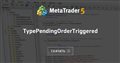
I have the following case: I want when a pending limit order is canceled or when it is executed the EA gives me the information of this order. I tried the following, but it didn't work:
The problem is that 'Select' is not selecting any order and keeps returning false. Can anybody help me?
I tried the code below too but it didn't work...
//+------------------------------------------------------------------+ //| abcd.mq5 | //| Anderson | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Anderson" #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Includes | //+------------------------------------------------------------------+ #include <Trade\HistoryOrderInfo.mqh> //+------------------------------------------------------------------+ //| Globals | //+------------------------------------------------------------------+ CHistoryOrderInfo myhistory; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction& trans, const MqlTradeRequest& request, const MqlTradeResult& result) { myhistory.Ticket(result.order); ulong numberOrder = myhistory.Ticket(); if(numberOrder) { datetime entry_datetime = m_order.TimeSetup(); // ... Print("ORDER DATETIME -> ", entry_datetime ); } }
If you need to track the triggering of a pending order, I use the following code: TypePendingOrderTriggered
Thanks for the feedback! What I want is that when OnTradeTransaction() generates a transaction it does that check -> m_order.Select(result.order) -> give true
I read and understood your code, but this is not my application. I'll try to explain it again: I want that when an order is filled or when an order is cancelled, generate a history with the order information. For example, for a canceled order to show me when it was cancelled, what the opening price was, etc.
I don't care about pending orders information.
//+------------------------------------------------------------------+ //| abcd.mq5 | //| Anderson | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Anderson" #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Includes | //+------------------------------------------------------------------+ #include <Trade\OrderInfo.mqh> //+------------------------------------------------------------------+ //| Globals | //+------------------------------------------------------------------+ COrderInfo m_order; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction& trans, const MqlTradeRequest& request, const MqlTradeResult& result) { if(trans.type == TRADE_TRANSACTION_ORDER_DELETE) { m_order.Select(request.order); Print("CANCEL ", m_order.Select(request.order)); // <<<--- FALSE !!!! } } //+------------------------------------------------------------------+
I read and understood your code, but this is not my application. I'll try to explain it again: I want that when an order is filled or when an order is cancelled, generate a history with the order information. For example, for a canceled order to show me when it was cancelled, what the opening price was, etc.
I don't care about pending orders information.
You need to do the following: You must take and use my code. I didn’t just write protection in my code - I protected myself from possible errors. If a transaction has passed, this is not yet a guarantee that the order is recorded in the history - since THIS IS AN EVENT model.
You need to do the following: You must take and use my code. I didn’t just write protection in my code - I protected myself from possible errors. If a transaction has passed, this is not yet a guarantee that the order is recorded in the history - since THIS IS AN EVENT model.
I use your structure as you insisted; but what is important to me is not returning. I wanted m_order.Select() to return true, which was the chosen order, but it didn't work...
//+------------------------------------------------------------------+ //| TypePendingOrderTriggered.mq5 | //| Copyright © 2017, Vladimir Karputov | //| http://wmua.ru/slesar/ | //+------------------------------------------------------------------+ #property copyright "Copyright © 2017, Vladimir Karputov" #property link "http://wmua.ru/slesar/" #property description "What type of a pending order triggered?" #property version "1.001" #include <Trade\OrderInfo.mqh> COrderInfo m_order; //--- bool bln_find_order=false; // true -> you should look for a order ulong ul_find_order=0; // order ticket //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- if(bln_find_order) // true -> you should look for a order { static long counter=0; Print("Attempt number ",counter); ResetLastError(); if(HistoryOrderSelect(ul_find_order)) { long type_order=HistoryOrderGetInteger(ul_find_order,ORDER_TYPE); if(type_order==ORDER_TYPE_BUY_LIMIT || type_order==ORDER_TYPE_BUY_STOP || type_order==ORDER_TYPE_SELL_LIMIT ||type_order==ORDER_TYPE_SELL_STOP) { Print("The pending order ",ul_find_order," is found! Type of order is ", EnumToString((ENUM_ORDER_TYPE)HistoryOrderGetInteger(ul_find_order,ORDER_TYPE))); Print("ORDER SELECTED? ", m_order.Select(ul_find_order)); // <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<< IS RETURN FALSE bln_find_order=false; // true -> you should look for a order counter=0; return; } else { Print("The order ",ul_find_order," is not pending"); Print("ORDER SELECTED? ", m_order.Select(ul_find_order)); // <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<< IS RETURN FALSE bln_find_order=false; // true -> you should look for a order return; } } else { Print("Order ",ul_find_order," is not find, error#",GetLastError()); } counter++; } } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //+------------------------------------------------------------------+ //| TRADE_TRANSACTION_DEAL_* | //| The following fields in MqlTradeTransaction structure | //| are filled for trade transactions related to deals handling | //| (TRADE_TRANSACTION_DEAL_ADD, TRADE_TRANSACTION_DEAL_UPDATE | //| and TRADE_TRANSACTION_DEAL_DELETE): | //| •deal - deal ticket; | //| •order - order ticket, based on which a deal has been performed;| //| •symbol - deal symbol name; | //| •type - trade transaction type; | //| •deal_type - deal type; | //| •price - deal price; | //| •price_sl - Stop Loss price (filled, if specified in the order, | //| •based on which a deal has been performed); | //| •price_tp - Take Profit price (filled, if specified | //| in the order, based on which a deal has been performed); | //| •volume - deal volume in lots. | //| •position - the ticket of the position that was opened, | //| modified or closed as a result of deal execution. | //| •position_by - the ticket of the opposite position. | //| It is only filled for the out by deals | //| (closing a position by an opposite one). | //+------------------------------------------------------------------+ //--- get transaction type as enumeration value ENUM_TRADE_TRANSACTION_TYPE type=trans.type; //--- if transaction is result of addition of the transaction in history if(type==TRADE_TRANSACTION_DEAL_ADD) { bln_find_order=true; // true -> you should look for a order ul_find_order=trans.order; } } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+
I need to use this class because I need the following fields: TimeSetup(), TimeDone(), StateDescription()
Read and study the DOCUMENTATION! You access the trading history and then try to CHOOSE A PENDING ORDER!!! - this is a gross mistake and a demonstration of a complete misunderstanding of the main trading terms:
"pending order", "order" (trading order), "deal", "position".
Read here: Basic Principles
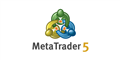
- www.metatrader5.com
Here is the modified code:
//+------------------------------------------------------------------+ //| TypePendingOrderTriggered.mq5 | //| Copyright © 2017-2021, Vladimir Karputov | //| https://www.mql5.com/en/users/barabashkakvn | //+------------------------------------------------------------------+ #property copyright "Copyright © 2017-2021, Vladimir Karputov" #property link "https://www.mql5.com/en/users/barabashkakvn" #property version "1.003" #property description "What type of a pending order triggered?" //--- bool bln_find_order=false; // true -> you should look for a order ulong ul_find_order=0; // order ticket //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- if(bln_find_order) // true -> you should look for a order { static long counter=0; PrintFormat("Attempt %d, looking for order number %",counter,ul_find_order); ResetLastError(); if(HistoryOrderSelect(ul_find_order)) { //--- long o_ticket =HistoryOrderGetInteger(ul_find_order,ORDER_TICKET); long o_time_setup =HistoryOrderGetInteger(ul_find_order,ORDER_TIME_SETUP); long o_type =HistoryOrderGetInteger(ul_find_order,ORDER_TYPE); long o_state =HistoryOrderGetInteger(ul_find_order,ORDER_STATE); long o_time_expiration =HistoryOrderGetInteger(ul_find_order,ORDER_TIME_EXPIRATION); long o_time_done =HistoryOrderGetInteger(ul_find_order,ORDER_TIME_DONE); long o_time_setup_msc =HistoryOrderGetInteger(ul_find_order,ORDER_TIME_SETUP_MSC); long o_time_done_msc =HistoryOrderGetInteger(ul_find_order,ORDER_TIME_DONE_MSC); long o_type_filling =HistoryOrderGetInteger(ul_find_order,ORDER_TYPE_FILLING); long o_type_time =HistoryOrderGetInteger(ul_find_order,ORDER_TYPE_TIME); long o_magic =HistoryOrderGetInteger(ul_find_order,ORDER_MAGIC); long o_reason =HistoryOrderGetInteger(ul_find_order,ORDER_REASON); long o_position_id =HistoryOrderGetInteger(ul_find_order,ORDER_POSITION_ID); long o_position_by_id =HistoryOrderGetInteger(ul_find_order,ORDER_POSITION_BY_ID); double o_volume_initial =HistoryOrderGetDouble(ul_find_order,ORDER_VOLUME_INITIAL); double o_volume_current =HistoryOrderGetDouble(ul_find_order,ORDER_VOLUME_CURRENT); double o_open_price =HistoryOrderGetDouble(ul_find_order,ORDER_PRICE_OPEN); double o_sl =HistoryOrderGetDouble(ul_find_order,ORDER_SL); double o_tp =HistoryOrderGetDouble(ul_find_order,ORDER_TP); double o_price_current =HistoryOrderGetDouble(ul_find_order,ORDER_PRICE_CURRENT); double o_price_stoplimit =HistoryOrderGetDouble(ul_find_order,ORDER_PRICE_STOPLIMIT); string o_symbol =HistoryOrderGetString(ul_find_order,ORDER_SYMBOL); string o_comment =HistoryOrderGetString(ul_find_order,ORDER_COMMENT); string o_extarnal_id =HistoryOrderGetString(ul_find_order,ORDER_EXTERNAL_ID); string str_o_time_setup =TimeToString((datetime)o_time_setup,TIME_DATE|TIME_MINUTES|TIME_SECONDS); string str_o_type =EnumToString((ENUM_ORDER_TYPE)o_type); string str_o_state =EnumToString((ENUM_ORDER_STATE)o_state); string str_o_time_expiration =TimeToString((datetime)o_time_expiration,TIME_DATE|TIME_MINUTES|TIME_SECONDS); string str_o_time_done =TimeToString((datetime)o_time_done,TIME_DATE|TIME_MINUTES|TIME_SECONDS); string str_o_type_filling =EnumToString((ENUM_ORDER_TYPE_FILLING)o_type_filling); string str_o_type_time =TimeToString((datetime)o_type_time,TIME_DATE|TIME_MINUTES|TIME_SECONDS); string str_o_reason =EnumToString((ENUM_ORDER_REASON)o_reason); //--- long digits=5; if(SymbolSelect(o_symbol,true)) digits=SymbolInfoInteger(o_symbol,SYMBOL_DIGITS); //--- string text=""; //--- text="Order:"; OutputTest(text); text=StringFormat("%-20s %-20s %-20s %-20s %-20s %-20s %-20s %-20s %-20s", "|Ticket","|Time setup","|Type","|State","|Time expiration", "|Time done","|Time setup msc","|Time done msc","|Type filling"); OutputTest(text); text=StringFormat("|%-19d |%-19s |%-19s |%-19s |%-19s |%-19s |%-19I64d |%-19I64d |%-19s", o_ticket,str_o_time_setup,str_o_type,str_o_state,str_o_time_expiration,str_o_time_done, o_time_setup_msc,o_time_done_msc,str_o_type_filling); OutputTest(text); text=StringFormat("%-20s %-20s %-20s %-20s %-20s", "|Type time","|Magic","|Reason","|Position id","|Position by id"); OutputTest(text); text=StringFormat("|%-19s |%-19d |%-19s |%-19d |%-19d", str_o_type_time,o_magic,str_o_reason,o_position_id,o_position_by_id); OutputTest(text); text=StringFormat("%-20s %-20s %-20s %-20s %-20s %-20s %-20s", "|Volume initial","|Volume current","|Open price","|sl","|tp","|Price current","|Price stoplimit"); OutputTest(text); text=StringFormat("|%-19.2f |%-19.2f |%-19."+IntegerToString(digits)+"f |%-19."+IntegerToString(digits)+ "f |%-19."+IntegerToString(digits)+"f |%-19."+IntegerToString(digits)+ "f |%-19."+IntegerToString(digits)+"f", o_volume_initial,o_volume_current,o_open_price,o_sl,o_tp,o_price_current,o_price_stoplimit); OutputTest(text); text=StringFormat("%-20s %-41s %-20s","|Symbol","|Comment","|External id"); OutputTest(text); text=StringFormat("|%-19s |%-40s |%-19s",o_symbol,o_comment,o_extarnal_id); OutputTest(text); //--- long type_order=HistoryOrderGetInteger(ul_find_order,ORDER_TYPE); if(type_order==ORDER_TYPE_BUY_LIMIT || type_order==ORDER_TYPE_BUY_STOP || type_order==ORDER_TYPE_SELL_LIMIT ||type_order==ORDER_TYPE_SELL_STOP) { Print("The pending order ",ul_find_order," is found! Type of order is ", EnumToString((ENUM_ORDER_TYPE)HistoryOrderGetInteger(ul_find_order,ORDER_TYPE))); bln_find_order=false; // true -> you should look for a order counter=0; return; } else { Print("The order ",ul_find_order," is not pending"); bln_find_order=false; // true -> you should look for a order return; } } else { Print("Order ",ul_find_order," is not find, error #",GetLastError()); } counter++; } } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //+------------------------------------------------------------------+ //| TRADE_TRANSACTION_DEAL_* | //| The following fields in MqlTradeTransaction structure | //| are filled for trade transactions related to deals handling | //| (TRADE_TRANSACTION_DEAL_ADD, TRADE_TRANSACTION_DEAL_UPDATE | //| and TRADE_TRANSACTION_DEAL_DELETE): | //| •deal - deal ticket; | //| •order - order ticket, based on which a deal has been performed;| //| •symbol - deal symbol name; | //| •type - trade transaction type; | //| •deal_type - deal type; | //| •price - deal price; | //| •price_sl - Stop Loss price (filled, if specified in the order, | //| •based on which a deal has been performed); | //| •price_tp - Take Profit price (filled, if specified | //| in the order, based on which a deal has been performed); | //| •volume - deal volume in lots. | //| •position - the ticket of the position that was opened, | //| modified or closed as a result of deal execution. | //| •position_by - the ticket of the opposite position. | //| It is only filled for the out by deals | //| (closing a position by an opposite one). | //+------------------------------------------------------------------+ //--- get transaction type as enumeration value ENUM_TRADE_TRANSACTION_TYPE type=trans.type; //--- if transaction is result of addition of the transaction in history if(type==TRADE_TRANSACTION_DEAL_ADD) { bln_find_order=true; // true -> you should look for a order ul_find_order=trans.order; } } //+------------------------------------------------------------------+ //| Output text | //+------------------------------------------------------------------+ void OutputTest(const string text) { Print(text); } //+------------------------------------------------------------------+
and the result of triggering the PENDING ORDER 'Buy limit':
Order: |Ticket |Time setup |Type |State |Time expiration |Time done |Time setup msc |Time done msc |Type filling |1289692469 |2022.03.23 05:55:44 |ORDER_TYPE_BUY_LIMIT |ORDER_STATE_FILLED |1970.01.01 00:00:00 |2022.03.23 05:56:45 |1648014944865 |1648015005302 |ORDER_FILLING_RETURN |Type time |Magic |Reason |Position id |Position by id |1970.01.01 00:00:00 |0 |ORDER_REASON_CLIENT |1289692469 |0 |Volume initial |Volume current |Open price |sl |tp |Price current |Price stoplimit |0.01 |0.00 |0.93799 |0.00000 |0.00000 |0.93799 |0.00000 |Symbol |Comment |External id |AUDCAD | | The pending order 1289692469 is found! Type of order is ORDER_TYPE_BUY_LIMIT

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have the following case: I want when a pending limit order is canceled or when it is executed the EA gives me the information of this order. I tried the following, but it didn't work:
The problem is that 'Select' is not selecting any order and keeps returning false. Can anybody help me?