correct is: 1-2/21=0.9048
tema1ema1[19] = MathMean(typical); //typical is an array for the last 20 bars where 0 is the most recent for(int i=1;i<20;i++) { tema1ema1[19-i] = typical[19-i] * .0952 + tema1ema1[19-i+1] * .9048; //price * 2/21 + lastcalculation * (1- 2/21) } tema1ema2[19] = MathMean(tema1ema1); for(int i=1;i<20;i++) { tema1ema2[19-i] = tema1ema1[19-i] * .0952 + tema1ema2[19-i+1] * .9048; } tema1ema3[19] = MathMean(tema1ema2); for(int i=1;i<20;i++) { tema1ema3[19-i] = tema1ema2[19-i] * .0952 + tema1ema3[19-i+1] * .9048; } tema1out = (3*tema1ema1[0])-(3*tema1ema2[0])+tema1ema3[0]; //output
I did not test this...
I tried with 200 and it was accurate but for 150 not very accurate. I tried comparing the result of your code with another version.
Here is the link to the formula:
https://www.investopedia.com/terms/t/triple-exponential-moving-average.asp
The amount of data you require is 4 times (+ 3 extra) the period count of the TEMA to be calculated.
Lets say you want an TEMA with 20 periods. then from left to right (oldest to newest), you do the following:
Periods 1 to 20 -> SMA value. -> this is your initialization value for your first EMA.
Now you calculate the EMA for periods 21 to 83, beginning with the SMA value as offset (using a period count of 20 for the algorithm).
Now you repeat this process for the next EMA. EMA2
Calculate the SMA on the results of the EMA1 from period 21 to 40.
Use this value to initialize your second EMA, EMA2.
Now calculate the EMA2 on periods 41 to 83 on the results of EMA1 (using a period count of 20 for the algorithm)
Repeat the process accordingly to get the EMA3 values, as you did for EMA2.
Calculate the SMA from EMA2 results on periods 41 to 60.
Now calculate the EMA3 on the results of EMA2 from period 61 to 83 (using a period count of 20 for the algorithm).
Now your values 80 to 83 have valid EMAs, and you can calculate the actual TEMA value according to the formula:
TEMA = (3* EMA1) - (3 * EMA2) + EMA3;
EMA1 = value from period 81 of the EMA1 array.
EMA2 = value from period 82 of the EMA2 array.
EMA3 = value from period 83 of the EMA3 array.
For subsequent updates, you can actually feed in the last EMA(1, 2, 3) value into the EMA calculation as your init_value, and shortcut the long process of initially building the EMA series. - So you replace the SMA value you have to calculate initially with the last EMA value calculated on that series. This will make the algorithm very efficient.
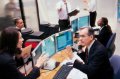
- www.investopedia.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to code my own triple exponential moving average. Here is what I have:
Is this correct? Just making sure from the onset.