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MAX)); /* Sample output: Session price limit max: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the maximum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMax(symbol,header_width,indent)); }
Tamanho do Contrato para uma Posição Hedge (SYMBOL_MARGIN_HEDGED)
O tamanho do contrato ou margem para uma posição hedge (posições em direções opostas no mesmo símbolo). Existem duas formas de calcular a margem para posições hedge, determinadas pela corretora.
Cálculo Básico:
- Se a margem inicial (SYMBOL_MARGIN_INITIAL) estiver definida para o instrumento, a margem de hedge é especificada como um valor absoluto (em dinheiro).
- Se a margem inicial não estiver definida (igual a 0), então SYMBOL_MARGIN_HEDGED indica o tamanho do contrato que será usado no cálculo da margem de acordo com a fórmula correspondente ao tipo de instrumento de negociação (SYMBOL_TRADE_CALC_MODE).
Cálculo com Base na Maior Posição:
- O valor de SYMBOL_MARGIN_HEDGED não é considerado.
- O volume de todas as posições curtas e longas no instrumento é calculado.
- Para cada lado, é calculado o preço médio ponderado de abertura, bem como o preço médio ponderado de conversão para a moeda da conta.
- Em seguida, a margem é calculada para o lado curto e para o lado longo de acordo com as fórmulas correspondentes ao tipo de instrumento (SYMBOL_TRADE_CALC_MODE).
- O maior valor é usado como resultado.
//+------------------------------------------------------------------+ //| Return the contract or margin size as a string | //| for a single lot of covered positions | //+------------------------------------------------------------------+ string SymbolMarginHedged(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_HEDGED)); /* Sample output: Margin hedged: 100000.00 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the contract or margin size in the journal | //| for a single lot of covered positions | //+------------------------------------------------------------------+ void SymbolMarginHedgedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedged(symbol,header_width,indent)); }
Variação Atual da Cotação em Percentagem (SYMBOL_PRICE_CHANGE)
A variação atual da cotação em relação ao final do dia de negociação anterior, expressa em percentagem.
//+------------------------------------------------------------------+ //| Return the change of the current price relative to | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceChange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price change:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_CHANGE)); /* Sample output: Price change: -0.28 % */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display in the journal | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ void SymbolPriceChangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceChange(symbol,header_width,indent)); }
Volatilidade da Cotação em Percentagem (SYMBOL_PRICE_VOLATILITY)
//+------------------------------------------------------------------+ //| Return the price volatility in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceVolatility(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price volatility:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_VOLATILITY)); /* Sample output: Price volatility: 0.00 % */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the price volatility in percentage in the journal | //+------------------------------------------------------------------+ void SymbolPriceVolatilityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVolatility(symbol,header_width,indent)); }
Preço Teórico da Opção (SYMBOL_PRICE_THEORETICAL)
//+------------------------------------------------------------------+ //| Return the theoretical price of an option as a string | //+------------------------------------------------------------------+ string SymbolPriceTheoretical(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theoretical:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THEORETICAL)); /* Sample output: Price theoretical: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the theoretical price of an option in the journal | //+------------------------------------------------------------------+ void SymbolPriceTheoreticalPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheoretical(symbol,header_width,indent)); }
Delta da Opção/Warrant (SYMBOL_PRICE_DELTA)
O delta da opção/warrant. Indica quantas unidades o preço da opção irá variar quando o preço do ativo subjacente variar em uma unidade.
//+------------------------------------------------------------------+ //| Return as the option/warrant delta as a string | //+------------------------------------------------------------------+ string SymbolPriceDelta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price delta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_DELTA)); /* Sample output: Price delta: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant delta in the journal | //+------------------------------------------------------------------+ void SymbolPriceDeltaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceDelta(symbol,header_width,indent)); }
Theta da Opção/Warrant (SYMBOL_PRICE_THETA)
O theta da opção/warrant. O número de pontos que o preço da opção perderá a cada dia devido ao decadência do tempo, ou seja, quando a data de expiração se aproximar.
//+------------------------------------------------------------------+ //| Return as the option/warrant theta as a string | //+------------------------------------------------------------------+ string SymbolPriceTheta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THETA)); /* Sample output: Price theta: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant theta in the journal | //+------------------------------------------------------------------+ void SymbolPriceThetaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheta(symbol,header_width,indent)); }
Gamma da Opção/Warrant (SYMBOL_PRICE_GAMMA)
A gamma da opção/warrant. Indica a taxa de mudança da delta - quão rapidamente ou lentamente o prêmio da opção muda.
//+------------------------------------------------------------------+ //| Return as the option/warrant gamma as a string | //+------------------------------------------------------------------+ string SymbolPriceGamma(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price gamma:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_GAMMA)); /* Sample output: Price gamma: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant gamma in the journal | //+------------------------------------------------------------------+ void SymbolPriceGammaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceGamma(symbol,header_width,indent)); }
Vega da Opção/Warrant (SYMBOL_PRICE_VEGA)
A vega da opção/warrant. Indica o número de pontos pelos quais o preço da opção mudará quando a volatilidade aumentar em 1%.
//+------------------------------------------------------------------+ //| Return as the option/warrant vega as a string | //+------------------------------------------------------------------+ string SymbolPriceVega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price vega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_VEGA)); /* Sample output: Price vega: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant vega in the journal | //+------------------------------------------------------------------+ void SymbolPriceVegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVega(symbol,header_width,indent)); }
Rho da Opção/Warrant (SYMBOL_PRICE_RHO)
O rho da opção/warrant. Reflete a sensibilidade do preço teórico da opção a uma mudança na taxa de juros em 1%.
//+------------------------------------------------------------------+ //| Return as the option/warrant rho as a string | //+------------------------------------------------------------------+ string SymbolPriceRho(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price rho:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_RHO)); /* Sample output: Price rho: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant rho in the journal | //+------------------------------------------------------------------+ void SymbolPriceRhoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceRho(symbol,header_width,indent)); }
Omega da Opção/Warrant (SYMBOL_PRICE_OMEGA)
O omega da opção/warrant. Elasticidade da opção - a alteração percentual relativa no preço da opção em relação à alteração percentual no preço do ativo subjacente.
//+------------------------------------------------------------------+ //| Return as the option/warrant omega as a string | //+------------------------------------------------------------------+ string SymbolPriceOmega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price omega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_OMEGA)); /* Sample output: Price omega: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant omega in the journal | //+------------------------------------------------------------------+ void SymbolPriceOmegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceOmega(symbol,header_width,indent)); }
Sensibilidade da Opção/Warrant (SYMBOL_PRICE_SENSITIVITY)
A sensibilidade da opção/warrant. Indica quantos pontos o preço do ativo subjacente da opção deve mudar para que o preço da opção mude em um ponto.
//+------------------------------------------------------------------+ //| Return the option/warrant sensitivity as a string | //+------------------------------------------------------------------+ string SymbolPriceSensitivity(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price sensitivity:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_SENSITIVITY)); /* Sample output: Price sensitivity: 0.00000 */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the option/warrant sensitivity in the journal | //+------------------------------------------------------------------+ void SymbolPriceSensitivityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceSensitivity(symbol,header_width,indent)); }
Obtenção e exibição das propriedades de string do símbolo
Nome do Ativo Subjacente (SYMBOL_BASIS)
O nome do ativo subjacente para o instrumento derivativo.
//+------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument | //+------------------------------------------------------------------+ string SymbolBasis(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Basis:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BASIS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Basis: '' (Not specified) */ }
Se o parâmetro não estiver definido e retornar uma string vazia, insira uma explicação (Não especificado) ao lado do parâmetro vazio.
Exibe no log o valor retornado pela primeira função:
//+----------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument in the journal| //+----------------------------------------------------------------------+ void SymbolBasisPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBasis(symbol,header_width,indent)); }
Categoria ou Setor do Instrumento (SYMBOL_CATEGORY)
O nome da categoria ou setor ao qual o instrumento financeiro pertence.
//+------------------------------------------------------------------+ //| Return the name of the financial instrument category or sector | //+------------------------------------------------------------------+ string SymbolCategory(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Category:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CATEGORY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Category: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the name of the financial instrument category or sector | //| in the journal | //+------------------------------------------------------------------+ void SymbolCategoryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCategory(symbol,header_width,indent)); }
País do Instrumento Financeiro (SYMBOL_COUNTRY)
O país ao qual o instrumento financeiro está relacionado.
//+------------------------------------------------------------------+ //| Return the country the trading instrument belongs to | //+------------------------------------------------------------------+ string SymbolCountry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Country:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_COUNTRY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Country: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+----------------------------------------------------------------------+ //| Display the country the trading instrument belongs to in the journal | //+----------------------------------------------------------------------+ void SymbolCountryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCountry(symbol,header_width,indent)); }
Setor da Economia (SYMBOL_SECTOR_NAME)
O setor da economia ao qual o instrumento financeiro está relacionado.
//+------------------------------------------------------------------+ //| Return the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolSectorName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_SECTOR_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Sector name: 'Currency' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display in the journal the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolSectorNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSectorName(symbol,header_width,indent)); }
Indústria da Economia (SYMBOL_INDUSTRY_NAME)
A indústria da economia ou tipo de indústria ao qual o instrumento financeiro está relacionado.
//+------------------------------------------------------------------+ //| Return the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolIndustryName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_INDUSTRY_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Industry name: 'Undefined' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display in the journal the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolIndustryNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustryName(symbol,header_width,indent)); }
Moeda Base (SYMBOL_CURRENCY_BASE)
A moeda base do instrumento.
//+------------------------------------------------------------------+ //| Return the instrument base currency | //+------------------------------------------------------------------+ string SymbolCurrencyBase(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency base:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency base: 'GBP' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the instrument base currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyBasePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyBase(symbol,header_width,indent)); }
Moeda de Lucro (SYMBOL_CURRENCY_PROFIT)
//+------------------------------------------------------------------+ //| Return the profit currency | //+------------------------------------------------------------------+ string SymbolCurrencyProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_PROFIT); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency profit: 'USD' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the profit currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyProfit(symbol,header_width,indent)); }
Moeda de Margem (SYMBOL_CURRENCY_MARGIN)
A moeda na qual a margem é calculada.
//+------------------------------------------------------------------+ //| Return margin currency | //+------------------------------------------------------------------+ string SymbolCurrencyMargin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency margin:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency margin: 'GBP' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the margin currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyMarginPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyMargin(symbol,header_width,indent)); }
Fonte de Cotação (SYMBOL_BANK)
A fonte da cotação atual.
//+------------------------------------------------------------------+ //| Return the current quote source | //+------------------------------------------------------------------+ string SymbolBank(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bank:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BANK); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Bank: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the current quote source in the journal | //+------------------------------------------------------------------+ void SymbolBankPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBank(symbol,header_width,indent)); }
Descrição do Símbolo (SYMBOL_DESCRIPTION)
Uma descrição em formato de texto do símbolo.
//+------------------------------------------------------------------+ //| Return the symbol string description | //+------------------------------------------------------------------+ string SymbolDescription(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Description:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_DESCRIPTION); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Description: 'Pound Sterling vs US Dollar' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the symbol string description in the journal | //+------------------------------------------------------------------+ void SymbolDescriptionPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDescription(symbol,header_width,indent)); }
Nome da Bolsa (SYMBOL_EXCHANGE)
O nome da bolsa ou plataforma de negociação na qual o símbolo é negociado.
//+------------------------------------------------------------------+ //| Return the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ string SymbolExchange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exchange:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_EXCHANGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Exchange: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display in the journal the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ void SymbolExchangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExchange(symbol,header_width,indent)); }
Fórmula do Símbolo Personalizado (SYMBOL_FORMULA)
A fórmula para calcular o preço de um símbolo personalizado. Se o nome do instrumento financeiro incluído na fórmula começar com um número ou conter caracteres especiais (" ", ".", "-", "&", "#" etc.), o nome desse instrumento deve estar entre aspas.
- Ativo sintético: "@ESU19"/EURCAD
- Spread calendário: "Si-9.13"-"Si-6.13"
- Índice do euro: 34.38805726 * pow(EURUSD,0.3155) * pow(EURGBP,0.3056) * pow(EURJPY,0.1891) * pow(EURCHF,0.1113) * pow(EURSEK,0.0785)
//+------------------------------------------------------------------+ //| Return a formula for constructing a custom symbol price | //+------------------------------------------------------------------+ string SymbolFormula(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Formula:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_FORMULA); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Formula: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the equation for constructing | //| the custom symbol price in the journal | //+------------------------------------------------------------------+ void SymbolFormulaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFormula(symbol,header_width,indent)); }
Nome ISIN no Sistema (SYMBOL_ISIN)
O nome do símbolo de negociação no sistema de Códigos de Identificação Internacional de Valores Mobiliários (ISIN - International Securities Identification Number). O código de identificação internacional de valores mobiliários é um código alfanumérico de 12 dígitos que identifica unicamente um valor mobiliário. A disponibilidade dessa propriedade do símbolo é determinada pelo servidor de negociação.
//+------------------------------------------------------------------+ //| Return the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ string SymbolISIN(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="ISIN:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_ISIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: ISIN: '' (Not specified) */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display in the journal the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ void SymbolISINPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolISIN(symbol,header_width,indent)); }
Endereço da Página na Internet (SYMBOL_PAGE)
O endereço da página na internet com informações sobre o símbolo. Esse endereço será exibido como um link ao visualizar as propriedades do símbolo na plataforma.
//+------------------------------------------------------------------+ //| Return the address of a web page with a symbol data | //+------------------------------------------------------------------+ string SymbolPage(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Page:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PAGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Page: 'https://www.mql5.com/en/quotes/currencies/gbpusd' */ }
Exibe no log o valor retornado pela primeira função:
//+--------------------------------------------------------------------+ //| Display the address of a web page with a symbol data in the journal| //+--------------------------------------------------------------------+ void SymbolPagePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPage(symbol,header_width,indent)); }
Caminho na Árvore de Símbolos (SYMBOL_PATH)
//+------------------------------------------------------------------+ //| Return the path in the symbol tree | //+------------------------------------------------------------------+ string SymbolPath(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Path:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PATH); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Path: 'Forex\GBPUSD' */ }
Exibe no log o valor retornado pela primeira função:
//+------------------------------------------------------------------+ //| Display the path in the symbol tree in the journal | //+------------------------------------------------------------------+ void SymbolPathPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPath(symbol,header_width,indent)); }
As funções apresentadas acima são logicamente idênticas entre si. Cada uma delas pode ser usada "como está" para registrar no log ou obter a propriedade necessária do símbolo como uma string. Por padrão, a linha de recuo é zero e a largura do campo de cabeçalho é igual à largura do texto do cabeçalho, ou seja, não há recuo nem alinhamento no texto da descrição da propriedade. Eles são necessários no caso de você desejar exibir um grupo de propriedades do símbolo para alinhar os campos de dados no log. Vamos escrever uma função assim.
Função que imprime no log todos os dados do símbolo
Com base nas funções criadas, vamos criar uma função que imprime no log todas as propriedades do símbolo na ordem em que estão listadas nas enumerações ENUM_SYMBOL_INFO_INTEGER, ENUM_SYMBOL_INFO_DOUBLE e
//+------------------------------------------------------------------+ //| Send all symbol properties to the journal | //+------------------------------------------------------------------+ void SymbolInfoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Display descriptions of integer properties according to their location in ENUM_SYMBOL_INFO_INTEGER Print("SymbolInfoInteger properties:"); SymbolSubscriptionDelayPrint(symbol,header_width,indent); SymbolSectorPrint(symbol,header_width,indent); SymbolIndustryPrint(symbol,header_width,indent); SymbolCustomPrint(symbol,header_width,indent); SymbolBackgroundColorPrint(symbol,header_width,indent); SymbolChartModePrint(symbol,header_width,indent); SymbolExistsPrint(symbol,header_width,indent); SymbolSelectedPrint(symbol,header_width,indent); SymbolVisiblePrint(symbol,header_width,indent); SymbolSessionDealsPrint(symbol,header_width,indent); SymbolSessionBuyOrdersPrint(symbol,header_width,indent); SymbolSessionSellOrdersPrint(symbol,header_width,indent); SymbolVolumePrint(symbol,header_width,indent); SymbolVolumeHighPrint(symbol,header_width,indent); SymbolVolumeLowPrint(symbol,header_width,indent); SymbolTimePrint(symbol,header_width,indent); SymbolTimeMSCPrint(symbol,header_width,indent); SymbolDigitsPrint(symbol,header_width,indent); SymbolSpreadPrint(symbol,header_width,indent); SymbolSpreadFloatPrint(symbol,header_width,indent); SymbolTicksBookDepthPrint(symbol,header_width,indent); SymbolTradeCalcModePrint(symbol,header_width,indent); SymbolTradeModePrint(symbol,header_width,indent); SymbolSymbolStartTimePrint(symbol,header_width,indent); SymbolSymbolExpirationTimePrint(symbol,header_width,indent); SymbolTradeStopsLevelPrint(symbol,header_width,indent); SymbolTradeFreezeLevelPrint(symbol,header_width,indent); SymbolTradeExeModePrint(symbol,header_width,indent); SymbolSwapModePrint(symbol,header_width,indent); SymbolSwapRollover3DaysPrint(symbol,header_width,indent); SymbolMarginHedgedUseLegPrint(symbol,header_width,indent); SymbolExpirationModePrint(symbol,header_width,indent); SymbolFillingModePrint(symbol,header_width,indent); SymbolOrderModePrint(symbol,header_width,indent); SymbolOrderGTCModePrint(symbol,header_width,indent); SymbolOptionModePrint(symbol,header_width,indent); SymbolOptionRightPrint(symbol,header_width,indent); //--- Display descriptions of real properties according to their location in ENUM_SYMBOL_INFO_DOUBLE Print("SymbolInfoDouble properties:"); SymbolBidPrint(symbol,header_width,indent); SymbolBidHighPrint(symbol,header_width,indent); SymbolBidLowPrint(symbol,header_width,indent); SymbolAskPrint(symbol,header_width,indent); SymbolAskHighPrint(symbol,header_width,indent); SymbolAskLowPrint(symbol,header_width,indent); SymbolLastPrint(symbol,header_width,indent); SymbolLastHighPrint(symbol,header_width,indent); SymbolLastLowPrint(symbol,header_width,indent); SymbolVolumeRealPrint(symbol,header_width,indent); SymbolVolumeHighRealPrint(symbol,header_width,indent); SymbolVolumeLowRealPrint(symbol,header_width,indent); SymbolOptionStrikePrint(symbol,header_width,indent); SymbolPointPrint(symbol,header_width,indent); SymbolTradeTickValuePrint(symbol,header_width,indent); SymbolTradeTickValueProfitPrint(symbol,header_width,indent); SymbolTradeTickValueLossPrint(symbol,header_width,indent); SymbolTradeTickSizePrint(symbol,header_width,indent); SymbolTradeContractSizePrint(symbol,header_width,indent); SymbolTradeAccruedInterestPrint(symbol,header_width,indent); SymbolTradeFaceValuePrint(symbol,header_width,indent); SymbolTradeLiquidityRatePrint(symbol,header_width,indent); SymbolVolumeMinPrint(symbol,header_width,indent); SymbolVolumeMaxPrint(symbol,header_width,indent); SymbolVolumeStepPrint(symbol,header_width,indent); SymbolVolumeLimitPrint(symbol,header_width,indent); SymbolSwapLongPrint(symbol,header_width,indent); SymbolSwapShortPrint(symbol,header_width,indent); SymbolSwapByDayPrint(symbol,SUNDAY,header_width,indent); SymbolSwapByDayPrint(symbol,MONDAY,header_width,indent); SymbolSwapByDayPrint(symbol,TUESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,WEDNESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,THURSDAY,header_width,indent); SymbolSwapByDayPrint(symbol,FRIDAY,header_width,indent); SymbolSwapByDayPrint(symbol,SATURDAY,header_width,indent); SymbolMarginInitialPrint(symbol,header_width,indent); SymbolMarginMaintenancePrint(symbol,header_width,indent); SymbolSessionVolumePrint(symbol,header_width,indent); SymbolSessionTurnoverPrint(symbol,header_width,indent); SymbolSessionInterestPrint(symbol,header_width,indent); SymbolSessionBuyOrdersVolumePrint(symbol,header_width,indent); SymbolSessionSellOrdersVolumePrint(symbol,header_width,indent); SymbolSessionOpenPrint(symbol,header_width,indent); SymbolSessionClosePrint(symbol,header_width,indent); SymbolSessionAWPrint(symbol,header_width,indent); SymbolSessionPriceSettlementPrint(symbol,header_width,indent); SymbolSessionPriceLimitMinPrint(symbol,header_width,indent); SymbolSessionPriceLimitMaxPrint(symbol,header_width,indent); SymbolMarginHedgedPrint(symbol,header_width,indent); SymbolPriceChangePrint(symbol,header_width,indent); SymbolPriceVolatilityPrint(symbol,header_width,indent); SymbolPriceTheoreticalPrint(symbol,header_width,indent); SymbolPriceDeltaPrint(symbol,header_width,indent); SymbolPriceThetaPrint(symbol,header_width,indent); SymbolPriceGammaPrint(symbol,header_width,indent); SymbolPriceVegaPrint(symbol,header_width,indent); SymbolPriceRhoPrint(symbol,header_width,indent); SymbolPriceOmegaPrint(symbol,header_width,indent); SymbolPriceSensitivityPrint(symbol,header_width,indent); //--- Display descriptions of string properties according to their location in ENUM_SYMBOL_INFO_STRING Print("SymbolInfoString properties:"); SymbolBasisPrint(symbol,header_width,indent); SymbolCategoryPrint(symbol,header_width,indent); SymbolCountryPrint(symbol,header_width,indent); SymbolSectorNamePrint(symbol,header_width,indent); SymbolIndustryNamePrint(symbol,header_width,indent); SymbolCurrencyBasePrint(symbol,header_width,indent); SymbolCurrencyProfitPrint(symbol,header_width,indent); SymbolCurrencyMarginPrint(symbol,header_width,indent); SymbolBankPrint(symbol,header_width,indent); SymbolDescriptionPrint(symbol,header_width,indent); SymbolExchangePrint(symbol,header_width,indent); SymbolFormulaPrint(symbol,header_width,indent); SymbolISINPrint(symbol,header_width,indent); SymbolPagePrint(symbol,header_width,indent); SymbolPathPrint(symbol,header_width,indent); } //+------------------------------------------------------------------+
Aqui, todas as propriedades do símbolo são simplesmente impressas no log, uma após a outra. Os tipos de propriedades são precedidos por cabeçalhos que indicam o tipo: inteiros, de ponto flutuante e de texto.
O resultado da chamada da função a partir de um script com uma largura de campo de cabeçalho de 28 caracteres e um recuo de 2 caracteres:
void OnStart() { //--- SymbolInfoPrint(Symbol(),28,2); /* Sample output: SymbolInfoInteger properties: Subscription Delay: No Sector: Currency Industry: Undefined Custom symbol: No Background color: Default Chart mode: Bid Exists: Yes Selected: Yes Visible: Yes Session deals: 0 Session Buy orders: 0 Session Sell orders: 0 Volume: 0 Volume high: 0 Volume low: 0 Time: 2023.07.14 22:07:01 Time msc: 2023.07.14 22:07:01.706 Digits: 5 Spread: 5 Spread float: Yes Ticks book depth: 10 Trade calculation mode: Forex Trade mode: Full Start time: 1970.01.01 00:00:00 (Not used) Expiration time: 1970.01.01 00:00:00 (Not used) Stops level: 0 (By Spread) Freeze level: 0 (Not used) Trade Execution mode: Instant Swap mode: Points Swap Rollover 3 days: Wednesday Margin hedged use leg: No Expiration mode: GTC|DAY|SPECIFIED Filling mode: FOK|IOC|RETURN Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY Order GTC mode: GTC Option mode: European Option right: Call SymbolInfoDouble properties: Bid: 1.30979 Bid High: 1.31422 Bid Low: 1.30934 Ask: 1.30984 Ask High: 1.31427 Ask Low: 1.30938 Last: 0.00000 Last High: 0.00000 Last Low: 0.00000 Volume real: 0.00 Volume High real: 0.00 Volume Low real: 0.00 Option strike: 0.00000 Point: 0.00001 Tick value: 1.00000 Tick value profit: 1.00000 Tick value loss: 1.00000 Tick size: 0.00001 Contract size: 100000.00 GBP Accrued interest: 0.00 Face value: 0.00 Liquidity rate: 0.00 Volume min: 0.01 Volume max: 500.00 Volume step: 0.01 Volume limit: 0.00 Swap long: -0.20 Swap short: -2.20 Swap Sunday: 0 (no swap is charged) Swap Monday: 1 (single swap) Swap Tuesday: 1 (single swap) Swap Wednesday: 3 (triple swap) Swap Thursday: 1 (single swap) Swap Friday: 1 (single swap) Swap Saturday: 0 (no swap is charged) Margin initial: 0.00 GBP Margin maintenance: 0.00 GBP Session volume: 0.00 Session turnover: 0.00 Session interest: 0.00 Session Buy orders volume: 0.00 Session Sell orders volume: 0.00 Session Open: 1.31314 Session Close: 1.31349 Session AW: 0.00000 Session price settlement: 0.00000 Session price limit min: 0.00000 Session price limit max: 0.00000 Margin hedged: 100000.00 Price change: -0.28 % Price volatility: 0.00 % Price theoretical: 0.00000 Price delta: 0.00000 Price theta: 0.00000 Price gamma: 0.00000 Price vega: 0.00000 Price rho: 0.00000 Price omega: 0.00000 Price sensitivity: 0.00000 SymbolInfoString properties: Basis: '' (Not specified) Category: '' (Not specified) Country: '' (Not specified) Sector name: 'Currency' Industry name: 'Undefined' Currency base: 'GBP' Currency profit: 'USD' Currency margin: 'GBP' Bank: '' (Not specified) Description: 'Pound Sterling vs US Dollar' Exchange: '' (Not specified) Formula: '' (Not specified) ISIN: '' (Not specified) Page: 'https://www.mql5.com/en/quotes/currencies/gbpusd' Path: 'Forex\GBPUSD' */ }
Como podemos ver, todos os dados estão dispostos de forma organizada em formato de tabela. Os valores negativos não se destacam no contexto geral devido ao seu recuo para a esquerda.
Isso é apenas um exemplo de como você pode usar as funções criadas anteriormente. Você pode usá-las diretamente em seus programas, compor a saída de algumas categorias de propriedades de símbolos ou adaptá-las de acordo com sua visão e necessidades.
Considerações finais
Nós examinamos funções para imprimir propriedades de contas e símbolos usando strings formatadas. Em seguida, veremos como imprimir no log algumas das estruturas implementadas no MQL5.
Traduzido do russo pela MetaQuotes Ltd.
Artigo original: https://www.mql5.com/ru/articles/12953





- Aplicativos de negociação gratuitos
- 8 000+ sinais para cópia
- Notícias econômicas para análise dos mercados financeiros
Você concorda com a política do site e com os termos de uso