-
Why did you post your MT4 question in the MT5 General section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. -
Don't try to do that. There are no buffers, no IndicatorCounted() or prev_calculated. No way to know if older bars have changed or been added (history update.)
Just get the value(s) of the indicator(s) into EA/indicator (using iCustom) and do what you want with it.
(MT4) Detailed explanation of iCustom - MQL4 programming forum (2017) -
You have several reasons, but do not state them.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problem
The XY Problem -
Just embed the other indicator(s) inside your indicator/EA. Add the CI(s) to your code as a resource.
Use the publicly released code - MQL5 programming forum (2017)
Resources - MQL4 ReferenceBe aware that using resources is 40x times slower than using CIs directly.
A custom indicator as a resource - MQL4 programming forum (2019)Also make use there are no spaces in the path.
Getting error 4802 when loading custom indicator that loads another custom indicator with iCustom - Technical Indicators - MQL5 programming forum. (2020)
- You can embed an indicator in an EA's final executable code, while still using iCustom, by making use of resources.
- Also, stop using the old MQL4 event handlers (init, start, etc.) and use the updated MQL4+ ones (OnInit, OnCalculate, etc.), and do not mix them.
- Always make use of "#property strict" for better code, and always fix all compiler warnings too. Don't ignore them.
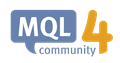
-
Why did you post your MT4 question in the MT5 General section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. -
Don't try to do that. There are no buffers, no IndicatorCounted() or prev_calculated. No way to know if older bars have changed or been added (history update.)
Just get the value(s) of the indicator(s) into EA/indicator (using iCustom) and do what you want with it.
(MT4) Detailed explanation of iCustom - MQL4 programming forum (2017) -
You have several reasons, but do not state them.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problem
The XY Problem -
Just embed the other indicator(s) inside your indicator/EA. Add the CI(s) to your code as a resource.
Use the publicly released code - MQL5 programming forum (2017)
Resources - MQL4 ReferenceBe aware that using resources is 40x times slower than using CIs directly.
A custom indicator as a resource - MQL4 programming forum (2019)Also make use there are no spaces in the path.
Getting error 4802 when loading custom indicator that loads another custom indicator with iCustom - Technical Indicators - MQL5 programming forum. (2020)
I apologize for posting in the wrong section.
So if I use the iCustom function and I insert the custom indicator as a resource will it be part of the executable file?
What is the right syntax to insert a custom indicator as a resource?
I apologize for posting in the wrong section.
So if I use the iCustom function and I insert the custom indicator as a resource will it be part of the executable file?
What is the right syntax to insert a custom indicator as a resource?
- OK
- Did you bother to read the links provided about resources?
- See those blue, underlined, words? They are links. You click on them to see more information.
- OK
- Did you bother to read the links provided about resources?
- See those blue, underlined, words? They are links. You click on them to see more information.
Thanks for your reply William, sorry for trivial questions.
Thank you too Fernando Carreiro.
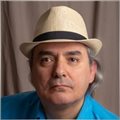

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would also be interested in paid private lessons from very experienced MQL4 developers if any of you offer this kind of service.
Thanks to everyone who can help me
I also did the indicator conversion using OnCalculate(), do you think this is correct?