Hey all, I am writing an EA that uses the Dialog class to display a nice floating window for the user to interact with. Problem is whenever the time frame is changed the panel bugs out creating artifacts on the chart. When testing the basic dialog code(below) I get a failed to initialize message which removes the EA and crashes the chart. When running my code, the panel scatters randomly across my chart leaving ghost images on the chart of the buttons and panel. If you find the real buttons the EA still functions correctly. When that happens I don't get the failed initialize message or any errors. It also seems to break the second time I change time frames not the first in both cases.
Here is an image of what happens to my EA after loading on the 1hour and changing time frames 3 times. The Expert log shows each time change and no errors:
Now when I switch timeframes the EA destroy()s the window in uninit giving "reason 3"(meaning period was changed) and then re-initializes but I can't tell what is going wrong in between that. All the create fuctions in OnInit have failure modes but I never get any indication of failures just a bugged EA. Is there any way to tell what is breaking, or has anyone used this class before and encountered this issue?
Now the sample code(below) returns the error "failed to initialize" (reason 8) when it crashes but I still don't know why it is failing to create the dialog on the second attempt to change time frames and not the first.
If you want to try it yourself this is the sample code for the simple panel that comes with MT4. I moved PanelDialog.mqh to the Experts folder so it can find it for this test and removed the on calc expression so it can run as an EA.
Hi, I solved the problem by not destroying the dialog on uninit reason 3 (symbol and chart change) and not creating the dialog if a part of it was found on the chart.
//| SimplePanel.mq4 |
//| Copyright 2009-2014, MetaQuotes Software Corp. |
//| https://www.mql4.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2009-2014, MetaQuotes Software Corp."
#property link "https://www.mql4.com"
#property version "1.00"
#property strict
#include "PanelDialog.mqh"
//+------------------------------------------------------------------+
//| Global Variables |
//+------------------------------------------------------------------+
CPanelDialog ExtDialog;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit(void)
{
if (ObjectFind(chart_id,"name_of_any_part_of_the_dialog")>-1)
return(INIT_SUCCEEDED);
//--- create application dialog
if(!ExtDialog.Create(0,"Title",0,50,50,390,200))
return(INIT_FAILED);
//--- run application
if(!ExtDialog.Run())
return(INIT_FAILED);
//--- ok
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//--- destroy application dialog
if (reason != 3)
ExtDialog.Destroy(reason);
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
ExtDialog.ChartEvent(id,lparam,dparam,sparam);
}
//+---------------------------------------------------------
I have same error with my panel when i've change ea parameters (F7)
Here you can find a fix as i did
at repy:
honest_knave 2017.04.07 13:43 #4
https://www.mql5.com/en/forum/189473
just edit as mql5
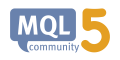
- 2017.04.05
- www.mql5.com
I just found this problem on my EA.
So, at the OnDeinit, it will not destroy the dashboard by change time frame :
void OnDeinit(const int reason) { //--- if(reason!=REASON_CHARTCHANGE) ExtDialog.Destroy(reason); }
At the OnInit, it will re-create the dashboard.
Then it should get all the existing value on the dashboard.
int OnInit() { //--- for(int i=ObjectsTotal()-1; i>=0; i--) { string objname = ObjectName(i); string text = ObjectGetString(0,objname,OBJPROP_TEXT); //find the text of the object if(text==ea_name) { ExtDialog.GetInputValue(); //get all input value if object exist return(INIT_SUCCEEDED); } } if(!ExtDialog.Create(0,ea_name,0,20,20,280,340)) { MessageBox("Sorry, the panel can't be created.","Panel not created"); return(INIT_FAILED); } ExtDialog.Run(); //--- return(INIT_SUCCEEDED); }
It is working good.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hey all, I am writing an EA that uses the Dialog class to display a nice floating window for the user to interact with. Problem is whenever the time frame is changed the panel bugs out creating artifacts on the chart. When testing the basic dialog code(below) I get a failed to initialize message which removes the EA and crashes the chart. When running my code, the panel scatters randomly across my chart leaving ghost images on the chart of the buttons and panel. If you find the real buttons the EA still functions correctly. When that happens I don't get the failed initialize message or any errors. It also seems to break the second time I change time frames not the first in both cases.
Here is an image of what happens to my EA after loading on the 1hour and changing time frames 3 times. The Expert log shows each time change and no errors:
Now when I switch timeframes the EA destroy()s the window in uninit giving "reason 3"(meaning period was changed) and then re-initializes but I can't tell what is going wrong in between that. All the create fuctions in OnInit have failure modes but I never get any indication of failures just a bugged EA. Is there any way to tell what is breaking, or has anyone used this class before and encountered this issue?
Now the sample code(below) returns the error "failed to initialize" (reason 8) when it crashes but I still don't know why it is failing to create the dialog on the second attempt to change time frames and not the first.
If you want to try it yourself this is the sample code for the simple panel that comes with MT4. I moved PanelDialog.mqh to the Experts folder so it can find it for this test and removed the on calc expression so it can run as an EA.