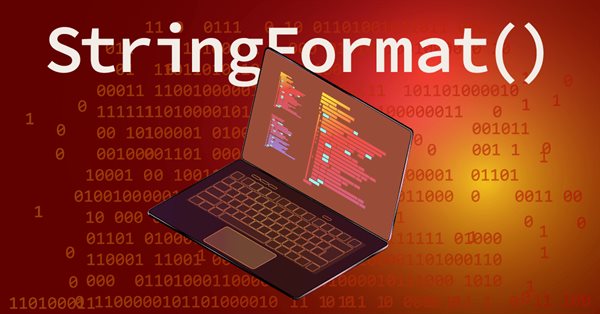
StringFormat(). Review and ready-made examples
Contents
- Introduction
- StringFormat(). Summary
- Formatting strings
- Formatted output of symbol properties
- Receiving and displaying integer properties of a symbol
- Data latency
- Economic sector
- Industry type
- Custom symbol
- Background color in Market Watch
- Price for building bars
- Symbol presence
- Selected in Market Watch
- Displayed in Market Watch
- Number of deals in the current session
- Number of Buy orders
- Number of Sell orders
- Last trade volume
- Maximum volume per day
- Minimum volume per day
- Time of the last quote
- Last quote time in ms
- Digits
- Spread
- Floating spread
- Number of requests in the market depth
- Contract price calculation mode
- Order execution type
- Trading start date
- Trading end date
- Stops Level
- Freeze Level
- Deal execution mode
- Swap calculation model
- Triple swap accrual day
- Hedged margin calculation mode
- Flags of order expiration modes
- Flags of order filling modes
- Flags of allowed order types
- StopLoss and TakeProfit validity periods
- Option type
- Receiving and displaying symbol real properties
- Bid price
- Highest Bid price of the day
- Lowest Bid price of the day
- Ask price
- Highest Ask price of the day
- Lowest Ask price of the day
- Last price
- Highest Last price of the day
- Lowest Last price of the day
- Last trade volume
- Maximum volume per day
- Minimum volume per day
- Option strike price
- Point
- Tick price
- Tick price for a profitable position
- Tick price for a losing position
- Minimum price change
- Trade contract size
- Accrued interest
- Face value
- Liquidity rate
- Minimum volume
- Highest volume
- Volume change step
- Maximum volume of position and orders in one direction
- Buy swap
- Sell swap
- Swap accrual ratio
- Swap accrual ratio from Monday to Tuesday
- Swap accrual ratio from Tuesday to Wednesday
- Swap accrual ratio from Wednesday to Thursday
- Swap accrual ratio from Thursday to Friday
- Swap accrual ratio from Friday to Saturday
- Swap accrual ratio from Saturday to Sunday
- Initial margin
- Maintenance margin
- Trade volume in the current session
- Current session turnover
- Volume of open positions
- Volume of buy orders
- Volume of sell orders
- Session open price
- Session close price
- Session average weighted price
- Current session settlement price
- Minimum price per session
- Maximum price per session
- Contract size for one lot of covered positions
- Change in current price in percentage
- Price volatility in percentage
- Theoretical option price
- Option/warrant delta
- Option/warrant theta
- Option/warrant gamma
- Option/warrant vega
- Option/warrant rho
- Option/warrant omega
- Receiving and displaying symbol string properties
- Underlying asset name
- Instrument category or sector
- Financial instrument country
- Economic sector
- Economy branch
- Base currency
- Profit currency
- Collateral currency
- Quote source
- Symbol description
- Exchange name
- Custom symbol equation
- ISIN system name
- Web page address
- Path in the symbol tree
- Function that prints all symbol data to the journal
- Conclusion
Introduction
When logging structured and formatted information using PrintFormat(), we simply format the data according to the specified format and display it in the terminal journal. Such formatted information is no longer available for subsequent use. To display it again, we need to resort to formatting using PrintFormat() again. It would be nice to be able to reuse data once formatted into the desired type. Actually, there is such a possibility — the StringFormat() function. Unlike PrintFormat(), which simply prints data to the journal, this function returns a formatted string. This allows us to use this string further in the program. In other words, the data formatted into the required form is not lost. This is much more convenient. You can save formatted strings into variables or an array and take them for repeated use. It is much more flexible and convenient.
Rather than being educational, the current article represents an extended reference material with an emphasis on ready-made function templates.
StringFormat(). Summary
The StringFormat() function formats the received parameters and returns the formatted string. The string formatting rules are exactly the same as for the PrintFormat() function. They were considered in detail in the article "Studying PrintFormat() and applying ready-made examples". A string with text and format specifiers is passed first followed by the comma-separated necessary data that should replace the specifiers in the string. Each specifier must have its own data set in strict order.
The function allows a once formatted string to be reused in a program. We will use this feature here when creating templates for displaying symbol properties to the journal. We will create two functions for each property: the first will format and return a string, while the second one will print the resulting string in the journal.
Formatting strings
To familiarize yourself with the rules for formatting strings, you can read the section about all the specifiers in the article about PrintFormat(). To clarify the operation of specifiers indicating the data size (h | l | ll | I32 | I64), you can use this script, which clearly shows the difference between the output of data of different sizes:
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- long x = (long)0xFFFFFFFF * 1000; Print("\nDEC: ", typename(x)," x=",x,"\n---------"); Print("%hu: ", StringFormat("%hu",x)); Print("%u: ", StringFormat("%u",x)); Print("%I64u: ",StringFormat("%I64u",x)); Print("%llu: ", StringFormat("%llu",x)); Print("\nHEX: ", typename(x)," x=",StringFormat("%llx",x),"\n---------"); Print("%hx: ", StringFormat("%hx",x)); Print("%x: ", StringFormat("%x",x)); Print("%I64x: ",StringFormat("%I64x",x)); Print("%llx: ", StringFormat("%llx",x)); }
Result:
2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) DEC: long x=4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hu: 64536 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %u: 4294966296 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64u: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llu: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) HEX: long x=3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hx: fc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %x: fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64x: 3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llx: 3e7fffffc18
This is probably the only specifier that was not fully covered in the article about PrintFormat().
Formatted output of symbol properties
For the this article, we will need to write two functions for each symbol property. The first function will format the string into the form required for printing, and the second will print the string received by the first function into the journal. To follow the same principle of string formatting adopted in the article about PrintFormat(), we will also pass the string indentation from the left edge and the width of the header field to each function. This way we will maintain uniform formatting for logging account and symbol properties.
To get time in milliseconds, let's create a function that returns a string with time in the Date Time.Msc format based on the function discussed in the previous article about PrintFormat():
//+------------------------------------------------------------------+ //| Accept a date in ms, return time in Date Time.Msc format | //+------------------------------------------------------------------+ string TimeMSC(const long time_msc) { return StringFormat("%s.%.3hu",string((datetime)time_msc / 1000),time_msc % 1000); /* Sample output: 2023.07.13 09:31:58.177 */ }
Unlike the previously created function, here we will return only the date and time in milliseconds in a string. The rest of the formatting will be done in functions that display data in this time format.
So, let's start in the following order: integer, real and string symbol properties. The functions will be set in the order set in the Symbol Properties documentation section.
Receiving and displaying integer properties of a symbol ENUM_SYMBOL_INFO_INTEGER
Data latency (SYMBOL_SUBSCRIPTION_DELAY)
Symbol data arrives with a delay. The property can be requested only for symbols selected in MarketWatch (SYMBOL_SELECT = true). The ERR_MARKET_NOT_SELECTED (4302) error is generated for other symbols
Return string value of the property with a header. For the formatted string, it is possible to specify the left margin and width of the header field:
//+------------------------------------------------------------------+ //| Return the flag | //} of symbol data latency as a string | //+------------------------------------------------------------------+ string SymbolSubscriptionDelay(const string symbol,const uint header_width=0,const uint indent=0) { //--- Create a variable to store the error text //string error_str; //--- If symbol does not exist, return the error text if(!SymbolInfoInteger(symbol,SYMBOL_EXIST)) return StringFormat("%s: Error. Symbol '%s' is not exist",__FUNCTION__,symbol); //--- If a symbol is not selected in MarketWatch, try to select it if(!SymbolInfoInteger(symbol,SYMBOL_SELECT)) { //--- If unable to select a symbol in MarketWatch, return the error text if(!SymbolSelect(symbol,true)) return StringFormat("%s: Failed to select '%s' symbol in MarketWatch. Error %lu",__FUNCTION__,symbol,GetLastError()); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Subscription Delay:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SUBSCRIPTION_DELAY) ? "Yes" : "No"); /* Sample output: Subscription Delay: No */ }
Here, before requesting data, the presence of such a symbol is first checked, then, if the presence check is successful, the symbol is selected in the MarketWatch window. If there is no such symbol, or it was not possible to select it, the function returns the error text.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flag | //| of the symbol data latency in the journal | //+------------------------------------------------------------------+ void SymbolSubscriptionDelayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSubscriptionDelay(symbol,header_width,indent)); }
Economic sector (SYMBOL_SECTOR)
Economic sector the symbol belongs to.
//+------------------------------------------------------------------+ //| Return the economic sector as a string | //| a symbol belongs to | //+------------------------------------------------------------------+ string SymbolSector(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the economic sector ENUM_SYMBOL_SECTOR symbol_sector=(ENUM_SYMBOL_SECTOR)SymbolInfoInteger(symbol,SYMBOL_SECTOR); //--- "Cut out" the sector name from the string obtained from enum string sector=StringSubstr(EnumToString(symbol_sector),7); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(sector.Lower()) sector.SetChar(0,ushort(sector.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(sector,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,sector); /* Sample output: Sector: Currency */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Economy sector the symbol belongs to in the journal | //+------------------------------------------------------------------+ void SymbolSectorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSector(symbol,header_width,indent)); }
Industry type (SYMBOL_INDUSTRY)
The industry or the economy branch the symbol belongs to.
//+------------------------------------------------------------------+ //| Return the industry type or economic sector as a string | //| the symbol belongs to | //+------------------------------------------------------------------+ string SymbolIndustry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get industry type value ENUM_SYMBOL_INDUSTRY symbol_industry=(ENUM_SYMBOL_INDUSTRY)SymbolInfoInteger(symbol,SYMBOL_INDUSTRY); //--- "Cut out" the industry type from the string obtained from enum string industry=StringSubstr(EnumToString(symbol_industry),9); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(industry.Lower()) industry.SetChar(0,ushort(industry.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(industry,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,industry); /* Sample output: Industry: Undefined */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the industry type or economic sector | //| the symbol belongs to | //+------------------------------------------------------------------+ void SymbolIndustryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustry(symbol,header_width,indent)); }
Custom symbol (SYMBOL_CUSTOM)
The flag that the symbol is custom - artificially created based on other Market Watch symbols and/or external data sources.
//+------------------------------------------------------------------+ //| Return the flag | //| of a custom symbol | //+------------------------------------------------------------------+ string SymbolCustom(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Custom symbol:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_CUSTOM) ? "Yes" : "No"); /* Sample output: Custom symbol: No */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flag | //| of a custom symbol | //+------------------------------------------------------------------+ void SymbolCustomPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCustom(symbol,header_width,indent)); }
Background color in Market Watch (SYMBOL_BACKGROUND_COLOR)
The color of the background used for the symbol in Market Watch.
//+------------------------------------------------------------------+ //| Return the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ string SymbolBackgroundColor(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Background color:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the symbol background color in Market Watch color back_color=(color)SymbolInfoInteger(symbol,SYMBOL_BACKGROUND_COLOR); //--- Return the property value with a header having the required width and indentation //--- If a default color is set for a symbol (0xFF000000), return 'Default', otherwise - return its string description return StringFormat("%*s%-*s%-s",indent,"",w,header,back_color==0xFF000000 ? "Default" : ColorToString(back_color,true)); /* Sample output: Background color: Default */ }
The background color can be set to a completely transparent color, which ColorToString() returns as black (0, 0, 0). However, it is not. This is the so-called default color: 0xFF000000 — CLR_DEFAULT. It has RGB of zero, which corresponds to black, but it is completely transparent. The default color has an alpha channel that sets full transparency 0xFF000000 for the background. Therefore, before displaying the color name using ColorToString(), we check it for equality with CLR_DEFAULT.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Displays the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ void SymbolBackgroundColorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBackgroundColor(symbol,header_width,indent)); }
Price for building bars (SYMBOL_CHART_MODE)
The price type used for generating bars – Bid or Last.
//+------------------------------------------------------------------+ //| Return the price type for building bars as a string | //+------------------------------------------------------------------+ string SymbolChartMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get price type used for generating bars ENUM_SYMBOL_CHART_MODE symbol_chart_mode=(ENUM_SYMBOL_CHART_MODE)SymbolInfoInteger(symbol,SYMBOL_CHART_MODE); //--- "Cut out" price type from the string obtained from enum string chart_mode=StringSubstr(EnumToString(symbol_chart_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(chart_mode.Lower()) chart_mode.SetChar(0,ushort(chart_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Chart mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,chart_mode); /* Sample output: Chart mode: Bid */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Log the price type for building bars | //+------------------------------------------------------------------+ void SymbolChartModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolChartMode(symbol,header_width,indent)); }
Symbol presence (SYMBOL_EXIST)
Flag indicating that the symbol under this name exists.
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ string SymbolExists(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exists:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_EXIST) ? "Yes" : "No"); /* Sample output: Exists: Yes */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ void SymbolExistsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExists(symbol,header_width,indent)); }
Selected in Market Watch (SYMBOL_SELECT)
A flag indicating that the symbol is selected in Market Watch
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is selected in Market Watch | //+------------------------------------------------------------------+ string SymbolSelected(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Selected:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SELECT) ? "Yes" : "No"); /* Sample output: Selected: Yes */ }
Displays the return value of the first function in the journal:
//+--------------------------------------------------------------------------------+ //| Display the flag, that the symbol is selected in Market Watch, in the journal | //+--------------------------------------------------------------------------------+ void SymbolSelectedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSelected(symbol,header_width,indent)); }
Displayed in Market Watch (SYMBOL_VISIBLE)
Flag that the symbol is displayed in Market Watch.
Some symbols (usually cross rates, which are necessary to calculate margin requirements and profits in the deposit currency) are selected automatically, but may not be displayed in Market Watch. Such symbols should be explicitly selected to be displayed.
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ string SymbolVisible(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Visible:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_VISIBLE) ? "Yes" : "No"); /* Sample output: Visible: Yes */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ void SymbolVisiblePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVisible(symbol,header_width,indent)); }
Number of deals in the current session (SYMBOL_SESSION_DEALS)
//+------------------------------------------------------------------+ //| Return the number of deals in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionDeals(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session deals:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_DEALS)); /* Sample output: Session deals: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the number of deals in the current session in the journal| //+------------------------------------------------------------------+ void SymbolSessionDealsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionDeals(symbol,header_width,indent)); }
Number of Buy orders (SYMBOL_SESSION_BUY_ORDERS)
The total number of Buy orders at the moment
//+------------------------------------------------------------------+ //| Return the total number | //| of current buy orders as a string | //+------------------------------------------------------------------+ string SymbolSessionBuyOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Buy orders: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the total number of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrders(symbol,header_width,indent)); }
Number of Sell orders (SYMBOL_SESSION_SELL_ORDERS)
The total number of Sell orders at the moment.
//+------------------------------------------------------------------+ //| Return the total number | //| of current sell orders as a string | //+------------------------------------------------------------------+ string SymbolSessionSellOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Sell orders: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the total number of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrders(symbol,header_width,indent)); }
Last trade volume (SYMBOL_VOLUME)
Volume — last trade volume.
//+------------------------------------------------------------------+ //| Return the last trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUME)); /* Sample output: Volume: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the last trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolume(symbol,header_width,indent)); }
Maximum volume per day (SYMBOL_VOLUMEHIGH)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume high:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMEHIGH)); /* Sample output: Volume high: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHigh(symbol,header_width,indent)); }
Minimum volume per day (SYMBOL_VOLUMELOW)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMELOW)); /* Sample output: Volume low: 0 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLow(symbol,header_width,indent)); }
Last quote time (SYMBOL_TIME)
//+------------------------------------------------------------------+ //| Return the last quote time as a string | //+------------------------------------------------------------------+ string SymbolTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(string)(datetime)SymbolInfoInteger(symbol,SYMBOL_TIME)); /* Sample output: Time: 2023.07.13 21:05:12 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the last quote time in the journal | //+------------------------------------------------------------------+ void SymbolTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTime(symbol,header_width,indent)); }
Last quote time in ms (SYMBOL_TIME_MSC)
Time of the last quote in milliseconds since 1970.01.01.
//+------------------------------------------------------------------+ //| Return the last quote time as a string in milliseconds | //+------------------------------------------------------------------+ string SymbolTimeMSC(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time msc:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,TimeMSC(SymbolInfoInteger(symbol,SYMBOL_TIME_MSC))); /* Sample output: Time msc: 2023.07.13 21:09:24.327 */ }
Here we use the TimeMSC() function set at the beginning to get a date-time string with milliseconds.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the last quote time in milliseconds in the journal | //+------------------------------------------------------------------+ void SymbolTimeMSCPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTimeMSC(symbol,header_width,indent)); }
Digits (SYMBOL_DIGITS)
Number of digits after the decimal point.
//+------------------------------------------------------------------+ //| Return the number of decimal places as a string | //+------------------------------------------------------------------+ string SymbolDigits(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Digits:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_DIGITS)); /* Sample output: Digits: 5 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the number of decimal places in the journal | //+------------------------------------------------------------------+ void SymbolDigitsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDigits(symbol,header_width,indent)); }
Spread (SYMBOL_SPREAD)
Spread value in points.
//+------------------------------------------------------------------+ //| Return the spread size in points as a string | //+------------------------------------------------------------------+ string SymbolSpread(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SPREAD)); /* Sample output: Spread: 7 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the spread size in points in the journal | //+------------------------------------------------------------------+ void SymbolSpreadPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpread(symbol,header_width,indent)); }
Floating spread (SYMBOL_SPREAD_FLOAT)
An indication of the floating spread
//+------------------------------------------------------------------+ //| Return the floating spread flag as a string | //+------------------------------------------------------------------+ string SymbolSpreadFloat(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Floating Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SPREAD_FLOAT) ? "Yes" : "No"); /* Sample output: Spread float: Yes */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the floating spread flag in the journal | //+------------------------------------------------------------------+ void SymbolSpreadFloatPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpreadFloat(symbol,header_width,indent)); }
Number of requests in the market depth (SYMBOL_TICKS_BOOKDEPTH)
Maximum number of requests displayed in the Depth of Market. For instruments having no request queue, the value is 0.
//+------------------------------------------------------------------+ //| Return the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ string SymbolTicksBookDepth(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ticks book depth:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_TICKS_BOOKDEPTH)); /* Sample output: Ticks book depth: 10 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ void SymbolTicksBookDepthPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTicksBookDepth(symbol,header_width,indent)); }
Contract price calculation mode (SYMBOL_TRADE_CALC_MODE)
Contract price calculation mode.
//+------------------------------------------------------------------+ //| Return the contract price calculation mode as a string | //+------------------------------------------------------------------+ string SymbolTradeCalcMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_CALC_MODE symbol_trade_calc_mode=(ENUM_SYMBOL_CALC_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_CALC_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_calc_mode=StringSubstr(EnumToString(symbol_trade_calc_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_calc_mode.Lower()) trade_calc_mode.SetChar(0,ushort(trade_calc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(trade_calc_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade calculation mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_calc_mode); /* Sample output: Trade calculation mode: Forex */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the contract price calculation mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeCalcModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeCalcMode(symbol,header_width,indent)); }
Order execution type (SYMBOL_TRADE_MODE)
//+------------------------------------------------------------------+ //| Return the order execution type as a string | //+------------------------------------------------------------------+ string SymbolTradeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_MODE symbol_trade_mode=(ENUM_SYMBOL_TRADE_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_mode=StringSubstr(EnumToString(symbol_trade_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_mode.Lower()) trade_mode.SetChar(0,ushort(trade_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_mode); /* Sample output: Trade mode: Full */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the order execution type in the journal | //+------------------------------------------------------------------+ void SymbolTradeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeMode(symbol,header_width,indent)); }
Trading start date (SYMBOL_START_TIME)
Symbol trading start date (usually used for futures).
//+------------------------------------------------------------------+ //| Return the trading start date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolStartTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Start time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_START_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Start time: 1970.01.01 00:00:00 (Not used) */ }
If the value is not specified (equal to zero), then it is displayed as a date 1970.01.01. 00:00:00. This can be a bit confusing. Therefore, "Not used" is displayed after the date and time value in that case.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the trading start date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolStartTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolStartTime(symbol,header_width,indent)); }
Trading end date
Symbol trading end date (usually used for futures).
//+------------------------------------------------------------------+ //| Return the trading end date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolExpirationTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Expiration time: 1970.01.01 00:00:00 (Not used) */ }
If the value is not specified (equal to zero), "Not used" is displayed after the date and time value in that case.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the trading end date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolExpirationTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationTime(symbol,header_width,indent)); }
Stops Level (SYMBOL_TRADE_STOPS_LEVEL)
Minimum distance in points from the current close price for setting Stop orders.
//+-------------------------------------------------------------------------+ //| Return the minimum indentation | //| from the current close price in points to place Stop orders as a string | //+-------------------------------------------------------------------------+ string SymbolTradeStopsLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Stops level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int stops_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL); string descr=(stops_level==0 ? " (By Spread)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,stops_level,descr); /* Sample output: Stops level: 0 (By Spread) */ }
If the value is zero, this does not mean a zero level for setting stops or its absence. This means that Stop Level depends on the Spread value. Usually, 2 * Spread, which is why the explanatory entry "By Spread" is displayed.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum indentation in points from the previous | //| close price for setting Stop orders in the journal | //+------------------------------------------------------------------+ void SymbolTradeStopsLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeStopsLevel(symbol,header_width,indent)); }
Freeze Level (SYMBOL_TRADE_FREEZE_LEVEL)
Freeze distance for trading operations (in points).
//+------------------------------------------------------------------+ //| Return the distance of freezing | //| trading operations in points as a string | //+------------------------------------------------------------------+ string SymbolTradeFreezeLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Freeze level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int freeze_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_FREEZE_LEVEL); string descr=(freeze_level==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,freeze_level,descr); /* Sample output: Freeze level: 0 (Not used) */ }
If the value is zero, this means that Freeze Level is not used, which is why "Not used" text is displayed.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------------------+ //| Display the distance of freezing trading operations in points in the journal | //+------------------------------------------------------------------------------+ void SymbolTradeFreezeLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFreezeLevel(symbol,header_width,indent)); }
Trade execution mode (SYMBOL_TRADE_EXEMODE)
//+------------------------------------------------------------------+ //| Return the trade execution mode as a string | //+------------------------------------------------------------------+ string SymbolTradeExeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_EXECUTION symbol_trade_exemode=(ENUM_SYMBOL_TRADE_EXECUTION)SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_exemode=StringSubstr(EnumToString(symbol_trade_exemode),23); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_exemode.Lower()) trade_exemode.SetChar(0,ushort(trade_exemode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade Execution mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_exemode); /* Sample output: Trade Execution mode: Instant */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the trade execution mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeExeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeExeMode(symbol,header_width,indent)); }
Swap calculation model (SYMBOL_SWAP_MODE)
//+------------------------------------------------------------------+ //| Return the swap calculation model as a string | //+------------------------------------------------------------------+ string SymbolSwapMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_SYMBOL_SWAP_MODE symbol_swap_mode=(ENUM_SYMBOL_SWAP_MODE)SymbolInfoInteger(symbol,SYMBOL_SWAP_MODE); //--- "Cut out" the swap calculation model from the string obtained from enum string swap_mode=StringSubstr(EnumToString(symbol_swap_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_mode.Lower()) swap_mode.SetChar(0,ushort(swap_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(swap_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_mode); /* Sample output: Swap mode: Points */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap calculation model to the journal | //+------------------------------------------------------------------+ void SymbolSwapModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMode(symbol,header_width,indent)); }
Triple swap accrual day (SYMBOL_SWAP_ROLLOVER3DAYS)
Day of the week for calculating triple swap.
//+--------------------------------------------------------------------+ //| Return the day of the week for calculating triple swap as a string | //+--------------------------------------------------------------------+ string SymbolSwapRollover3Days(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_DAY_OF_WEEK symbol_swap_rollover3days=(ENUM_DAY_OF_WEEK)SymbolInfoInteger(symbol,SYMBOL_SWAP_ROLLOVER3DAYS); //--- Convert enum into a string with the name of the day string swap_rollover3days=EnumToString(symbol_swap_rollover3days); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_rollover3days.Lower()) swap_rollover3days.SetChar(0,ushort(swap_rollover3days.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Rollover 3 days:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_rollover3days); /* Sample output: Swap Rollover 3 days: Wednesday */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------------+ //| Display the day of the week for calculating triple swap in the journal | //+------------------------------------------------------------------------+ void SymbolSwapRollover3DaysPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapRollover3Days(symbol,header_width,indent)); }
Hedged margin calculation mode (SYMBOL_MARGIN_HEDGED_USE_LEG)
Calculating hedging margin using the larger leg (Buy or Sell).
//+------------------------------------------------------------------+ //| Return the calculation mode | //| of hedged margin using the larger leg as a string | //+------------------------------------------------------------------+ string SymbolMarginHedgedUseLeg(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged use leg:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_MARGIN_HEDGED_USE_LEG) ? "Yes" : "No"); /* Sample output: Margin hedged use leg: No */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the mode for calculating the hedged | //| margin using the larger leg in the journal | //+------------------------------------------------------------------+ void SymbolMarginHedgedUseLegPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedgedUseLeg(symbol,header_width,indent)); }
Flags of order expiration modes (SYMBOL_EXPIRATION_MODE)
If the SYMBOL_EXPIRATION_SPECIFIED flag is specified for a symbol, then, while sending a pending order, you can specifically indicate until what point this pending order is valid.
//+------------------------------------------------------------------+ //| Return the flags of allowed order expiration modes as a string | //+------------------------------------------------------------------+ string SymbolExpirationMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order expiration modes int exp_mode=(int)SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((exp_mode&SYMBOL_EXPIRATION_GTC)==SYMBOL_EXPIRATION_GTC) flags+=(flags.Length()>0 ? "|" : "")+"GTC"; if((exp_mode&SYMBOL_EXPIRATION_DAY)==SYMBOL_EXPIRATION_DAY) flags+=(flags.Length()>0 ? "|" : "")+"DAY"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED)==SYMBOL_EXPIRATION_SPECIFIED) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED_DAY)==SYMBOL_EXPIRATION_SPECIFIED_DAY) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED_DAY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Expiration mode: GTC|DAY|SPECIFIED */ }
Displays the return value of the first function in the journal:
//+--------------------------------------------------------------------+ //| Display the flags of allowed order expiration modes in the journal | //+--------------------------------------------------------------------+ void SymbolExpirationModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationMode(symbol,header_width,indent)); }
Flags of order filling modes (SYMBOL_FILLING_MODE)
Flags of allowed order filling modes.
When sending an order, we can specify the filling policy of a volume set in the order. It is possible to set several modes for each instrument via a combination of flags. The combination of flags is expressed by the logical OR (|) operation, for example SYMBOL_FILLING_FOK|SYMBOL_FILLING_IOC. The ORDER_FILLING_RETURN filling type is enabled in any execution mode except for "Market execution" (SYMBOL_TRADE_EXECUTION_MARKET).//+------------------------------------------------------------------+ //| Return the flags of allowed order filling modes as a string | //+------------------------------------------------------------------+ string SymbolFillingMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order filling modes int fill_mode=(int)SymbolInfoInteger(symbol,SYMBOL_FILLING_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Filling mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((fill_mode&ORDER_FILLING_FOK)==ORDER_FILLING_FOK) flags+=(flags.Length()>0 ? "|" : "")+"FOK"; if((fill_mode&ORDER_FILLING_IOC)==ORDER_FILLING_IOC) flags+=(flags.Length()>0 ? "|" : "")+"IOC"; if((fill_mode&ORDER_FILLING_BOC)==ORDER_FILLING_BOC) flags+=(flags.Length()>0 ? "|" : "")+"BOC"; if(SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE)!=SYMBOL_TRADE_EXECUTION_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"RETURN"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Filling mode: FOK|IOC|RETURN */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flags of allowed order filling modes in the journal | //+------------------------------------------------------------------+ void SymbolFillingModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFillingMode(symbol,header_width,indent)); }
Flags of allowed order types (SYMBOL_ORDER_MODE)
//+------------------------------------------------------------------+ //| Return flags of allowed order types as a string | //+------------------------------------------------------------------+ string SymbolOrderMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order types int order_mode=(int)SymbolInfoInteger(symbol,SYMBOL_ORDER_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse type flags into components string flags=""; if((order_mode&SYMBOL_ORDER_MARKET)==SYMBOL_ORDER_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"MARKET"; if((order_mode&SYMBOL_ORDER_LIMIT)==SYMBOL_ORDER_LIMIT) flags+=(flags.Length()>0 ? "|" : "")+"LIMIT"; if((order_mode&SYMBOL_ORDER_STOP)==SYMBOL_ORDER_STOP) flags+=(flags.Length()>0 ? "|" : "")+"STOP"; if((order_mode&SYMBOL_ORDER_STOP_LIMIT )==SYMBOL_ORDER_STOP_LIMIT ) flags+=(flags.Length()>0 ? "|" : "")+"STOP_LIMIT"; if((order_mode&SYMBOL_ORDER_SL)==SYMBOL_ORDER_SL) flags+=(flags.Length()>0 ? "|" : "")+"SL"; if((order_mode&SYMBOL_ORDER_TP)==SYMBOL_ORDER_TP) flags+=(flags.Length()>0 ? "|" : "")+"TP"; if((order_mode&SYMBOL_ORDER_CLOSEBY)==SYMBOL_ORDER_CLOSEBY) flags+=(flags.Length()>0 ? "|" : "")+"CLOSEBY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the flags of allowed order types in the journal | //+------------------------------------------------------------------+ void SymbolOrderModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderMode(symbol,header_width,indent)); }
StopLoss and TakeProfit validity periods (SYMBOL_ORDER_GTC_MODE)
Expiration of StopLoss and TakeProfit orders if SYMBOL_EXPIRATION_MODE=SYMBOL_EXPIRATION_GTC (Good till cancelled).
//+------------------------------------------------------------------+ //| Return StopLoss and TakeProfit validity periods as a string | //+------------------------------------------------------------------+ string SymbolOrderGTCMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the validity period of pending, StopLoss and TakeProfit orders ENUM_SYMBOL_ORDER_GTC_MODE symbol_order_gtc_mode=(ENUM_SYMBOL_ORDER_GTC_MODE)SymbolInfoInteger(symbol,SYMBOL_ORDER_GTC_MODE); //--- Set the validity period description as 'GTC' string gtc_mode="GTC"; //--- If the validity period is not GTC if(symbol_order_gtc_mode!=SYMBOL_ORDERS_GTC) { //--- "Cut out" pending, StopLoss and TakeProfit order validity periods from the string obtained from enum StringSubstr(EnumToString(symbol_order_gtc_mode),14); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(gtc_mode.Lower()) gtc_mode.SetChar(0,ushort(gtc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(gtc_mode,"_"," "); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order GTC mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,gtc_mode); /* Sample output: Order GTC mode: GTC */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display StopLoss and TakeProfit validity periods in the journal | //+------------------------------------------------------------------+ void SymbolOrderGTCModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderGTCMode(symbol,header_width,indent)); }
Option type (SYMBOL_OPTION_MODE)
//+------------------------------------------------------------------+ //| Return option type as a string | //+------------------------------------------------------------------+ string SymbolOptionMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the option type model ENUM_SYMBOL_OPTION_MODE symbol_option_mode=(ENUM_SYMBOL_OPTION_MODE)SymbolInfoInteger(symbol,SYMBOL_OPTION_MODE); //--- "Cut out" the option type from the string obtained from enum string option_mode=StringSubstr(EnumToString(symbol_option_mode),19); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_mode.Lower()) option_mode.SetChar(0,ushort(option_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_mode); /* Sample output: Option mode: European */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option type in the journal | //+------------------------------------------------------------------+ void SymbolOptionModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionMode(symbol,header_width,indent)); }
Option right (SYMBOL_OPTION_RIGHT)
//+------------------------------------------------------------------+ //| Return the option right as a string | //+------------------------------------------------------------------+ string SymbolOptionRight(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the option right value ENUM_SYMBOL_OPTION_RIGHT symbol_option_right=(ENUM_SYMBOL_OPTION_RIGHT)SymbolInfoInteger(symbol,SYMBOL_OPTION_RIGHT); //--- "Cut out" the option right from the string obtained from enum string option_right=StringSubstr(EnumToString(symbol_option_right),20); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_right.Lower()) option_right.SetChar(0,ushort(option_right.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_right,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option right:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_right); /* Sample output: Option right: Call */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option right in the journal | //+------------------------------------------------------------------+ void SymbolOptionRightPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionRight(symbol,header_width,indent)); }
Receiving and displaying symbol real properties ENUM_SYMBOL_INFO_DOUBLE
Bid price (SYMBOL_BID)
Bid — the best price at which an instrument can be sold.
//+------------------------------------------------------------------+ //| Return the Bid price as a string | //+------------------------------------------------------------------+ string SymbolBid(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BID)); /* Sample output: Bid: 1.31017 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Bid price in the journal | //+------------------------------------------------------------------+ void SymbolBidPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBid(symbol,header_width,indent)); }
Highest Bid price of the day (SYMBOL_BIDHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDHIGH)); /* Sample output: Bid High: 1.31422 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidHigh(symbol,header_width,indent)); }
Lowest Bid price of the day (SYMBOL_BIDLOW)
//+------------------------------------------------------------------+ //| Return the minimum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDLOW)); /* Sample output: Bid Low: 1.30934 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidLow(symbol,header_width,indent)); }
Ask price (SYMBOL_ASK)
Ask — the best price at which an instrument can be bought
//+------------------------------------------------------------------+ //| Return the Ask price as a string | //+------------------------------------------------------------------+ string SymbolAsk(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASK)); /* Sample output: Ask: 1.31060 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Ask price in the journal | //+------------------------------------------------------------------+ void SymbolAskPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAsk(symbol,header_width,indent)); }
Highest Ask price of the day (SYMBOL_ASKHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKHIGH)); /* Sample output: Ask High: 1.31427 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskHigh(symbol,header_width,indent)); }
Lowest Ask price of the day (SYMBOL_ASKLOW)
//+------------------------------------------------------------------+ //| Return the minimum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKLOW)); /* Sample output: Ask Low: 1.30938 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskLow(symbol,header_width,indent)); }
Last price (SYMBOL_LAST)
The price at which the last deal was executed.
//+------------------------------------------------------------------+ //| Return the Last price as a string | //+------------------------------------------------------------------+ string SymbolLast(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LAST)); /* Sample output: Last: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Last price in the journal | //+------------------------------------------------------------------+ void SymbolLastPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLast(symbol,header_width,indent)); }
The highest Last price of the day (SYMBOL_LASTHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTHIGH)); /* Sample output: Last High: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastHigh(symbol,header_width,indent)); }
The lowest Last price of the day (SYMBOL_LASTLOW)
//+------------------------------------------------------------------+ //| Return the minimum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTLOW)); /* Sample output: Last Low: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastLow(symbol,header_width,indent)); }
Last trade volume (SYMBOL_VOLUME_REAL)
Last executed trade volume
//+------------------------------------------------------------------+ //| Return the last executed trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUME_REAL)); /* Sample output: Volume real: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the last executed trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeReal(symbol,header_width,indent)); }
Maximum volume per day (SYMBOL_VOLUMEHIGH_REAL)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHighReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume High real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMEHIGH_REAL)); /* Sample output: Volume High real: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHighReal(symbol,header_width,indent)); }
Minimum volume per day (SYMBOL_VOLUMELOW_REAL)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLowReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume Low real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMELOW_REAL)); /* Sample output: Volume Low real: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLowReal(symbol,header_width,indent)); }
Option execution price (SYMBOL_OPTION_STRIKE)
Option strike price. This is the price at which the buyer of an option can buy (Call option) or sell (Put option) the underlying asset, while the option seller, accordingly, is obliged to sell or buy the corresponding amount of the underlying asset.
//+------------------------------------------------------------------+ //| Return the strike price as a string | //+------------------------------------------------------------------+ string SymbolOptionStrike(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option strike:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_OPTION_STRIKE)); /* Sample output: Option strike: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option strike price in the journal | //+------------------------------------------------------------------+ void SymbolOptionStrikePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionStrike(symbol,header_width,indent)); }
Point (SYMBOL_POINT)
Point value
//+------------------------------------------------------------------+ //| Return the point value as a string | //+------------------------------------------------------------------+ string SymbolPoint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Point:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_POINT)); /* Sample output: Point: 0.00001 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the point value in the journal | //+------------------------------------------------------------------+ void SymbolPointPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPoint(symbol,header_width,indent)); }
Tick price (SYMBOL_TRADE_TICK_VALUE)
SYMBOL_TRADE_TICK_VALUE_PROFIT — calculated tick value for a winning position.
//+------------------------------------------------------------------+ //| Return the tick price as a string | //+------------------------------------------------------------------+ string SymbolTradeTickValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE)); /* Sample output: Tick value: 1.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the tick price in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValue(symbol,header_width,indent)); }
Tick price for a profitable position (SYMBOL_TRADE_TICK_VALUE_PROFIT)
Calculated tick value for a profitable position.
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a profitable position | //+------------------------------------------------------------------+ string SymbolTradeTickValueProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_PROFIT)); /* Sample output: Tick value profit: 1.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a profitable position | //+------------------------------------------------------------------+ void SymbolTradeTickValueProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueProfit(symbol,header_width,indent)); }
Tick price for a losing position (SYMBOL_TRADE_TICK_VALUE_LOSS)
Calculated tick value for a losing position.
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a losing position | //+------------------------------------------------------------------+ string SymbolTradeTickValueLoss(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value loss:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_LOSS)); /* Sample output: Tick value loss: 1.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a losing position | //+------------------------------------------------------------------+ void SymbolTradeTickValueLossPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueLoss(symbol,header_width,indent)); }
Minimum price change (SYMBOL_TRADE_TICK_SIZE)
//+------------------------------------------------------------------+ //| Return the minimum price change as a string | //+------------------------------------------------------------------+ string SymbolTradeTickSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_SIZE)); /* Sample output: Tick size: 0.00001 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum price change in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickSize(symbol,header_width,indent)); }
Trade contract size (SYMBOL_TRADE_CONTRACT_SIZE)
//+------------------------------------------------------------------+ //| Return the trading contract size as a string | //+------------------------------------------------------------------+ string SymbolTradeContractSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Contract size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_CONTRACT_SIZE),SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE)); /* Sample output: Contract size: 100000.00 GBP */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the trading contract size in the journal | //+------------------------------------------------------------------+ void SymbolTradeContractSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeContractSize(symbol,header_width,indent)); }
Accrued interest (SYMBOL_TRADE_ACCRUED_INTEREST)
Accrued interest — accumulated coupon interest (part of the coupon interest calculated in proportion to the number of days since the coupon bond issuance or the last coupon interest payment).
//+------------------------------------------------------------------+ //| Return accrued interest as a string | //+------------------------------------------------------------------+ string SymbolTradeAccruedInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Accrued interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_ACCRUED_INTEREST)); /* Sample output: Accrued interest: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display accrued interest in the journal | //+------------------------------------------------------------------+ void SymbolTradeAccruedInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeAccruedInterest(symbol,header_width,indent)); }
Face value (SYMBOL_TRADE_FACE_VALUE)
Face value – initial bond value set by an issuer.
//+------------------------------------------------------------------+ //| Return the face value as a string | //+------------------------------------------------------------------+ string SymbolTradeFaceValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Face value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_FACE_VALUE)); /* Sample output: Face value: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the face value in the journal | //+------------------------------------------------------------------+ void SymbolTradeFaceValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFaceValue(symbol,header_width,indent)); }
Liquidity rate (SYMBOL_TRADE_LIQUIDITY_RATE)
Liquidity rate is the share of the asset that can be used for the margin.
//+------------------------------------------------------------------+ //| Return the liquidity rate as a string | //+------------------------------------------------------------------+ string SymbolTradeLiquidityRate(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Liquidity rate:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_LIQUIDITY_RATE)); /* Sample output: Liquidity rate: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the rate liquidity in the journal | //+------------------------------------------------------------------+ void SymbolTradeLiquidityRatePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeLiquidityRate(symbol,header_width,indent)); }
Minimum volume (SYMBOL_VOLUME_MIN)
Minimum volume for deal execution.
//+------------------------------------------------------------------+ //| Return the minimum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MIN)); /* Sample output: Volume min: 0.01 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMin(symbol,header_width,indent)); }
Maximum volume (SYMBOL_VOLUME_MAX)
Maximum volume for deal execution.
//+------------------------------------------------------------------+ //| Return the maximum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MAX)); /* Sample output: Volume max: 500.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMax(symbol,header_width,indent)); }
Volume change step (SYMBOL_VOLUME_STEP)
Minimal volume change step for deal execution.
//+------------------------------------------------------------------+ //| Return the minimum volume change step as a string | //+------------------------------------------------------------------+ string SymbolVolumeStep(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume step:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)); /* Sample output: Volume step: 0.01 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the minimum volume change step in the journal | //+------------------------------------------------------------------+ void SymbolVolumeStepPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeStep(symbol,header_width,indent)); }
Maximum volume of position and orders in one direction (SYMBOL_VOLUME_LIMIT)
The maximum allowed total volume of an open position and pending orders in one direction (either buy or sell) for this symbol. For example, if the limit is 5 lots, you may have an open position of 5 lots and place a Sell Limit order of 5 lots as well. But in this case you cannot place a pending Buy Limit order (since the total volume in one direction will exceed the limit) or place a Sell Limit order above 5 lots.
//+----------------------------------------------------------------------------+ //| Return the maximum acceptable | //| total volume of open position and pending orders for a symbol as a string | //+----------------------------------------------------------------------------+ string SymbolVolumeLimit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume limit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_LIMIT)); /* Sample output: Volume limit: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------------------+ //| Display the maximum acceptable | //| total volume of open position and pending orders for a symbol in the journal | //+------------------------------------------------------------------------------+ void SymbolVolumeLimitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLimit(symbol,header_width,indent)); }
Buy swap (SYMBOL_SWAP_LONG)
//+------------------------------------------------------------------+ //| Return the Buy swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapLong(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap long:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_LONG)); /* Sample output: Swap long: -0.20 */ }
Since the value of a property can be negative, it is necessary to shift negative property values one character to the left so that the minus sign is to the left of the column of remaining properties values in the list. To achieve this, reduce the passed field width by 1 and put a space in the format specifiers, which shifts the string to the right by one character for non-negative values. Thus, we reduce the field width by 1, while one space is added for a non-negative value leveling out the field width reduced by one character.
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Buy swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapLongPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapLong(symbol,header_width,indent)); }
Sell swap (SYMBOL_SWAP_SHORT)
//+------------------------------------------------------------------+ //| Return the Sell swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapShort(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap short:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_SHORT)); /* Sample output: Swap short: -2.20 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the Sell swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapShortPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapShort(symbol,header_width,indent)); }
Swap accrual ratio (SYMBOL_SWAP_SUNDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from SUNDAY to the next day. There following values are supported:
0 – no swap is charged
1 – single swap
3 – triple swap
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ string SymbolSwapSunday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap sunday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SUNDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap sunday: 0 (no swap is charged) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ void SymbolSwapSundayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSunday(symbol,header_width,indent)); }
Swap accrual ratio from Monday to Tuesday (SYMBOL_SWAP_MONDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from Monday to Tuesday.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ string SymbolSwapMonday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap monday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_MONDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap monday: 1 (single swap) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ void SymbolSwapMondayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMonday(symbol,header_width,indent)); }
Swap accrual ratio from Tuesday to Wednesday (SYMBOL_SWAP_TUESDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from Tuesday to Wednesday
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ string SymbolSwapTuesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Tuesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_TUESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Tuesday: 1 (single swap) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ void SymbolSwapTuesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapTuesday(symbol,header_width,indent)); }
Swap accrual ratio from Wednesday to Thursday (SYMBOL_SWAP_WEDNESDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from Wednesday to Thursday
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ string SymbolSwapWednesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Wednesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_WEDNESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Wednesday: 3 (triple swap) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ void SymbolSwapWednesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapWednesday(symbol,header_width,indent)); }
Swap accrual ratio from Thursday to Friday (SYMBOL_SWAP_THURSDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from Thursday to Friday.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ string SymbolSwapThursday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Thursday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_THURSDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Thursday: 1 (single swap) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ void SymbolSwapThursdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapThursday(symbol,header_width,indent)); }
Swap accrual ratio from Friday to Saturday (SYMBOL_SWAP_FRIDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) for overnight positions rolled over from Friday to Saturday.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ string SymbolSwapFriday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Friday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_FRIDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Friday: 1 (single swap) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ void SymbolSwapFridayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapFriday(symbol,header_width,indent)); }
Swap accrual ratio from Saturday to Sunday (SYMBOL_SWAP_SATURDAY)
Swap calculation ratio (SYMBOL_SWAP_LONG or SYMBOL_SWAP_SHORT) or overnight positions rolled over from Saturday to Sunday.
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ string SymbolSwapSaturday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Saturday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SATURDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Saturday: 0 (no swap is charged) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ void SymbolSwapSaturdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSaturday(symbol,header_width,indent)); }
In total, we have ended up with 7 * 2 functions to display the swap accrual coefficient for each day of the week. But we can make one universal function that returns a string describing the swap ratio for a specified day of the week, and one function to print the result returned by the first function to the journal. Let's add:
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| for a specified day of the week | //+------------------------------------------------------------------+ string SymbolSwapByDay(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Get a text description of a day of the week string day=EnumToString(day_week); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(day.Lower()) day.SetChar(0,ushort(day.GetChar(0)-0x20)); //--- Create the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header=StringFormat("Swap %s:",day); uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Declare the property that needs to be requested in SymbolInfoDouble() int prop=SYMBOL_SWAP_SUNDAY; //--- Depending on the day of the week, indicate the requested property switch(day_week) { case MONDAY : prop=SYMBOL_SWAP_MONDAY; break; case TUESDAY : prop=SYMBOL_SWAP_TUESDAY; break; case WEDNESDAY : prop=SYMBOL_SWAP_WEDNESDAY; break; case THURSDAY : prop=SYMBOL_SWAP_THURSDAY; break; case FRIDAY : prop=SYMBOL_SWAP_FRIDAY; break; case SATURDAY : prop=SYMBOL_SWAP_SATURDAY; break; default/*SUNDAY*/ : prop=SYMBOL_SWAP_SUNDAY; break; } //--- Get the ratio by a selected property and define its description string double swap_coeff=SymbolInfoDouble(symbol,(ENUM_SYMBOL_INFO_DOUBLE)prop); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Sunday: 0 (no swap is charged) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| for a specified day of the week | //+------------------------------------------------------------------+ void SymbolSwapByDayPrint(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapByDay(symbol,day_week,header_width,indent)); }
Initial margin (SYMBOL_MARGIN_INITIAL)
Initial margin - amount in the margin currency required for opening a position (1 lot). It is used when checking client funds during the market entry. The SymbolInfoMarginRate() function is used to obtain information about the amount of margin charged depending on the order type and direction.
//+------------------------------------------------------------------+ //| Return the initial margin as a string | //+------------------------------------------------------------------+ string SymbolMarginInitial(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin initial:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_INITIAL),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin initial: 0.00 GBP */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the initial margin in the journal | //+------------------------------------------------------------------+ void SymbolMarginInitialPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginInitial(symbol,header_width,indent)); }
Maintenance margin (SYMBOL_MARGIN_MAINTENANCE)
Maintenance margin for an instrument. If specified, it indicates the margin size in the margin currency of the instrument, withheld from one lot. It is used when checking client funds when checking the client's account status changes. If the maintenance margin is 0, then the initial margin is used. The SymbolInfoMarginRate() function is used to obtain information about the amount of margin charged depending on the order type and direction.
//+------------------------------------------------------------------+ //| Return the maintenance margin for an instrument as a string | //+------------------------------------------------------------------+ string SymbolMarginMaintenance(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin maintenance:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_MAINTENANCE),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin maintenance: 0.00 GBP */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maintenance margin for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolMarginMaintenancePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginMaintenance(symbol,header_width,indent)); }
Trade volume in the current session (SYMBOL_SESSION_VOLUME)
The total volume of trades in the current session.
//+------------------------------------------------------------------------+ //| Returns the total volume of trades in the current session as a string | //+------------------------------------------------------------------------+ string SymbolSessionVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_VOLUME)); /* Sample output: Session volume: 0.00 */ }
Displays the return value of the first function in the journal:
//+---------------------------------------------------------------------------+ //| Display the total volume of trades in the current session in the journal | //+---------------------------------------------------------------------------+ void SymbolSessionVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionVolume(symbol,header_width,indent)); }
Current session turnover (SYMBOL_SESSION_TURNOVER)
The total turnover in the current session.
//+------------------------------------------------------------------+ //| Return the total turnover in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionTurnover(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session turnover:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_TURNOVER)); /* Sample output: Session turnover: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------------+ //| Displays the total turnover during the current session in the journal | //+------------------------------------------------------------------------+ void SymbolSessionTurnoverPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionTurnover(symbol,header_width,indent)); }
Volume of open positions (SYMBOL_SESSION_INTEREST)
The total volume of open positions.
//+------------------------------------------------------------------+ //| Return the total volume of open positions as a string | //+------------------------------------------------------------------+ string SymbolSessionInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_INTEREST)); /* Sample output: Session interest: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the total volume of open positions in the journal | //+------------------------------------------------------------------+ void SymbolSessionInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionInterest(symbol,header_width,indent)); }
Volume of buy orders (SYMBOL_SESSION_BUY_ORDERS_VOLUME)
The total volume of Buy orders at the moment.
//+------------------------------------------------------------------+ //| Return the current total volume of buy orders | //| as a string | //+------------------------------------------------------------------+ string SymbolSessionBuyOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_BUY_ORDERS_VOLUME)); /* Sample output: Session Buy orders volume: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the total volume of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrdersVolume(symbol,header_width,indent)); }
Volume of sell orders (SYMBOL_SESSION_SELL_ORDERS_VOLUME)
The total volume of Sell orders at the moment.
//+------------------------------------------------------------------+ //| Return the current total volume of sell orders | //| as a string | //+------------------------------------------------------------------+ string SymbolSessionSellOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_SELL_ORDERS_VOLUME)); /* Sample output: Session Sell orders volume: 0.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the total volume of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrdersVolume(symbol,header_width,indent)); }
Session open price (SYMBOL_SESSION_OPEN)
//+------------------------------------------------------------------+ //| Return the session open price as a string | //+------------------------------------------------------------------+ string SymbolSessionOpen(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Open:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_OPEN)); /* Sample output: Session Open: 1.31314 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the session open price in the journal | //+------------------------------------------------------------------+ void SymbolSessionOpenPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionOpen(symbol,header_width,indent)); }
Session close price (SYMBOL_SESSION_CLOSE)
//+------------------------------------------------------------------+ //| Return the session close price as a string | //+------------------------------------------------------------------+ string SymbolSessionClose(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Close:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_CLOSE)); /* Sample output: Session Close: 1.31349 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the session close price in the journal | //+------------------------------------------------------------------+ void SymbolSessionClosePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionClose(symbol,header_width,indent)); }
Session average weighted price (SYMBOL_SESSION_AW)
//+------------------------------------------------------------------+ //| Return the session average weighted price as a string | //+------------------------------------------------------------------+ string SymbolSessionAW(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session AW:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_AW)); /* Sample output: Session AW: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the weighted average session price in the journal | //+------------------------------------------------------------------+ void SymbolSessionAWPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionAW(symbol,header_width,indent)); }
Settlement price of the current session (SYMBOL_SESSION_PRICE_SETTLEMENT)
//+------------------------------------------------------------------+ //| Return the settlement price of the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceSettlement(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price settlement:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_SETTLEMENT)); /* Sample output: Session price settlement: 0.00000 */ }
Displays the return value of the first function in the journal:
//+--------------------------------------------------------------------+ //| Display the settlement price of the current session in the journal | //+--------------------------------------------------------------------+ void SymbolSessionPriceSettlementPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceSettlement(symbol,header_width,indent)); }
Minimum price per session (SYMBOL_SESSION_PRICE_LIMIT_MIN)
The minimum allowable price value for the session.
//+------------------------------------------------------------------+ //| Return the minimum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MIN)); /* Sample output: Session price limit min: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Displays the minimum acceptable | //| price value per session in the journal | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMin(symbol,header_width,indent)); }
Maximum price per session (SYMBOL_SESSION_PRICE_LIMIT_MAX)
The maximum allowable price value for the session.
//+------------------------------------------------------------------+ //| Return the maximum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MAX)); /* Sample output: Session price limit max: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the maximum acceptable | //| price value per session in the journal | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMax(symbol,header_width,indent)); }
Contract size for one lot of covered positions (SYMBOL_MARGIN_HEDGED)
Size of a contract or margin for one lot of hedged positions (oppositely directed positions at one symbol). Two margin calculation methods are possible for hedged positions. The calculation method is determined by the broker.
Basic calculation:
- If the initial margin (SYMBOL_MARGIN_INITIAL) is specified for a symbol, the hedged margin is specified as an absolute value (in monetary terms).
- If the initial margin is not set (equal to 0), a contract size to be used in the margin calculation is specified in SYMBOL_MARGIN_HEDGED. The margin is calculated using the equation that corresponds to a trade symbol type (SYMBOL_TRADE_CALC_MODE).
Calculation based on the largest position:
- The SYMBOL_MARGIN_HEDGED value is ignored.
- The volume of all short and all long positions of a symbol is calculated.
- For each direction, a weighted average open price and a weighted average rate of conversion to the deposit currency is calculated.
- Next, the margin for short and long legs is calculated using the equations corresponding to a symbol type (SYMBOL_TRADE_CALC_MODE).
- The largest one of the values is used as the final value.
//+------------------------------------------------------------------+ //| Return the contract or margin size as a string | //| for a single lot of covered positions | //+------------------------------------------------------------------+ string SymbolMarginHedged(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_HEDGED)); /* Sample output: Margin hedged: 100000.00 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the contract or margin size in the journal | //| for a single lot of covered positions | //+------------------------------------------------------------------+ void SymbolMarginHedgedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedged(symbol,header_width,indent)); }
Change in current price in percentage (SYMBOL_PRICE_CHANGE)
Change of the current price relative to the end of the previous trading day in %.
//+------------------------------------------------------------------+ //| Return the change of the current price relative to | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceChange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price change:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_CHANGE)); /* Sample output: Price change: -0.28 % */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display in the journal | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ void SymbolPriceChangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceChange(symbol,header_width,indent)); }
Price volatility in % (SYMBOL_PRICE_VOLATILITY)
//+------------------------------------------------------------------+ //| Return the price volatility in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceVolatility(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price volatility:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_VOLATILITY)); /* Sample output: Price volatility: 0.00 % */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the price volatility in percentage in the journal | //+------------------------------------------------------------------+ void SymbolPriceVolatilityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVolatility(symbol,header_width,indent)); }
Theoretical option price (SYMBOL_PRICE_THEORETICAL)
//+------------------------------------------------------------------+ //| Return the theoretical price of an option as a string | //+------------------------------------------------------------------+ string SymbolPriceTheoretical(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theoretical:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THEORETICAL)); /* Sample output: Price theoretical: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the theoretical price of an option in the journal | //+------------------------------------------------------------------+ void SymbolPriceTheoreticalPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheoretical(symbol,header_width,indent)); }
Option/warrant delta (SYMBOL_PRICE_DELTA)
Option/warrant delta. Shows the value the option price changes by, when the underlying asset price changes by 1.
//+------------------------------------------------------------------+ //| Return as the option/warrant delta as a string | //+------------------------------------------------------------------+ string SymbolPriceDelta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price delta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_DELTA)); /* Sample output: Price delta: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant delta in the journal | //+------------------------------------------------------------------+ void SymbolPriceDeltaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceDelta(symbol,header_width,indent)); }
Option/warrant theta (SYMBOL_PRICE_THETA)
Option/warrant theta. Number of points the option price is to lose every day due to a temporary breakup, i.e. when the expiration date approaches.
//+------------------------------------------------------------------+ //| Return as the option/warrant theta as a string | //+------------------------------------------------------------------+ string SymbolPriceTheta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THETA)); /* Sample output: Price theta: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant theta in the journal | //+------------------------------------------------------------------+ void SymbolPriceThetaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheta(symbol,header_width,indent)); }
Option/warrant gamma (SYMBOL_PRICE_GAMMA)
Option/warrant gamma. SYMBOL_PRICE_GAMMA — option/warrant gamma.
//+------------------------------------------------------------------+ //| Return as the option/warrant gamma as a string | //+------------------------------------------------------------------+ string SymbolPriceGamma(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price gamma:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_GAMMA)); /* Sample output: Price gamma: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant gamma in the journal | //+------------------------------------------------------------------+ void SymbolPriceGammaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceGamma(symbol,header_width,indent)); }
Option/warrant vega (SYMBOL_PRICE_VEGA)
Option/warrant vega. Shows the number of points the option price changes by when the volatility changes by 1%.
//+------------------------------------------------------------------+ //| Return as the option/warrant vega as a string | //+------------------------------------------------------------------+ string SymbolPriceVega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price vega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_VEGA)); /* Sample output: Price vega: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant vega in the journal | //+------------------------------------------------------------------+ void SymbolPriceVegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVega(symbol,header_width,indent)); }
Option/warrant rho (SYMBOL_PRICE_RHO)
Option/warrant rho. Reflects the sensitivity of the theoretical option price to the interest rate changing by 1%.
//+------------------------------------------------------------------+ //| Return as the option/warrant rho as a string | //+------------------------------------------------------------------+ string SymbolPriceRho(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price rho:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_RHO)); /* Sample output: Price rho: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant rho in the journal | //+------------------------------------------------------------------+ void SymbolPriceRhoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceRho(symbol,header_width,indent)); }
Option/warrant omega (SYMBOL_PRICE_OMEGA)
Option/warrant omega. Option elasticity — a relative percentage change of the option price by the percentage change of the underlying asset price.
//+------------------------------------------------------------------+ //| Return as the option/warrant omega as a string | //+------------------------------------------------------------------+ string SymbolPriceOmega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price omega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_OMEGA)); /* Sample output: Price omega: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant omega in the journal | //+------------------------------------------------------------------+ void SymbolPriceOmegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceOmega(symbol,header_width,indent)); }
Option/warrant sensitivity (SYMBOL_PRICE_SENSITIVITY)
Option/warrant sensitivity. Shows by how many points the price of the option underlying asset should change so that the price of the option changes by one point.
//+------------------------------------------------------------------+ //| Return the option/warrant sensitivity as a string | //+------------------------------------------------------------------+ string SymbolPriceSensitivity(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price sensitivity:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_SENSITIVITY)); /* Sample output: Price sensitivity: 0.00000 */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the option/warrant sensitivity in the journal | //+------------------------------------------------------------------+ void SymbolPriceSensitivityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceSensitivity(symbol,header_width,indent)); }
Receiving and displaying symbol string properties
Underlying asset name (SYMBOL_BASIS)
The name of the underlaying asset for a derivative symbol.
//+------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument | //+------------------------------------------------------------------+ string SymbolBasis(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Basis:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BASIS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Basis: '' (Not specified) */ }
If the parameter is not specified and returns an empty string, add the "Not specified" text next to the empty parameter .
Displays the return value of the first function in the journal:
//+----------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument in the journal| //+----------------------------------------------------------------------+ void SymbolBasisPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBasis(symbol,header_width,indent)); }
Instrument category or sector (SYMBOL_CATEGORY)
The name of the category or sector the financial instrument belongs to.
//+------------------------------------------------------------------+ //| Return the name of the financial instrument category or sector | //+------------------------------------------------------------------+ string SymbolCategory(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Category:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CATEGORY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Category: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the name of the financial instrument category or sector | //| in the journal | //+------------------------------------------------------------------+ void SymbolCategoryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCategory(symbol,header_width,indent)); }
Financial instrument country (SYMBOL_COUNTRY)
Country the trading instrument belongs to.
//+------------------------------------------------------------------+ //| Return the country the trading instrument belongs to | //+------------------------------------------------------------------+ string SymbolCountry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Country:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_COUNTRY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Country: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+----------------------------------------------------------------------+ //| Display the country the trading instrument belongs to in the journal | //+----------------------------------------------------------------------+ void SymbolCountryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCountry(symbol,header_width,indent)); }
Economic sector (SYMBOL_SECTOR_NAME)
The sector of the economy to which the trading instrument belongs.
//+------------------------------------------------------------------+ //| Return the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolSectorName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_SECTOR_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Sector name: 'Currency' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display in the journal the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolSectorNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSectorName(symbol,header_width,indent)); }
Economy branch (SYMBOL_INDUSTRY_NAME)
The industry branch or the industry the financial instrument belongs to.
//+------------------------------------------------------------------+ //| Return the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolIndustryName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_INDUSTRY_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Industry name: 'Undefined' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display in the journal the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolIndustryNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustryName(symbol,header_width,indent)); }
Base currency (SYMBOL_CURRENCY_BASE)
Instrument base currency.
//+------------------------------------------------------------------+ //| Return the instrument base currency | //+------------------------------------------------------------------+ string SymbolCurrencyBase(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency base:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency base: 'GBP' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the instrument base currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyBasePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyBase(symbol,header_width,indent)); }
Profit currency (SYMBOL_CURRENCY_PROFIT)
//+------------------------------------------------------------------+ //| Return the profit currency | //+------------------------------------------------------------------+ string SymbolCurrencyProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_PROFIT); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency profit: 'USD' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the profit currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyProfit(symbol,header_width,indent)); }
Collateral currency (SYMBOL_CURRENCY_MARGIN)
Margin currency.
//+------------------------------------------------------------------+ //| Return margin currency | //+------------------------------------------------------------------+ string SymbolCurrencyMargin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency margin:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency margin: 'GBP' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the margin currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyMarginPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyMargin(symbol,header_width,indent)); }
Quote source (SYMBOL_BANK)
The source of the current quote.
//+------------------------------------------------------------------+ //| Return the current quote source | //+------------------------------------------------------------------+ string SymbolBank(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bank:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BANK); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Bank: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the current quote source in the journal | //+------------------------------------------------------------------+ void SymbolBankPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBank(symbol,header_width,indent)); }
Symbol description (SYMBOL_DESCRIPTION)
Symbol string description.
//+------------------------------------------------------------------+ //| Return the symbol string description | //+------------------------------------------------------------------+ string SymbolDescription(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Description:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_DESCRIPTION); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Description: 'Pound Sterling vs US Dollar' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the symbol string description in the journal | //+------------------------------------------------------------------+ void SymbolDescriptionPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDescription(symbol,header_width,indent)); }
Exchange name (SYMBOL_EXCHANGE)
The name of the exchange in which the security is traded.
//+------------------------------------------------------------------+ //| Return the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ string SymbolExchange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exchange:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_EXCHANGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Exchange: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display in the journal the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ void SymbolExchangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExchange(symbol,header_width,indent)); }
Custom symbol equation (SYMBOL_FORMULA)
The equation used for custom symbol pricing. If the name of a financial instrument included in the equation begins with a number or contains a special character (" ", ".", "-", "&", "#" etc.), then the name should be enclosed in quotation marks.
- Synthetic symbol: "@ESU19"/EURCAD
- Calendar spread: "Si-9.13"-"Si-6.13"
- Euro index: 34.38805726 * pow(EURUSD,0.3155) * pow(EURGBP,0.3056) * pow(EURJPY,0.1891) * pow(EURCHF,0.1113) * pow(EURSEK,0.0785)
//+------------------------------------------------------------------+ //| Return a formula for constructing a custom symbol price | //+------------------------------------------------------------------+ string SymbolFormula(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Formula:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_FORMULA); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Formula: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the equation for constructing | //| the custom symbol price in the journal | //+------------------------------------------------------------------+ void SymbolFormulaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFormula(symbol,header_width,indent)); }
ISIN system name (SYMBOL_ISIN)
The name of a trading symbol in the international system of securities identification numbers (ISIN). The International Security Identification Code is a 12-digit alphanumeric code that uniquely identifies a security. The availability of this symbol property is defined on the trade server side.
//+------------------------------------------------------------------+ //| Return the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ string SymbolISIN(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="ISIN:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_ISIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: ISIN: '' (Not specified) */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display in the journal the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ void SymbolISINPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolISIN(symbol,header_width,indent)); }
Web page address (SYMBOL_PAGE)
The address of the web page containing symbol information. This address will be displayed as a link when viewing symbol properties in the terminal.
//+------------------------------------------------------------------+ //| Return the address of a web page with a symbol data | //+------------------------------------------------------------------+ string SymbolPage(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Page:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PAGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Page: 'https://www.mql5.com/en/quotes/currencies/gbpusd' */ }
Displays the return value of the first function in the journal:
//+--------------------------------------------------------------------+ //| Display the address of a web page with a symbol data in the journal| //+--------------------------------------------------------------------+ void SymbolPagePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPage(symbol,header_width,indent)); }
Path in the symbol tree (SYMBOL_PATH)
//+------------------------------------------------------------------+ //| Return the path in the symbol tree | //+------------------------------------------------------------------+ string SymbolPath(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Path:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PATH); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Path: 'Forex\GBPUSD' */ }
Displays the return value of the first function in the journal:
//+------------------------------------------------------------------+ //| Display the path in the symbol tree in the journal | //+------------------------------------------------------------------+ void SymbolPathPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPath(symbol,header_width,indent)); }
The functions presented above are logically identical to each other. Each of them can be used "as is" to output to the journal, or to obtain the required symbol property as a string. By default, the string indent is zero and the width of the header field is equal to the width of the header text, i.e. there are no indents or alignments in the property description text. They are required if you need to display a group of symbol properties to align data fields in the journal. Let's implement such a function.
Function that prints all symbol data to the journal
Based on the created functions, we will make a function that logs all the properties of the symbol in the order they appear in the ENUM_SYMBOL_INFO_INTEGER, ENUM_SYMBOL_INFO_DOUBLE and
ENUM_SYMBOL_INFO_STRING enumerations.
//+------------------------------------------------------------------+ //| Send all symbol properties to the journal | //+------------------------------------------------------------------+ void SymbolInfoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Display descriptions of integer properties according to their location in ENUM_SYMBOL_INFO_INTEGER Print("SymbolInfoInteger properties:"); SymbolSubscriptionDelayPrint(symbol,header_width,indent); SymbolSectorPrint(symbol,header_width,indent); SymbolIndustryPrint(symbol,header_width,indent); SymbolCustomPrint(symbol,header_width,indent); SymbolBackgroundColorPrint(symbol,header_width,indent); SymbolChartModePrint(symbol,header_width,indent); SymbolExistsPrint(symbol,header_width,indent); SymbolSelectedPrint(symbol,header_width,indent); SymbolVisiblePrint(symbol,header_width,indent); SymbolSessionDealsPrint(symbol,header_width,indent); SymbolSessionBuyOrdersPrint(symbol,header_width,indent); SymbolSessionSellOrdersPrint(symbol,header_width,indent); SymbolVolumePrint(symbol,header_width,indent); SymbolVolumeHighPrint(symbol,header_width,indent); SymbolVolumeLowPrint(symbol,header_width,indent); SymbolTimePrint(symbol,header_width,indent); SymbolTimeMSCPrint(symbol,header_width,indent); SymbolDigitsPrint(symbol,header_width,indent); SymbolSpreadPrint(symbol,header_width,indent); SymbolSpreadFloatPrint(symbol,header_width,indent); SymbolTicksBookDepthPrint(symbol,header_width,indent); SymbolTradeCalcModePrint(symbol,header_width,indent); SymbolTradeModePrint(symbol,header_width,indent); SymbolSymbolStartTimePrint(symbol,header_width,indent); SymbolSymbolExpirationTimePrint(symbol,header_width,indent); SymbolTradeStopsLevelPrint(symbol,header_width,indent); SymbolTradeFreezeLevelPrint(symbol,header_width,indent); SymbolTradeExeModePrint(symbol,header_width,indent); SymbolSwapModePrint(symbol,header_width,indent); SymbolSwapRollover3DaysPrint(symbol,header_width,indent); SymbolMarginHedgedUseLegPrint(symbol,header_width,indent); SymbolExpirationModePrint(symbol,header_width,indent); SymbolFillingModePrint(symbol,header_width,indent); SymbolOrderModePrint(symbol,header_width,indent); SymbolOrderGTCModePrint(symbol,header_width,indent); SymbolOptionModePrint(symbol,header_width,indent); SymbolOptionRightPrint(symbol,header_width,indent); //--- Display descriptions of real properties according to their location in ENUM_SYMBOL_INFO_DOUBLE Print("SymbolInfoDouble properties:"); SymbolBidPrint(symbol,header_width,indent); SymbolBidHighPrint(symbol,header_width,indent); SymbolBidLowPrint(symbol,header_width,indent); SymbolAskPrint(symbol,header_width,indent); SymbolAskHighPrint(symbol,header_width,indent); SymbolAskLowPrint(symbol,header_width,indent); SymbolLastPrint(symbol,header_width,indent); SymbolLastHighPrint(symbol,header_width,indent); SymbolLastLowPrint(symbol,header_width,indent); SymbolVolumeRealPrint(symbol,header_width,indent); SymbolVolumeHighRealPrint(symbol,header_width,indent); SymbolVolumeLowRealPrint(symbol,header_width,indent); SymbolOptionStrikePrint(symbol,header_width,indent); SymbolPointPrint(symbol,header_width,indent); SymbolTradeTickValuePrint(symbol,header_width,indent); SymbolTradeTickValueProfitPrint(symbol,header_width,indent); SymbolTradeTickValueLossPrint(symbol,header_width,indent); SymbolTradeTickSizePrint(symbol,header_width,indent); SymbolTradeContractSizePrint(symbol,header_width,indent); SymbolTradeAccruedInterestPrint(symbol,header_width,indent); SymbolTradeFaceValuePrint(symbol,header_width,indent); SymbolTradeLiquidityRatePrint(symbol,header_width,indent); SymbolVolumeMinPrint(symbol,header_width,indent); SymbolVolumeMaxPrint(symbol,header_width,indent); SymbolVolumeStepPrint(symbol,header_width,indent); SymbolVolumeLimitPrint(symbol,header_width,indent); SymbolSwapLongPrint(symbol,header_width,indent); SymbolSwapShortPrint(symbol,header_width,indent); SymbolSwapByDayPrint(symbol,SUNDAY,header_width,indent); SymbolSwapByDayPrint(symbol,MONDAY,header_width,indent); SymbolSwapByDayPrint(symbol,TUESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,WEDNESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,THURSDAY,header_width,indent); SymbolSwapByDayPrint(symbol,FRIDAY,header_width,indent); SymbolSwapByDayPrint(symbol,SATURDAY,header_width,indent); SymbolMarginInitialPrint(symbol,header_width,indent); SymbolMarginMaintenancePrint(symbol,header_width,indent); SymbolSessionVolumePrint(symbol,header_width,indent); SymbolSessionTurnoverPrint(symbol,header_width,indent); SymbolSessionInterestPrint(symbol,header_width,indent); SymbolSessionBuyOrdersVolumePrint(symbol,header_width,indent); SymbolSessionSellOrdersVolumePrint(symbol,header_width,indent); SymbolSessionOpenPrint(symbol,header_width,indent); SymbolSessionClosePrint(symbol,header_width,indent); SymbolSessionAWPrint(symbol,header_width,indent); SymbolSessionPriceSettlementPrint(symbol,header_width,indent); SymbolSessionPriceLimitMinPrint(symbol,header_width,indent); SymbolSessionPriceLimitMaxPrint(symbol,header_width,indent); SymbolMarginHedgedPrint(symbol,header_width,indent); SymbolPriceChangePrint(symbol,header_width,indent); SymbolPriceVolatilityPrint(symbol,header_width,indent); SymbolPriceTheoreticalPrint(symbol,header_width,indent); SymbolPriceDeltaPrint(symbol,header_width,indent); SymbolPriceThetaPrint(symbol,header_width,indent); SymbolPriceGammaPrint(symbol,header_width,indent); SymbolPriceVegaPrint(symbol,header_width,indent); SymbolPriceRhoPrint(symbol,header_width,indent); SymbolPriceOmegaPrint(symbol,header_width,indent); SymbolPriceSensitivityPrint(symbol,header_width,indent); //--- Display descriptions of string properties according to their location in ENUM_SYMBOL_INFO_STRING Print("SymbolInfoString properties:"); SymbolBasisPrint(symbol,header_width,indent); SymbolCategoryPrint(symbol,header_width,indent); SymbolCountryPrint(symbol,header_width,indent); SymbolSectorNamePrint(symbol,header_width,indent); SymbolIndustryNamePrint(symbol,header_width,indent); SymbolCurrencyBasePrint(symbol,header_width,indent); SymbolCurrencyProfitPrint(symbol,header_width,indent); SymbolCurrencyMarginPrint(symbol,header_width,indent); SymbolBankPrint(symbol,header_width,indent); SymbolDescriptionPrint(symbol,header_width,indent); SymbolExchangePrint(symbol,header_width,indent); SymbolFormulaPrint(symbol,header_width,indent); SymbolISINPrint(symbol,header_width,indent); SymbolPagePrint(symbol,header_width,indent); SymbolPathPrint(symbol,header_width,indent); } //+------------------------------------------------------------------+
Here all the symbol properties are simply printed in the journal in a row. Property types are preceded by headers indicating the type: integer, real, and string.
The result of calling a function from a script with a header field width of 28 characters and indentation of 2 characters:
void OnStart() { //--- SymbolInfoPrint(Symbol(),28,2); /* Sample output: SymbolInfoInteger properties: Subscription Delay: No Sector: Currency Industry: Undefined Custom symbol: No Background color: Default Chart mode: Bid Exists: Yes Selected: Yes Visible: Yes Session deals: 0 Session Buy orders: 0 Session Sell orders: 0 Volume: 0 Volume high: 0 Volume low: 0 Time: 2023.07.14 22:07:01 Time msc: 2023.07.14 22:07:01.706 Digits: 5 Spread: 5 Spread float: Yes Ticks book depth: 10 Trade calculation mode: Forex Trade mode: Full Start time: 1970.01.01 00:00:00 (Not used) Expiration time: 1970.01.01 00:00:00 (Not used) Stops level: 0 (By Spread) Freeze level: 0 (Not used) Trade Execution mode: Instant Swap mode: Points Swap Rollover 3 days: Wednesday Margin hedged use leg: No Expiration mode: GTC|DAY|SPECIFIED Filling mode: FOK|IOC|RETURN Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY Order GTC mode: GTC Option mode: European Option right: Call SymbolInfoDouble properties: Bid: 1.30979 Bid High: 1.31422 Bid Low: 1.30934 Ask: 1.30984 Ask High: 1.31427 Ask Low: 1.30938 Last: 0.00000 Last High: 0.00000 Last Low: 0.00000 Volume real: 0.00 Volume High real: 0.00 Volume Low real: 0.00 Option strike: 0.00000 Point: 0.00001 Tick value: 1.00000 Tick value profit: 1.00000 Tick value loss: 1.00000 Tick size: 0.00001 Contract size: 100000.00 GBP Accrued interest: 0.00 Face value: 0.00 Liquidity rate: 0.00 Volume min: 0.01 Volume max: 500.00 Volume step: 0.01 Volume limit: 0.00 Swap long: -0.20 Swap short: -2.20 Swap Sunday: 0 (no swap is charged) Swap Monday: 1 (single swap) Swap Tuesday: 1 (single swap) Swap Wednesday: 3 (triple swap) Swap Thursday: 1 (single swap) Swap Friday: 1 (single swap) Swap Saturday: 0 (no swap is charged) Margin initial: 0.00 GBP Margin maintenance: 0.00 GBP Session volume: 0.00 Session turnover: 0.00 Session interest: 0.00 Session Buy orders volume: 0.00 Session Sell orders volume: 0.00 Session Open: 1.31314 Session Close: 1.31349 Session AW: 0.00000 Session price settlement: 0.00000 Session price limit min: 0.00000 Session price limit max: 0.00000 Margin hedged: 100000.00 Price change: -0.28 % Price volatility: 0.00 % Price theoretical: 0.00000 Price delta: 0.00000 Price theta: 0.00000 Price gamma: 0.00000 Price vega: 0.00000 Price rho: 0.00000 Price omega: 0.00000 Price sensitivity: 0.00000 SymbolInfoString properties: Basis: '' (Not specified) Category: '' (Not specified) Country: '' (Not specified) Sector name: 'Currency' Industry name: 'Undefined' Currency base: 'GBP' Currency profit: 'USD' Currency margin: 'GBP' Bank: '' (Not specified) Description: 'Pound Sterling vs US Dollar' Exchange: '' (Not specified) Formula: '' (Not specified) ISIN: '' (Not specified) Page: 'https://www.mql5.com/en/quotes/currencies/gbpusd' Path: 'Forex\GBPUSD' */ }
As we can see, all the data is neatly arranged in tabular form. Negative values do not stand out from the overall picture due to their shift to the left.
This is just an example of how the functions described above can be used. You can use them "as is" in your programs, display some groups of symbol properties or modify them to suit your vision and needs.
Conclusion
We have considered the functions for displaying symbol and account properties using formatted strings. The next step is to log some structures implemented in MQL5.
Translated from Russian by MetaQuotes Ltd.
Original article: https://www.mql5.com/ru/articles/12953





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use