Even if it is on Linux, it is still running under Wine, so why not just use DLL calls to the WinAPI to build windows and panels?
You could even create your own DLL's in C/C++ for more sophisticated solutions.
I am assuming this is for your own use and not for sale in the Market, so DLL calls should not be an issue.
Wow. Am I really this far behind? Just found a superb article that may be precisely what I'm looking for.
Why create a .json file ('socket') when all one needs to do is to Post a webrequest? Going to attempt this as an approach.
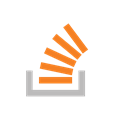
- 2016.10.10
- koul koul 461 3 3 gold badges 10 10 silver badges 20 20 bronze badges
- stackoverflow.com
Even if it is on Linux, it is still running under Wine, so why not just use DLL calls to the WinAPI to build windows and panels?
You could even create your own DLL's in C/C++ for more sophisticated solutions.
I am assuming this is for your own use and not for sale in the Market, so DLL calls should not be an issue.
This is true - MT4 running under wine. I wanted to go with with DLL's initially - but don't have enough samples to find a starting point. Most of the samples are windows based using the dreaded .net framework.
That said, even though my project is personally developed - my source is public on github. I've been working with MT4 since 2008 off and on - now, full time. If you know of anyone willing to lend a hand, please do offer.
There are not that many examples of DLL written C/C++ for MQL, because they are not much different from any other DLL usage for normal C/C++ applications.
The available examples are enough to show that you can just write them is if you were coding for a pure Windows application.
However, here is an example with Linux in mind ...
Develop a Proof-of-Concept DLL with C++ multi-threading support for MetaTrader 5 on Linux
Wasin Thonkaew, 2023.01.27 16:22
We will begin the journey to explore the steps and workflow on how to base development for MetaTrader 5 platform solely on Linux system in which the final product works seamlessly on both Windows and Linux system. We will get to know Wine, and Mingw; both are the essential tools to make cross-platform development works. Especially Mingw for its threading implementations (POSIX, and Win32) that we need to consider in choosing which one to go with. We then build a proof-of-concept DLL and consume it in MQL5 code, finally compare the performance of both threading implementations. All for your foundation to expand further on your own. You should be comfortable building MT related tools on Linux after reading this article.
There are not that many examples of DLL written C/C++ for MQL, because they are not much different from any other DLL usage for normal C/C++ applications.
The available examples are enough to show that you can just write them is if you were coding for a pure Windows application.
However, here is an example with Linux in mind ...
Going to give this another shot - followed the steps to this point -
There are a *lot* of .h files in a *lot* of directories pulled from the git clone. This pic shows a *very* short list...:
Therefore, a cp command doesn't get us there - but rather a tarball especially if all the sub dirs are relative. I'm willing to go down this rabbit hole but need a little more grounding.
Going to give this another shot - followed the steps to this point -
There are a *lot* of .h files in a *lot* of directories pulled from the git clone. This pic shows a *very* short list...:
Therefore, a cp command doesn't get us there - but rather a tarball especially if all the sub dirs are relative. I'm willing to go down this rabbit hole but need a little more grounding.
LOL! Figured this out *finally*. It was always in the linker ..
First, I started by downloading a pure windows-based 32bit gcc compiler (TDM-GCC-32) - this may not be needed as long as long as gcc multilib is installed; (to use the gcc multilib, add the params -m32 $* to your cli compile opts)
Having successfully compiled the dll (i.e., 'error-free'), for 'some odd reason' the EA was unable to import the exported functions.
wine g++.exe -v -shared -fdiagnostics-color=always -g "$MT4_HOME/Scripts/Examples/DLL/DLLSampleTest.cpp" -o "$MT4_HOME/Scripts/Examples/DLL/DLLSampleTest.dll"
So, I downloaded the dependency walker and saw that all of the function exports had an ordinal attached (below). The exported function names attached an ordinal ('@NN') - which is why the .def file was supplied with the sample.
Not wanting to use a static .def file, after many hours, I stumbled into upon how to list the linker options and found the '--kill-at' linker option.
wine g++.exe -v -shared -fdiagnostics-color=always -g "$MT4_HOME/Scripts/Examples/DLL/DLLSampleTest.cpp" -o "$MT4_HOME/Scripts/Examples/DLL/DLLSampleTest.dll" -Wl,--dll,--compat-implib,--kill-at
Compile worked, error free and all of the attached ordinals were removed.
Then, the rerun:
Success!
*** Edit: One Other thing - very important ***
I added 'extern "C"' to the MT4_EXPFUNC #define...

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have fiddled about looking for design concepts to implement visuals of the data collection, aggregation, et al (pics below). As much as I love the MT4/5 platform, visuals are not its strong point. First, real estate is expensive; Second, I/O overhead on graphics rendering is high - the more objects added, the higher the performance loss.
So, I'm looking for solutions/recommendations on approaches to decouple the visuals from the trading platform altogether.
Solutions I've considered so far is a named pipe solution - but, as it turns out - tends to be very platform specific. As an aside, I have finally succeeded in throwing Gates/Windows out the window and have moved entirely to linux.
I found a few developers seriously implementing json/js solutions - and wow, ok - pretty powerful stuff. Sample MT4 script below:
This is merely a wireframe/mockup of what I am actually attempting to achieve. More seriously, I'd like to work from a single .json file containing all of the class objects thus:
Then use javascript/html to render web content thus:
Producing results thus:
So, I'm running into some issues - not show stoppers - but, rather, best practices in design issues.
Using js and a typical grid utility [that supports .json] to render web content, the .json ('apparently') must be raw as in:
or
or
This indicates that my implementation would require either a dedicated .json by object type (an approach that may have a tendency to scale out of control), or a function to deserialize the .json before interfacing with the grid.
But then, deserialization appears to be an issue - how does one deserialize mutliple object 'classes' into local containers?
So, I am going to cut and run using dedicated class by class .json 'threads' - it just feels dirty.
Any suggestions?