Try writing an array, see what comes out.
https://www.mql5.com/en/docs/files/filewritearray
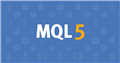
Documentation on MQL5: File Functions / FileWriteArray
- www.mql5.com
//| Demo_FileWriteArray.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| https://www.mql5.com | //| Structure for storing price data |...
Tried my best:
//--- display the window of input parameters when launching the script #property script_show_inputs //--- input parameters input string InpFileName="data.bin"; input string InpDirectoryName="SomeFolder"; //+------------------------------------------------------------------+ //| Structure for storing price data | //+------------------------------------------------------------------+ struct prices { double bid; // bid price double ask; // ask price }; //--- global variables int count=0; int size=20; string path=InpDirectoryName+"//"+InpFileName; prices arr[]; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- allocate memory for the array ArrayResize(arr,size); for (count=0; count<size ;count++) { arr[count].bid=22; arr[count].ask=223; } //--- open the file ResetLastError(); int handle=FileOpen(path,FILE_READ|FILE_WRITE|FILE_BIN); if(handle!=INVALID_HANDLE) { //--- write array data to the end of the file FileSeek(handle,0,SEEK_END); FileWriteArray(handle,arr); //--- close the file FileClose(handle); } else Print("Failed to open the file, error ",GetLastError()); }
And the result is:
Welcome
You cannot save structures with strings in them , and you cannot read strings with structures from files .
If you reopen the second file you created (the alien scribes one) and load the second structure you created with file read array
you will notice (after printing) it reads the correctly.
You cannot save structures with strings in them , and you cannot read strings with structures from files .
If you reopen the second file you created (the alien scribes one) and load the second structure you created with file read array
you will notice (after printing) it reads the correctly.
Ahh! Thank you Lorentzos. Yes it reads them correctly!
Now if you have files with strings and need to turn them to structure :
(even though , it will be slow with big files due to the constant resizing)
(even though , it will be slow with big files due to the constant resizing)
input string FileName="data.bin";//File Name input string Folder="";//File Folder input string Delimeter=" ";//Separator -------------------------------------- //Data and desired structure struct exportdata { datetime time; double ask; double bid; }; exportdata EXP[]; int EXP_Total=0; //Data and desired structure ends here //Open text file string location=FileName; string raw_line_feed; //Line splitter prep ushort u_sep; // The code of the separator character string result[]; // An array to get strings //--- Get the separator code u_sep=StringGetCharacter(Delimeter,0); //Line splitter prep ends here if(Folder!="") location=Folder+"\\"+location; int fop=FileOpen(location,FILE_READ|FILE_TXT); while(!FileIsEnding(fop)) { raw_line_feed=FileReadString(fop); int split=StringSplit(raw_line_feed,u_sep,result); //increase if(split==3) { EXP_Total++; ArrayResize(EXP,EXP_Total,0); EXP[EXP_Total-1].time=StringToTime(result[0]); EXP[EXP_Total-1].ask=StringToDouble(result[1]); EXP[EXP_Total-1].bid=StringToDouble(result[2]); Print("["+IntegerToString(EXP_Total)+"] Time :"+TimeToString(EXP[EXP_Total-1].time,TIME_DATE)+" A:"+DoubleToString(EXP[EXP_Total-1].ask,2)+" B:"+DoubleToString(EXP[EXP_Total-1].bid,2)); } //increase ends here } FileClose(fop);
Ziad El:
Perhaps you should read the manual. Separated is a text file not binary.For some reason I am not able to use the FileReadArray function: 1. How should fields be separated? TAB? space? semicolon? I used TABs
Please help, as I cannot find any resource on the internet giving a full example and complete explanation about this topic
Reads from a file of BIN type arrays of any type except string (may be an array of structures, not containing strings, and dynamic arrays).
File Functions / FileReadArray - Reference on algorithmic/automated trading language for MetaTrader 5
File Functions / FileReadArray - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
For some reason I am not able to use the FileReadArray function:
1. How should fields be separated? TAB? space? semicolon? I used TABs
2. How should datetime be entered? I used D'01.01.2004' format
I copied and run the following code from MQL tutorials:
My Data bin file and results are as follows:
Please help, as I cannot find any resource on the internet giving a full example and complete explanation about this topic