Can someone help me check this EA code to see if there are any errors and also I need help to optimize it. Just a newcomer here
- Invalid array access
- Help me fix this code pls
- Error message: 'close' - invalid array access & 'ticket' - variable already defined
Can someone help me check this EA code to see if there are any errors and also I need help to optimize it. Just a newcomer here
Why?
You want to trade two ema, if you would have googled for "site:mql5.com EA two ema" yopu would have found e.g. this: https://www.mql5.com/en/forum/20297#comment_763463
Or searching here: https://www.mql5.com/en/search#!keyword=two%20ema you can find lots of working examples.
Bear in mind there's virtually nothing that hasn't already been programmed for MT4/MT5 and is ready for you!
If you place the cursor on an MQL function and press F1, you will see the reference directly, many with examples to copy and paste - the fastest form to code.
https://www.mql5.com/en/articles/496
https://www.mql5.com/en/articles/100
https://www.mql5.com/en/articles/599
and for debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
https://www.mql5.com/en/search#!keyword=cookbook
=> Search in the articles: https://www.mql5.com/en/articles
=> Search in the codebase: https://www.mql5.com/en/code
=> Search in general: https://www.mql5.com/en/search or via Google with: "site:mql5.com .." (forgives misspelling)
indicators: see this series of articles:
https://www.mql5.com/en/users/m.aboud/publications
Each article explains an indicator (built into MT5) and what it tells you, and how you might use it in an EA.
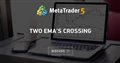
- 2014.02.12
- www.mql5.com
-
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)ChatGPT (the worst), “Bots Builder”, “EA builder”, “EA Builder Pro”, EATree, “Etasoft forex generator”, “Forex Strategy Builder”, ForexEAdvisor (aka. ForexEAdvisor STRATEGY BUILDER, and Online Forex Expert Advisor Generator), ForexRobotAcademy.com, forexsb, “FX EA Builder”, fxDreema, Forex Generator, FxPro, Molanis, Octa-FX Meta Editor, Strategy Builder FX, Strategy Quant, “Visual Trader Studio”, “MQL5 Wizard”, etc., are all the same. You will get something quick, but then you will spend a much longer time trying to get it right, than if you learned the language up front, and then just wrote it.
Since you haven't learned MQL4/5, therefor there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into yours.
We are willing to HELP you when you post your attempt (using Code button) and state the nature of your problem, but we are not going to debug your hundreds of lines of code. You are essentially going to be on your own.
ChatGPT - Even it says do not use it for coding. *
- Mixing MT4 and MT5 code together.
- Creating multiple OnCalculate/OnTick functions.
- OnCalculate returning a double.
- Filling buffers with zero in OnInit (they have no size yet). Setting buffer elements to zero but not setting Empty Value to correspond.
- Calling undefined functions.
- Calling MT4 functions in MT5 code.
- Sometimes, not using strict (MT4 code).
- Code that will not compile.
- Creating code outside of functions. *
- Creating incomplete code. *
- Initialization of Global variables with non-constants. *
- Assigning a MT5 handle to a double or missing the buffer and bar indexes in a MT4 call. *
- Useing MT4 Trade Functions without first selecting an order. *
- Uses NULL in OrderSend. *
bot builder Creating two OnInit() functions. * EA builder EATree Uses objects on chart to save values — not persistent storage (files or GV+Flush.) No recovery (crash/power failure.) ForexEAdvisor - Non-updateing global variables.
- Compilation errors.
- Not checking return codes.
- Not reporting errors.
FX EA Builder - Not checking return codes.
- Loosing open tickets on terminal restart. No recovery (crash/power failure.)
- Not adjusting stops for the spread. *
- Using OrdersTotal directly.
- Using the old event handlers.
-
ulong ticket = OrderSend(Symbol(), OP_BUY, lotSize, openPrice, 2, 0, stopLoss, takeProfit, 0, clrNONE);
This is MT4 code.
-
if (PositionsTotal() > 0) { for (int i = 0; i < PositionsTotal(); i++) {
MT4 does not have positions.
Your topic has been moved to the section: Expert Advisors and Automated Trading — Please consider which section is most appropriate — https://www.mql5.com/en/forum/443428#comment_49114884
Everyone else is going to tell you not to use ChatGPT. I'll offer some suggestions about how to use ChatGPT.
- It constantly makes the mistake of using the built-in functions (iMA, etc.) to directly produce the values, when what they actually return is a handle to retrieve the values. Tell ChatGPT this and it'll fix it:
iMA returns handles. You'll need to use those handles to copy the buffers every new candle. - It will then try to use something like emaFast[0], but note that that is being calculated on the currently forming candle. You need to use emaFast[1] to get the calculation on the last close candle. Again, tell this to ChatGPT and it'll fix it.
- ChatGPT does an absolutely terrible job if you copy/paste all the errors to it at once — it doesn't know how to sort them all out. Go line by line, and it does a pretty good job. A lot of the errors will be that it used MT4 syntax instead of MT5. This can be very frustrating to keep having the same errors over and over.
ChatGPT does make a lot of mistakes and does write some pretty bad code. But as you learn to work with it, you can get functional code. It's also pretty decent at making small modifications to existing working code. Even though I've gotten to where I can do things myself, I still use ChatGPT a fair amount because it's simply faster than I am... but not when you have to spend an hour getting it to correct itself.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use