Not sure I understand. Is it not what I am doing? Filling up the first array using iDeMarker and then computing the iMAOnArray?
the iMaOnArray is the moving Average on an array
where your Array in your case is the Demarker
This is what the code already does. Isn't it?
//--- main loops 1 and 2 for(i=0; i < limit; i++) { DMBuffer[i]=NormalizeDouble(iDeMarker(Symbol(),0,DMPeriod,i),4); } for(i=0; i < limit; i++) { ExtDMBuffer[i]=iMAOnArray(DMBuffer,0,MAPeriod,0,3,i); } //----
//+------------------------------------------------------------------+ //| demarker Moving Average.mq4 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict #property indicator_separate_window #property indicator_buffers 2 #property indicator_plots 1 //--- plot ExtDM #property indicator_label1 "MAofDM" //Please Note same as Short name #property indicator_type1 DRAW_LINE #property indicator_color1 clrAqua #property indicator_style1 STYLE_DOT #property indicator_width1 1 //--- input parameters input int DMPeriod=30; input int MAPeriod=30; //--- indicator buffers double ExtDMBuffer[]; double DMBuffer[]; //---Copy and Paste //---- input parameters int ExtDMPeriod=100; int ExtDMShift=0; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,ExtDMBuffer); SetIndexBuffer(1,DMBuffer,INDICATOR_CALCULATIONS); //---copy and paste SetIndexShift(0,ExtDMShift); //--- set indexing for the buffer like in timeseries ArraySetAsSeries(DMBuffer,true); //---- name for DataWindow and indicator subwindow label //---- first values aren't drawn SetIndexDrawBegin(0,ExtDMPeriod); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int limit; if(prev_calculated == 0) limit = rates_total-1; else limit = rates_total - prev_calculated; //--- main loops 1 and 2 for(int i=0; i < limit; i++) { DMBuffer[i]=NormalizeDouble(iDeMarker(Symbol(),0,DMPeriod,i),4); } for(int i=0; i < limit; i++) { ExtDMBuffer[i]=iMAOnArray(DMBuffer,0,MAPeriod,0,3,i); } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
Never tested just wrote.
if it dont compile just fix the errors maybe 1 or 2 errors
I'm confused as well.
I can't figure out what point you are trying to make.
just wrote the code using updated Syntax,
just upgrade ur mql4
Works well.
just wrote the code using updated Syntax,
just upgrade ur mql4
Works well.
The code works perfectly and I definitively have to upgrade my mql4.
Thanks for your help
The code works perfectly and I definitively have to upgrade my mql4.
Thanks for your help
First, the code only works if I predefine the DMBuffer array size (i.e. double DMBuffer[3000];). Simply declaring "double DMBuffer[];" I have no values. Why is that? How can I fix it?
Because DMBuffer is not Initialized as a buffer.
//--- indicator buffers mapping SetIndexBuffer(0,ExtDMBuffer); SetIndexBuffer(1,DMBuffer,INDICATOR_CALCULATIONS); //Here It was Initialised
Second, calling the custom indicator in the EA via " MAofDMBuffer=iCustom(NULL,0,"MAofDM",30,30,0,1);" (note that I need exclusively the value of the previous candle) only gives me MAofDMBuffer= 2147483647.0
Please use the PrintFormat function correctly.
try to use Print and see the Value
HERE I
asked a similar question
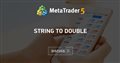
- 2019.12.05
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello All,
I want to use the moving average values of the DeMarker indicator in one EA I created. Reading some of the posts available I saw that
the best option to go about that is to create first such and indicator and then call it via iCustom in your expert. So I created the following code:
The code does what is supposed to do but I have two issues. First, the code only works if I predefine the DMBuffer array size (i.e. double DMBuffer[3000];). Simply declaring "double DMBuffer[];" I have no values. Why is that? How can I fix it?
Second, calling the custom indicator in the EA via " MAofDMBuffer=iCustom(NULL,0,"MAofDM",30,30,0,1);" (note that I need exclusively the value of the previous candle) only gives me MAofDMBuffer= 2147483647.0 (EMPTY VALUE) at every new candle. However, if I test the same command using a script it works just fine. Might this second issue be related to the first one?
Thanks for any help