So I read this:
"If instead of using a variable for the array size you call the ArraySize() function when checking condition in a for loop, the looping time may be significantly prolonged as the ArraySize() function will be called at every loop iteration. So the function call takes more time than calling a variable"
And changed the ArraySize() to be a variable instead, slight improvement but still painfully slow
int Size=ArrayRange(News,0); for(int i = 0; i < Size; i++) if(Gmt(TimeCurrent()) == StringToTime(News[i][0])) { Print(News[i][0], News[i][1], News[i][2], News[i][3]); }
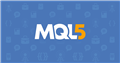
- www.mql5.com
So I read this:
"If instead of using a variable for the array size you call the ArraySize() function when checking condition in a for loop, the looping time may be significantly prolonged as the ArraySize() function will be called at every loop iteration. So the function call takes more time than calling a variable"
And changed the ArraySize() to be a variable instead, slight improvement but still painfully slow
I cannot estimate the structure of your file, but let me assume following.
You are using a CSV-File which you want to read.
The file should have two types of delimiters. -> One to separate the different datasets from each other, and another to separate the values inside the dataset.
Lets say a dataset consists of one line of text in your file, separated by CRLF "\r\n".
Then the values would most probably be separated by a comma, right?
Well, then do exactly that.
short delimiter = ('\r' << 8) + '\n'; // This will give you "\r\n" as the file delimiter in a short (16-bit) variable (2 Bytes). int Handle = FileOpen("Events.txt", FILE_TXT | FILE_COMMON | FILE_READ | FILE_ANSI, delimiter);
Then, when you split the string into its values, you can use the comma as separator.
If that doesnt help, maybe you want to process tha fiel as binary, using a char-array and read the file into memory in one function call. Then process the array with a loop, with the most simple approach possible. Use ternary operator. "variable = (condition) ? expr1 : expr2";
I would guess, you cannot get faster than that.
I cannot estimate the structure of your file, but let me assume following.
You are using a CSV-File which you want to read.
The file should have two types of delimiters. -> One to separate the different datasets from each other, and another to separate the values inside the dataset.
Lets say a dataset consists of one line of text in your file, separated by CRLF "\r\n".
Then the values would most probably be separated by a comma, right?
Well, then do exactly that.
Then, when you split the string into its values, you can use the comma as separator.
If that doesnt help, maybe you want to process tha fiel as binary, using a char-array and read the file into memory in one function call. Then process the array with a loop, with the most simple approach possible. Use ternary operator. "variable = (condition) ? expr1 : expr2";
I would guess, you cannot get faster than that.
Would dividing the file by years not help much?
I'll try the binary and char-array
Thank you
Actually not starting from zero every time improved the speed by 1 minute. But still, 01:26 minute for a month back test is still slow.
int memory=0; datetime t=TimeCurrent(); int Size=ArrayRange(News,0); for(int i = memory; i < Size; i++) if(Gmt(t) == StringToTime(News[i][0])) { Print(News[i][0], News[i][1], News[i][2], News[i][3]); memory=i; }
You can exit the loop when the current date is before an event
int memory=0; datetime t=TimeCurrent(); int Size=ArrayRange(News,0); for(int i = memory; i < Size; i++) if(Gmt(t) == StringToTime(News[i][0])) { Print(News[i][0], News[i][1], News[i][2], News[i][3]); memory=i; }else if(Gmt(t)<StringToTime(News[i][0])){break;}
And this :
Compare integers instead of strings.
(sorry for the constant edits mod , the interface is very easy to use)
Stop doing the same things multiple times | datetime t=TimeCurrent(); int Size=ArrayRange(News,0); for(int i = memory; i < Size; i++) if(Gmt(t) == StringToTime(News[i][0])) { Print(News[i][0], News[i][1], News[i][2], News[i][3]); memory=i; }else if(Gmt(t)<StringToTime(News[i][0])){break;} |
Simplify your code | datetime t=Gmt(TimeCurrent()); int Size=ArrayRange(News,0); for(int i = memory; i < Size; i++) datetime n=StringToTime(News[i][0]); if(t == n) { Print(n, News[i][1], News[i][2], News[i][3]); memory=i; }else if(t < n){break;} |

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello
I'm trying to read a news file on back test from 2000 until present.
Is there a way to read the Array quicker? because what I'm trying for now is painfully slow