Here is no error:
//+------------------------------------------------------------------+ //| Experttutorial.mq5 | //| Copyright © 2021, Vladimir Karputov | //+------------------------------------------------------------------+ #property copyright "Copyright © 2021, Vladimir Karputov" #property version "1.00" //--- #include <Trade\Trade.mqh> CTrade m_trade; // object of CTrade class //--- input parameters input int Inp_MA_ma_period = 12; // MA: averaging period input int Inp_MA_ma_shift = 6; // MA: horizontal shift input ENUM_MA_METHOD Inp_MA_ma_method = MODE_SMA; // MA: smoothing type input ENUM_APPLIED_PRICE Inp_MA_applied_price = PRICE_CLOSE; // MA: type of price //--- int handle_iMA; // variable for storing the handle of the iMA indicator bool m_init_error = false; // error on InInit //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- forced initialization of variables m_init_error = false; //--- create handle of the indicator iMA handle_iMA=iMA(Symbol(),PERIOD_D1,Inp_MA_ma_period,Inp_MA_ma_shift, Inp_MA_ma_method,Inp_MA_applied_price); //--- if the handle is not created if(handle_iMA==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iMA indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(PERIOD_D1), GetLastError()); //--- the indicator is stopped early m_init_error=true; return(INIT_SUCCEEDED); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(m_init_error) return; //--- if(PositionsTotal()==0) { double ma_buffer[]; MqlRates rates[]; ArraySetAsSeries(ma_buffer,true); ArraySetAsSeries(rates,true); int start_pos=0,count=3; if(!iGetArray(handle_iMA,0,start_pos,count,ma_buffer) || CopyRates(Symbol(),PERIOD_D1,start_pos,count,rates)!=count) return; //--- MqlTick tick; if(!SymbolInfoTick(Symbol(),tick)) return; //--- if(ma_buffer[0]>rates[0].close) m_trade.Sell(0.10,Symbol(),tick.bid,tick.bid+100*Point()); } } //+------------------------------------------------------------------+ //| Get value of buffers | //+------------------------------------------------------------------+ bool iGetArray(const int handle,const int buffer,const int start_pos, const int count,double &arr_buffer[]) { bool result=true; if(!ArrayIsDynamic(arr_buffer)) { PrintFormat("ERROR! EA: %s, FUNCTION: %s, this a no dynamic array!",__FILE__,__FUNCTION__); return(false); } ArrayFree(arr_buffer); //--- reset error code ResetLastError(); //--- fill a part of the iBands array with values from the indicator buffer int copied=CopyBuffer(handle,buffer,start_pos,count,arr_buffer); if(copied!=count) { //--- if the copying fails, tell the error code PrintFormat("ERROR! EA: %s, FUNCTION: %s, amount to copy: %d, copied: %d, error code %d", __FILE__,__FUNCTION__,count,copied,GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } return(result); } //+------------------------------------------------------------------+
Here is no error:
Hi, I entered the code but I understand that it does not work and no signals are generated , as this is happening to me with almost all the codes , do you think it is a platform problem?
Hi, I entered the code but I understand that it does not work and no signals are generated , as this is happening to me with almost all the codes , do you think it is a platform problem?
If the code canb be compiled without any error but it doesn't do what you expect use the debugger to find out why - it is exactly made for this.
https://www.metatrader5.com/en/metaeditor/help/development/debug
https://www.mql5.com/en/articles/2041 // Error Handling and Logging in MQL5
https://www.mql5.com/en/articles/272
https://www.mql5.com/en/articles/35 // scrol down to: "Launching and Debuggin"
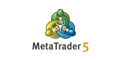
- www.metatrader5.com
Hi, I entered the code but I understand that it does not work and no signals are generated , as this is happening to me with almost all the codes , do you think it is a platform problem?
100% of the problem is you. Look for the problem in yourself. You may have confused the terminals (for example, you take the MQL5 code and try to run it in the old MetaTrader 4 terminal). Perhaps you are trying to trade some kind of exotic (for example, instead of the foreign exchange market, you are trying to trade coffee or cocoa).
Perhaps there are still 1,000,000 reasons - but We do not know about them, so you DO NOT REPORT ANYTHING AT ALL !. You do not show on which symbol and on which timeframe you trade, you do not show the log file ...
100% of the problem is you. Look for the problem in yourself. You may have confused the terminals (for example, you take the MQL5 code and try to run it in the old MetaTrader 4 terminal). Perhaps you are trying to trade some kind of exotic (for example, instead of the foreign exchange market, you are trying to trade coffee or cocoa).
Perhaps there are still 1,000,000 reasons - but We do not know about them, so you DO NOT REPORT ANYTHING AT ALL !. You do not show on which symbol and on which timeframe you trade, you do not show the log file ...

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi , this is one of the first and to which I plan , and my intention was to build a system that would sell when the MA is greater than current price and the previous price is greater than the MA , but i am having a problem trouble entering the prices , informing me on the web and i was consultuing che documentation i was building a price array , but I am reported an infinity of errors , could you tell me where am I wrong ?
this is the code :