So I have used the code from this article, it is doing the same.
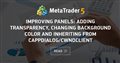
- www.mql5.com
Hello! So I have this Dialog Box with other objects on it.
My problem is that after I set these objects on a chart, once I change timeframe second time, everything becomes a mess. Objects are weird and EA is freezing. It looks like objects are deinitialized and reinitialed wrong. First time, I had only one "Destroy" function, for the Dialog Box only. And the error I got after changing the timeframe was " Objects already exists" (Error 4200 ERR_OBJECT_ALREADY_EXISTS). After that I have inserted Destroy function for all objects on the chart, but still objects are getting draw incorectly after change timeframe.
Then I have removed all objects, and leave only dialog box. Is the same. Once I change the timeframe, it is not reinitialing correctly.
Kindly advice!!
Thats not your actual code though is it? it is calling functions that don't exist so it won't even compile, for example:
GetError(InpPanelName);
and there is not return code for OnInit() etc.
Thats not your actual code though is it? it is calling functions that don't exist so it won't even compile, for example:
I did not post the entire code. Only showed a liptle just to understand what I want to do.
This function :
GetError(InpPanelName);
is actually this:
void GetError(string objname) { string err_=""; err_=StringConcatenate(ErrorDescription(GetLastError()) + " for ",objname); Print(err_); }
"InpPanelName" it is a string.
Anyway, nevermind my code, if you are using the code in the above article, it is drawing a panel, and once you are changing the timeframe twice, that panel is disrupted... cant understand why
I did not post the entire code. Only showed a liptle just to understand what I want to do.
This function :
is actually this:
"InpPanelName" it is a string.
Anyway, nevermind my code, if you are using the code in the above article, it is drawing a panel, and once you are changing the timeframe twice, that panel is disrupted... cant understand why
but you can't ignore your code - I circumvented your incomplete code and it works fine, so whatever you are doing in the rest of the code that is where your problem is
//+------------------------------------------------------------------+ //| ProjectName | //| Copyright 2018, CompanyName | //| http://www.companyname.net | //+------------------------------------------------------------------+ #property copyright "Copyright 2018, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" #property description "The MyAppWindow application based on the CMyAppDialog class" #property description "Added buttons for setting the background and header colors" //#include "MyAppDialog.mqh" #include <Controls\Button.mqh> //--- Macros for working with the color #define XRGB(r,g,b) (0xFF000000|(uchar(r)<<16)|(uchar(g)<<8)|uchar(b)) #define GETRGB(clr) ((clr)&0xFFFFFF) //+------------------------------------------------------------------+ //| defines | //+------------------------------------------------------------------+ //--- indents and gaps #define INDENT_LEFT (11) // indent from left (with allowance for border width) #define INDENT_TOP (11) // indent from top (with allowance for border width) #define CONTROLS_GAP_X (5) // gap by X coordinate //--- for buttons #define BUTTON_WIDTH (100) // size by X coordinate #define BUTTON_HEIGHT (20) // size by Y coordinate #include <Controls\MyDialogApp.mqh> //--- CMyAppDialog AppWindow; CButton m_button1; // the button object CButton m_button2; // the button object //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int _left=40,_top=40,_right=380,_btm=788; int OnInit() { // //--- create application dialog if(!AppWindow.Create(0,"CMyAppDialog: change Back and Caption colors",0,_left,_top,_right,_btm)) return(INIT_FAILED); //--- create dependent controls if(!CreateBackButton()) return(false); if(!CreateCaptionButton()) return(false); //--- run application AppWindow.Run(); //--- succeed return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- Comment(""); //--- destroy dialog AppWindow.Destroy(reason); } //+------------------------------------------------------------------+ //| Expert chart event function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, // event ID const long& lparam, // event parameter of the long type const double& dparam, // event parameter of the double type const string& sparam) // event parameter of the string type { //--- We handle button events first if((StringFind(sparam,"Back")!=-1) && id==(CHARTEVENT_OBJECT_CLICK)) { Print(__FUNCSIG__," sparam=",sparam); AppWindow.ColorBackground(GetRandomColor()); } if((StringFind(sparam,"Caption")!=-1) && id==(CHARTEVENT_OBJECT_CLICK)) { Print(__FUNCSIG__," sparam=",sparam); AppWindow.ColorCaption(GetRandomColor()); } //--- Then, all other events are processed by the CMyAppDialog class method AppWindow.ChartEvent(id,lparam,dparam,sparam); } //+------------------------------------------------------------------+ //| Create the "Button1" button | //+------------------------------------------------------------------+ bool CreateBackButton(void) { //--- coordinates int x1=INDENT_LEFT; // x1 = 11 pixels int y1=INDENT_TOP; // y1 = 11 pixels int x2=x1+BUTTON_WIDTH; // x2 = 11 + 100 = 111 pixels int y2=y1+BUTTON_HEIGHT; // y2 = 11 + 20 = 32 pixels //--- create if(!m_button1.Create(0,"Back",0,x1,y1,x2,y2)) return(false); if(!m_button1.Text("Back")) return(false); if(!AppWindow.Add(m_button1)) return(false); //--- succeed return(true); } //+------------------------------------------------------------------+ //| Create the "Button2" | //+------------------------------------------------------------------+ bool CreateCaptionButton(void) { //--- coordinates int x1=INDENT_LEFT+2*(BUTTON_WIDTH+CONTROLS_GAP_X); // x1 = 11 + 2 * (100 + 5) = 221 pixels int y1=INDENT_TOP; // y1 = 11 pixels int x2=x1+BUTTON_WIDTH; // x2 = 221 + 100 = 321 pixels int y2=y1+BUTTON_HEIGHT; // y2 = 11 + 20 = 31 pixels //--- create if(!m_button2.Create(0,"Caption",0,x1,y1,x2,y2)) return(false); if(!m_button2.Text("Caption")) return(false); if(!AppWindow.Add(m_button2)) return(false); //--- succeed return(true); } //+------------------------------------------------------------------+ //| Gets the color randomly | //+------------------------------------------------------------------+ color GetRandomColor() { color clr=(color)GETRGB(XRGB(rand()%255,rand()%255,rand()%255)); return clr; } //+----------- //+------------------------------------------------------------------+This is the code from the above article. Is copiling, kindly test, loaded and change the time frame several times
Here it is:
//+------------------------------------------------------------------+ //| ProjectName | //| Copyright 2018, CompanyName | //| http://www.companyname.net | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ #include <stderror.mqh> #include <stdlib.mqh> #include <Controls\Dialog.mqh> #include <Controls\Button.mqh> #include <Controls\Label.mqh> #include <Controls\Edit.mqh> #include <Controls\CheckBox.mqh> //#include <Controls\MyDialogApp.mqh> #include <Controls\RadioGroup.mqh> CAppDialog MyDialog; CButton Button; string InpPanelName ="MyBox"; string InpButton ="Button"; int AppDialogTop=30,AppDialogLeft=50,AppDialogWidth=390,AppDialogHeight=680; // Dialog Box //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int OnInit() { if(! MyDialog.Create(0,InpPanelName,0,AppDialogLeft,AppDialogTop,AppDialogLeft+AppDialogWidth,AppDialogTop+AppDialogHeight)) GetError(InpPanelName); if(!Button.Create(0,InpButton,0,10,10,60,60)) GetError(InpButton); Button.ColorBackground(clrGreen); Button.Font("Arial"); Button.FontSize(10); Button.Text("MyButton"); MyDialog.Add(Button); MyDialog.Run(); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { MyDialog.Destroy(reason); } void OnChartEvent(const int id,const long& lparam,const double& dparam,const string& sparam) { MyDialog.ChartEvent(id,lparam,dparam,sparam); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void GetError(string objname) { string err_=""; err_=StringConcatenate(ErrorDescription(GetLastError()) + " for ",objname); Print(err_); } //+------------------------------------------------------------------+
As I have noticed, in MT5 works, in MT4 NOT .
I can confirm your issue - I don't know why but interestingly it works as an indicator but not an EA - maybe William will enlighten me :)
I do however know of a solution/workaround. I use object pointers for such things in my products and it works fine. here is your amended code.
//+------------------------------------------------------------------+ //| Expert initialization function | //+------------------ ------------------------------------------------+ #include <stderror.mqh> #include <stdlib.mqh> #include <Controls\Dialog.mqh> #include <Controls\Button.mqh> #include <Controls\Label.mqh> #include <Controls\Edit.mqh> #include <Controls\CheckBox.mqh> //#include <Controls\MyDialogApp.mqh> #include <Controls\RadioGroup.mqh> CAppDialog *MyDialog; // declare an object pointer CButton Button; string InpPanelName ="MyBox"; string InpButton ="Button"; int AppDialogTop=30,AppDialogLeft=50,AppDialogWidth=390,AppDialogHeight=680; // Dialog Box //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int OnInit() { MyDialog = new CAppDialog; // create an object if(! MyDialog.Create(0,InpPanelName,0,AppDialogLeft,AppDialogTop,AppDialogLeft+AppDialogWidth,AppDialogTop+AppDialogHeight)) GetError(InpPanelName); if(!Button.Create(0,InpButton,0,10,10,60,60)) GetError(InpButton); Button.ColorBackground(clrGreen); Button.Font("Arial"); Button.FontSize(10); Button.Text("MyButton"); MyDialog.Add(Button); MyDialog.Run(); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { if(CheckPointer(MyDialog) != POINTER_INVALID) // never reference an invalid pointer { MyDialog.Destroy(reason); delete MyDialog; // you must delete the pointer or you will leak memory } } void OnChartEvent(const int id,const long& lparam,const double& dparam,const string& sparam) { MyDialog.ChartEvent(id,lparam,dparam,sparam); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void GetError(string objname) { string err_=""; err_=StringConcatenate(ErrorDescription(GetLastError()) + " for ",objname); Print(err_); } //+------------------------------------------------------------------+ void OnTick() {}

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello! So I have this Dialog Box with other objects on it.
My problem is that after I set these objects on a chart, once I change timeframe second time, everything becomes a mess. Objects are weird and EA is freezing. It looks like objects are deinitialized and reinitialed wrong. First time, I had only one "Destroy" function, for the Dialog Box only. And the error I got after changing the timeframe was " Objects already exists" (Error 4200 ERR_OBJECT_ALREADY_EXISTS). After that I have inserted Destroy function for all objects on the chart, but still objects are getting draw incorectly after change timeframe.
Then I have removed all objects, and leave only dialog box. Is the same. Once I change the timeframe, it is not reinitialing correctly.
Kindly advice!!