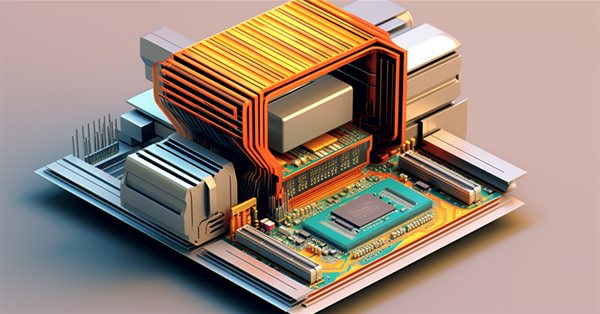
Master MQL5 from beginner to pro (Part I): Getting started with programming
Introduction
I sometimes receive private messages from those who want to learn how to create their own Expert Advisors or indicators. Although there is a lot of material on this site and on the Internet in general, including very good resources with examples, beginners still need help. Some users seek more consistency in presentation, others require clarity or something else. Sometimes users ask: "Add comments to the code of a working Expert Advisor, I will understand everything and make the same one myself!" But, after they see a hundred lines of code, they get scared, confused and give up the idea. Instead, they say: "Better create an Expert Advisor for me."
Anyway, there are people who still want to "understand everything". This is when a step-by-step guide, explaining things from simple to complex, may come in handy. Well, users can't find such a step-by-step guide for the MQL5 language.
Those manuals that I have seen assume that a person already has some experience programming and understands how it works. Therefore, the guides offer explanations of nuances.
I am starting this series of articles specifically for beginners, for those who are encountering programming for the first time and are willing to learn it. These articles are intended for traders who no longer want to depend on programmers and wish to understand how everything works. The articles offer consistent and detailed information, with pictures and examples, guiding readers to the level of "MQL5 language proficiency".
This language can be used not only to automate trading but also to solve other related trading tasks. For example, you can use it to plot indicators, optimization results and any custom shapes, to create files, such as lists of tick quotes or screenshots of the current trading situation, to send messages to Telegram and other messaging bots, to work with databases, and much more.
I want to gradually showcase the maximum capabilities of the language: the use of "virtual symbols", operations with mathematical libraries, and much more. This time we start with the basics.
Basic technical terms
Almost all modern computers are built based on the Von Neumann architecture. In order to successfully read literature, you need to understand the terms that programmers use.
- Central Processing Unit (or CPU). This module is responsible for all computations and logic.
- Random Access Memory (RAM, memory). RAM stores data and programs. When you turn off the computer or close a program, the data from the RAM is erased.
- External drives (flash drives, HDD, SSD, etc.). The CPU can write data to them as files.
- Files mean information written on external media (not in RAM).
- Execution thread. During operation, the CPU takes data from the RAM, "understands" whether it is a command or data, executes the command and writes the results either back to the RAM or to an external drive indicated by the running program. Then it reads the next memory fragment again, and so on until the program ends. This entire process is called the "execution thread".
Various metrics exist for measuring information.
- Bit is the most basic unit of information. Actually, it is a "switch" that determines the state of a specific, tiniest piece of memory. A bit contains either "0" (disabled) or "1" (enabled).
- Byte can be explained as a "syllable". It is the smallest "package" of information equal to 8 bit. When computers were large and took up entire floors, the amount of RAM was tiny, and files were stored on punch cards, 8 bits were the most convenient technical compromise between speed (as many as 8 holes can be read at the same time!) and media size.
It is sometimes important for a programmer to understand how much space a program will take up. In the modern world, programs (and especially their data) usually take up a lot of space, so metric prefixes are added to the word "byte".
When measuring physical rather than informational quantities, each such prefix reports an increase in the previous size by 1000 times. For example, a kilometer is equal to 1000 meters, and a megavolt is 1000 kilovolts or 1,000,000 volts.
However, when are we talking about computer data, each next metric prefix is larger than the previous one by 1024 times.
For example, kilobyte (KB) is equal to 1024 bytes, megabyte (MB) contains 1024 kilobytes), and so on. Further prefixes are: giga, tera, peta, etc.
We will deal with memory management, commands to the processor, file writing using MQL5 and other routine things in later articles.
Terms in bold will often appear in articles, so I describe them here at the very beginning. Other terms will be introduced in the place where they are encountered for the first time. I can't do without terms, but I'll try to use a minimum of them.
Software required to start programming in MQL5
To make a computer useful to people, you need to write a program for each type of activity: trading, viewing pictures, listening to music, and others.
It is quite possible to write programs directly in processor commands. But this is very unproductive.
Therefore, in the modern world, there are many assistant programs which help convert text written in languages close to human into commands understandable to processors.
Here is a minimum list of such programs required for programmers:
-
Text editor. In this software, you write your code. Any editor you like will do. However, it should not add any extra characters to the text that you type. It is very convenient when an editor can highlight the syntax of the language you need (in this case, MQL5).
When you install MetaTrader, a very powerful editor called MetaEditor is automatically installed on the system. It can do everything you need.
However, if for some reason you don't like it, you are free to choose anything else. For example, one of the very popular editors used by programmers is Visual Studio Code (VSCode). Using plugins, you can configure it to work with MQL5.
-
Compiler. The compiler converts text written by a programmer in an editor to machine code. Only after conversion, the CPU can execute this code.
For the MQL5 language, the compiler is built into MetaEditor. For other languages, such as C++, the compiler may be a separate program.
Some languages, such as Python, do not have an explicit compiler. They sort of execute text from your file directly.
The program that reads files for such languages is called an "interpreter", and the languages themselves are accordingly referred to as "interpreted". However, it is important to understand that even interpreters eventually execute machine code. The difference is that the conversions from text to code are not so obvious.
-
Debugger. This tool allows you to find and fix errors in your program. It allows the step-by-step execution of code aiming at checking the data your program is working with at any given time at each step.
MetaEditor provides a built-in debugger.
-
Version Control System (VCS). Such a system assists in keeping track of changes in your code.
If you have written a lot of code and suddenly realize that the version that was a day ago worked much better or you generally need to take a different path, a VCS will allow you to "revert" to the previous state of the file (and even the entire project) in just a few clicks or text commands from the keyboard.
Most importantly, VCSs often store data on a remote server, rather than on your local computer, ensuring that if a failure occurs (for example, a hard drive crashes), you can quickly restore your work.
These two important factors have made different version control systems virtually mandatory for corporate work because they also allow for collaboration with other developers. The habit of using these tools also helps a lot when working independently, because different things can happen.
There are third-party solutions such as Git. However, such a tool is also built into MetaEditor. The capabilities of the built-in VCS will be more than enough for beginners.
This is the toolkit without which modern programming cannot exist. Programmers might use versioning tools separately. But in the modern world, this happens relatively rarely. More commonly users choose software such as MetaEditor, which includes all these features (and even more).
The software solutions that include all of the above components (text editor, compiler, debugger and others) are referred to as the Integrated Development Environment (IDE).
Main types of MQL5 programs
The program applications that can be executed in MetaTrader 5 can be divided into four types:
- Scripts. They can display information, trade, or display graphical objects. In order to execute the script, you should run it anew every time you need it.
- Indicators. They are designed to display information for a trading strategy on each tick. They do not trade on their own.
- Expert Advisors (EAs). These are programs designed for trading. Like indicators, they are executed on every tick, but they do not possess all the tools for drawing arrows or useful lines.
- Services. Unlike all previous types of programs, services do not require any specific chart to operate. They run once at terminal startup. You can force them to remain in memory until the terminal is closed (and even for some time after that), but they cannot respond to terminal events, such as the arrival of a new tick or a mouse click.
Comparison of program types available in MQL5
Everything related to the use of programs in MQL5 can be summarized in a simple table:
Table 1. Comparing the capabilities of the main MQL5 program types.
Program capabilities | Scripts | Indicators | Expert Advisors | Services |
---|---|---|---|---|
Linked to a specific chart | yes | yes | yes | no |
Can tradС | yes | no | yes | yes |
Unloaded from memory when the timeframe changes | yes | yes | yes | no |
Executed on every tick | no | yes | yes | no |
Executed once when the terminal is opened | no | no | no | yes |
Run while the chart window is open | no | yes | yes | no |
Can handle various terminal or chart events | no | yes | yes | no |
Can start and stop the timer | no | yes | yes | no |
Automatically call initialization and deinitialization functions | no | yes | yes | no |
Only one instance of the program can be executed on the chart | yes | no | yes | — |
Functions
Every program should do something, that is, to perform some functions.
Any function is a set of program actions leading to some (often elementary) result. For example, they print a line of text, draw one letter, or open a deal, among others.
Some programming languages do not require explicit declarations of functions. Such a declaration is mandatory in MQL5: each program must have at least one declared start function, otherwise there will be an error message. For each type of program, the initial functions will be called differently. However, in any case, a program must have functions, otherwise it becomes meaningless as it does nothing at all.
Almost any programming language allows the creation of custom functions to separate pieces of code that are repeated, and then reuse those pieces in one line, rather than rewriting them over and over again. In addition, all languages have sets of predetermined functions. It is these predefined functions that distinguish one programming language from all others.
Actually, when talking about a language, the authors often try to describe which functions are predefined in it and how to best use them to get the desired result.
MQL5 is no exception. It contains a set of built-in functions and allows the creation of custom ones. The built-in functions of the language can be conveniently studied using the context help. To call Help, open any code (even the one written by someone else) in MetaEditor, click on any highlighted word and press the <F1> key.
Certain types of programs cannot call certain built-in functions. Some, as already mentioned, must be implemented exactly as described in the help. Furthermore, different types of programs will require different functions.
But otherwise, our creativity is almost unlimited. We can create any helper functions, organize them in any convenient way, and even use functions described in other languages, such as C++ or Python. We will definitely discuss all these features in future articles.
But now let's start with the simplest thing that the MQL5 language allows us to create: we will create a script that will be executed once when it is dragged onto the chart with the mouse. Using a standard MQL5 language function, in this script, we will display a regular text message for the user in a standard panel at the bottom of the terminal.
Launching and setting up the IDE
Finally, we got to practice! For now, we will do all the work in MetaEditor. It can be launched either using a shortcut (from the desktop or the Start menu), or directly from the MetaTrader terminal using one of the methods shown in the figure below (Figure 1).
.
Figure 1. Three ways to open MetaEditor: 1 - toolbar button, 2 - menu, 3 - hotkey (F4)
Once the editor window is open, I recommend immediately entering your username and password from the mql5.com website (or, if you are not registered yet, do so). Logging in will allow you to save your projects directly to the server using the version control solution built into MetaEditor. No one will see your saved files unless you allow access, so saving your work in the MetaQuotes cloud is as safe as storing information on the Internet can be, and this is a good practice (storing your work not only on your computer).
Figure 2. MQL5.com authorization dialog
To open the authorization dialog, select Tools -> Settings in the main menu of the editor window and navigate to the Community tab. This setting only needs to be done once. These data will be saved in the editor, and you will be able to smoothly utilize the version control system and other useful MQL5 services.
First script
Now... Just right-click on the folder in the left dashboard where we want to create the script and select "New File". Optionally, you can press <Ctrl>+<N>... In the window that appears, select Script. In the next window, enter the file name.
Figure 3. Methods for creating a new file.
Figure 4. File creation Wizard dialog - select the type of the program to be created.
Figure 5. File creation Wizard dialog - type the name of the program to be created.
As a result, you get a new window with the following content:
//+------------------------------------------------------------------+ //| HelloWorld.mq5 | //| Oleg Fedorov (aka certain) | //| mailto:coder.fedorov@gmail.com | //+------------------------------------------------------------------+ #property copyright "Oleg Fedorov (aka certain)" #property link "mailto:coder.fedorov@gmail.com" #property version "1.00" //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- } //+------------------------------------------------------------------+
Example 1. Script template generated by the Wizard.
It seems nothing complicated, only 17 lines of code.
Let's see what exactly is written here.
- First, there are several lines at the beginning, starting with double slash characters ( // ). Similar lines also appear in the middle of the program.
These are comments. They are written for people, so the compiler will ignore them when generating bytecode. Comments that begin with a double slash are referred to as single-line comments. They are placed at the end of the line. The rule is very simple: everything that comes before the double slash will be considered by the compiler as code, and everything that follows the double slash is text for a human.
In this wizard-created template, the "before" code was not required in this context.
In MetaEditor you can quickly comment out a few lines: select them and press the keyboard shortcut " <Ctrl>+< ' > (Ctrl + apostrophe). To uncomment such lines, select them again and press the keyboard shortcut <Ctrl>+< ; >.
There are also multi-line comments. They start with the characters /* and end in the reverse sequence */. Multi-line comments can be inserted anywhere in the code where whitespace is acceptable. When compiling, the compiler also first "removes" such sequences, and only then tries to figure out what the author wanted to implement.
In practice, comments are inserted into the middle of code quite rarely. Most often it is simply inconvenient. However, they are very useful in commenting on large blocks of unnecessary code or describing function headers.
Here's how the header of this file could look like if we used a multi-line comment (if I wrote the code myself rather than have it generated by the MetaEditor Wizard):
/*
HelloWorld.mq5
Oleg Fedorov (aka certain)
mailto:coder.fedorov@gmail.com
*/
Example 2. Multi-line comments.
Or like this:
/*******************************************************************
* HelloWorld.mq5 *
* Oleg Fedorov (aka certain) *
* mailto:coder.fedorov@gmail.com *
*******************************************************************/
Example 3. Decorated multi-line comments.
Generally, there can be any text in any language between the characters /* and */ or after //. I say that in any language because modern editors fully support Unicode encoding. However, it is still recommended to use English, since this saves time on switching languages (which is quite essential in large projects).
- The second interesting block consists of the lines starting with #. In this file, the initial words of each line of this block are #property.
Generally speaking, every word starting with a hash sign is a preprocessor directive. The compiler must not only remove all comments before converting text into machine code. It must also make other preparations. There is usually quite a lot of preparatory work because in large projects it is necessary to assemble the resulting solution from several files, define some data (for example constants like the number Pi or something similar, although there are more complex cases), and so on. We will consider all these directives much later.
For now, to understand the resulting code, we need to know that the #property directive describes some property of the resulting program. Most often, these properties affect the initial window, which appears immediately after the user launches our program by dragging its icon onto the chart.
To illustrate this, let's add one more line to the code:
#property copyright "Oleg Fedorov (aka certain)" #property link "mailto:coder.fedorov@gmail.com" #property version "1.00" #property script_show_inputs
Example 4. Adding the property script_show_inputs.
This line will allow you to display the inputs window, and only then start executing the main code. If this line is not present, the script will start executing immediately without displaying any messages. If there are any inputs, the script will use default values.
So, having written the required line to the desired location in the file (immediately after the 'version' property), we will run the first compilation of our program.
Select one of the methods shown in the figure (for example, press the <F7> key).
Figure 6. Several ways to compile a program.
Wait for a few seconds. That's it - the program has been compiled (Figure 7).
Figure 7. Compilation results. In this case, the compilation was successful (0 errors). It took 527 milliseconds and created the bytecode for a 64-bit processor.
Figure 9 shows that the compilation was successful. If there were errors in the text file, messages about them would appear in the same window.
After compilation, the script icon will automatically appear in the list of scripts in your trading terminal. To switch to the terminal, you can, for example, press the <F4> key in the editor. This will save some time.
Drag the script onto the chart with the mouse and see the properties window.
Figure 8. Script inputs window.
(1)The icon of the running script is displayed in the upper right corner of the main window.
(2) The Common tab shows the use of properties described using #property directives.
(3) MetaEditor has added additional parameters to the address specified in the "link" property.
Probably, this shows where the visitors come from if we use a website link.
Let's close the window, return to the editor and try to instruct the script to do something useful, for example, display a message for the user.
To do this, comment out the line that displays the parameters window (we don't have anything to change yet) and add another one that writes a message:
//+------------------------------------------------------------------+ //| HelloWorld.mq5 | //| Oleg Fedorov (aka certain) | //| mailto:coder.fedorov@gmail.com | //+------------------------------------------------------------------+ #property copyright "Oleg Fedorov (aka certain)" #property link "mailto:coder.fedorov@gmail.com" #property version "1.00" //#property script_show_inputs //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- Print("Hello, MQL5 world!"); } //+------------------------------------------------------------------+
Example 5. A script that prints a string to a user log.
- Pay attention to the predefined words.
In this code, the predefined words are #property, copyright, link, version, void, OnStart, and Print. Try clicking on any of them in the MetaEditor and pressing <F1> to open the built-in help.
- Inside the OnStart function generated by the wizard, we called another standard function Print. Using parameters (they are also referred to as arguments) listed in brackets we inform the Print function what exactly it should print.
In Example 5, the Print function receives only one argument: the text that is written in quotes. In the general case, there may be several such arguments. For example, this Print function can be called with any number of parameters (up to 64).
If a function needs to receive multiple parameters, these parameters should be separated by a comma, as in Example 6:
Print("Hello, MQL5 World!", " I have written my own program. ", "Hooray!");
Example 6. Function taking three parameters
After each comma that separates parameters, you can add a line break character (using the <Enter> key), so as not to get confused in quotes.
Also pay attention to spaces inside the second argument. The Print function "merges" its arguments into one large line, so the programmer must take care of all punctuation marks and additional separators between words.
The OnStart function is executed automatically, once, at the script or service startup.
We compile and run the script in the already known way.
Figure 9. First useful action: outputting a string to a log.
Conclusion
Expert Advisors and indicators also have predefined functions but with different names. The principle of their operation is the same as that of script functions: our code is written mainly internally, and the properties are written externally. But there are also differences.
For example, some functions in Expert Advisors and indicators are executed by the terminal at every tick, and not just once as OnStart.
Therefore, I suggest that those who want to check what they have learned from the article (and to refresh their knowledge) do a little "home research" on their own:
- Use the file creating Wizard to generate all other types of programs not covered by the examples in this article.
- Consider whether using the tools described in the article, you can find out which functions are called and their call order when each type of program starts.
- As an extra task, you can check what will happen if:
- you add the OnStart function to indicators and Expert Advisors
- you add the functions that the wizard creates for other types of programs into scripts and services
Perhaps these studies will bring you new ideas and will assist you in better understanding how exactly programs in the MQL5 language work.
I wish you good luck. See you soon in the next articles!
Translated from Russian by MetaQuotes Ltd.
Original article: https://www.mql5.com/ru/articles/13594





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Thanks, but I'm kind of self-taught. So it wouldn't hurt me either. So I thought I'd do some reading...
Thank you.
Here's the first point.
I don't think it's quite right. After all, it can be understood as the active window of the chart...
I thought that mentioning that services do not belong to the chart in principle, but to the whole terminal, removes this problem... Perhaps I am wrong.
Thank you very much for this phrase
It is very important for a beginner to understand it. You just have to read the documentation and find a way to solve your fantasy...
Cheers :-)
Second remark
Can OnStart and Print be called words like copyright,link,version? I think it may confuse a beginner in programming. OnStart and Print are functions. And OnStart is a function of event processing.
In this context , I think you can... Because <F1> will call the help for either of them.
OnStart and Print are predefined functions, in fact, a part of the language, without which it would be very different. After all, all the basic operators in MQL5 are very similar to C++, but the language is quite different for me...
And so, if you sort it out, there is a whole bunch of word types: preprocessor directives, property names and their values, function descriptions and their calls, and even data type description.... In terms of meaning, of course, all these are different pieces of the mosaic, you are absolutely right.
My verdict: The article is of high quality and useful for beginners.
Senkaiu.
Thank you.
I thought that mentioning that the services don't belong to the graph in principle, but to the whole terminal, removes this problem... I may be wrong.
This table contains not only services, but also indicators and Expert Advisors. Although, I cannot formulate it differently, so let it be as it is until someone suggests a more acceptable expression....
Please get to the structures and lay it all out. This is also a very interesting and important topic! The main thing in the series of articles is that all training material, taking into account its degree of complexity, should be dosed, smoothly and progressively.
Obligatory.
In my previous post I meant that in my next article I don't know if I will capture this material. In terms of meaning, it resonates more with classes for me.
It's just that the series is quite long, I want to do at least seven articles to cover as many important topics as possible. Maybe more, if the material requires it and the moderator allows it. :-)
What else would be interesting for a beginner, based on my personal experience of self-study:
Thank you.
Well, in my opinion, beginners need indicators too. And events are a good thing. And many other things.
In general, beginners, stay on the line. :-)
But thanks to the experienced developers for their opinion too. I'm glad you found the material useful.
I liked the article. It would be great if there was an opportunity to discuss with the author the difficulties that arise and solve them. I intend to continue to study the articles from this series.